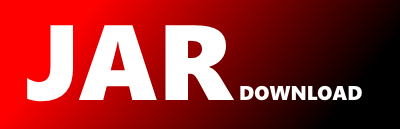
g1301_1400.s1361_validate_binary_tree_nodes.Solution.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-kotlin Show documentation
Show all versions of leetcode-in-kotlin Show documentation
Kotlin-based LeetCode algorithm problem solutions, regularly updated
package g1301_1400.s1361_validate_binary_tree_nodes
// #Medium #Depth_First_Search #Breadth_First_Search #Tree #Binary_Tree #Graph #Union_Find
// #2023_06_06_Time_316_ms_(83.33%)_Space_39.5_MB_(83.33%)
import java.util.ArrayDeque
import java.util.Deque
class Solution {
fun validateBinaryTreeNodes(n: Int, leftChild: IntArray, rightChild: IntArray): Boolean {
val inDeg = IntArray(n)
for (i in 0 until n) {
if (leftChild[i] >= 0) {
inDeg[leftChild[i]] += 1
}
if (rightChild[i] >= 0) {
inDeg[rightChild[i]] += 1
}
}
val queue: Deque = ArrayDeque()
for (i in 0 until n) {
if (inDeg[i] == 0) {
if (queue.isEmpty()) {
queue.offer(i)
} else {
// Violate rule 1.
return false
}
}
if (inDeg[i] > 1) {
// Violate rule 2.
return false
}
}
var tpLen = 0
while (queue.isNotEmpty()) {
val curNode = queue.poll()
tpLen++
val left = leftChild[curNode]
val right = rightChild[curNode]
if (left > -1 && --inDeg[left] == 0) {
queue.offer(left)
}
if (right > -1 && --inDeg[right] == 0) {
queue.offer(right)
}
}
return tpLen == n
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy