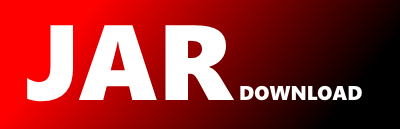
g1901_2000.s1912_design_movie_rental_system.MovieRentingSystem.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-kotlin Show documentation
Show all versions of leetcode-in-kotlin Show documentation
Kotlin-based LeetCode algorithm problem solutions, regularly updated
package g1901_2000.s1912_design_movie_rental_system
// #Hard #Array #Hash_Table #Design #Heap_Priority_Queue #Ordered_Set
// #2023_06_20_Time_3005_ms_(100.00%)_Space_206.6_MB_(100.00%)
import java.util.TreeSet
@Suppress("UNUSED_PARAMETER")
class MovieRentingSystem(n: Int, entries: Array) {
private class Point(var movie: Int, var shop: Int, var price: Int)
private val unrentedMovies = HashMap>()
private val shopMovieToPrice = HashMap()
private val comparator = Comparator { o1: Point, o2: Point ->
return@Comparator if (o1.price != o2.price) {
Integer.compare(o1.price, o2.price)
} else if (o1.shop != o2.shop) {
Integer.compare(o1.shop, o2.shop)
} else {
Integer.compare(o1.movie, o2.movie)
}
}
private val rented = TreeSet(comparator)
init {
for (entry in entries) {
val shop = entry[0]
val movie = entry[1]
val price = entry[2]
unrentedMovies.putIfAbsent(movie, TreeSet(comparator))
unrentedMovies[movie]!!.add(Point(movie, shop, price))
shopMovieToPrice["$shop+$movie"] = price
}
}
fun search(movie: Int): List {
if (!unrentedMovies.containsKey(movie)) {
return ArrayList()
}
val iterator: Iterator = unrentedMovies[movie]!!.iterator()
val listOfShops: MutableList = ArrayList()
while (iterator.hasNext() && listOfShops.size < 5) {
listOfShops.add(iterator.next().shop)
}
return listOfShops
}
fun rent(shop: Int, movie: Int) {
val price = shopMovieToPrice["$shop+$movie"]!!
rented.add(Point(movie, shop, price))
unrentedMovies[movie]!!.remove(Point(movie, shop, price))
}
fun drop(shop: Int, movie: Int) {
val price = shopMovieToPrice["$shop+$movie"]!!
rented.remove(Point(movie, shop, price))
unrentedMovies[movie]!!.add(Point(movie, shop, price))
}
fun report(): List> {
val ans: MutableList> = ArrayList()
val iterator: Iterator = rented.iterator()
while (iterator.hasNext() && ans.size < 5) {
val point = iterator.next()
ans.add(listOf(point.shop, point.movie))
}
return ans
}
}
/*
* Your MovieRentingSystem object will be instantiated and called as such:
* var obj = MovieRentingSystem(n, entries)
* var param_1 = obj.search(movie)
* obj.rent(shop,movie)
* obj.drop(shop,movie)
* var param_4 = obj.report()
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy