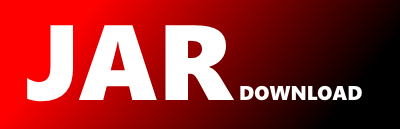
com.github.underscore.Optional Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of underscore8 Show documentation
Show all versions of underscore8 Show documentation
The java 8 port of Underscore.js
The newest version!
package com.github.underscore;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
public final class Optional {
private static final Optional> EMPTY = new Optional();
private final T arg;
private final boolean absent;
private Optional() {
this.arg = null;
this.absent = true;
}
private Optional(final T arg) {
this.arg = arg;
this.absent = false;
}
public static Optional of(final T arg) {
return new Optional(arg);
}
public static Optional fromNullable(final T nullableReference) {
return nullableReference == null ? Optional.absent()
: new Optional(nullableReference);
}
@SuppressWarnings("unchecked")
public static Optional absent() {
return (Optional) EMPTY;
}
public T get() {
if (absent) {
throw new IllegalStateException("Optional.get() cannot be called on an absent value");
}
return arg;
}
public T or(final T defaultValue) {
if (absent) {
return defaultValue;
}
return arg;
}
public T orNull() {
if (absent) {
return null;
}
return arg;
}
public boolean isEmpty() {
return absent;
}
public boolean isPresent() {
return !absent;
}
@SuppressWarnings("unchecked")
public Optional filter(Predicate super T> predicate) {
U.checkNotNull(predicate);
if (isPresent()) {
return predicate.test(arg) ? this : Optional.absent();
} else {
return this;
}
}
public Optional map(Function super T, F> mapper) {
U.checkNotNull(mapper);
if (isPresent()) {
return Optional.fromNullable(mapper.apply(arg));
} else {
return absent();
}
}
public T orThrow(Supplier extends X> exceptionFunction) throws X {
if (absent) {
throw exceptionFunction.get();
} else {
return arg;
}
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
final Optional optional = (Optional) o;
return absent == optional.absent && !(arg == null ? optional.arg != null : !arg.equals(optional.arg));
}
@Override
public int hashCode() {
int result = arg == null ? 0 : arg.hashCode();
result = 31 * result + (absent ? 1 : 0);
return result;
}
@Override
public String toString() {
return absent ? "Optional.absent()" : "Optional.of(" + arg + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy