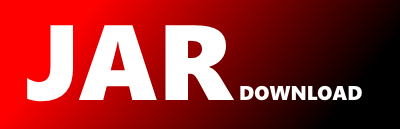
com.github.javahao.util.MapSort Maven / Gradle / Ivy
The newest version!
package com.github.javahao.util;
import java.util.*;
public class MapSort {
/**
* * 使用 Map按key进行排序
* @param map 参数
* @param 键
* @param 值
* @return 返回排序结果
*/
public static Map sortMapByKey(Map map) {
if (map == null || map.isEmpty()) {
return null;
}
Map sortMap = new TreeMap(new Comparator() {
public int compare(K o1, K o2) {
return String.valueOf(o1).compareTo(String.valueOf(o2));
}
});
sortMap.putAll(map);
return sortMap;
}
/**
* 使用 Map按value进行排序
* @param map 参数
* @param 键
* @param 值
* @return 结果
*/
public static Map sortMapByValue(Map map) {
if (map == null || map.isEmpty()) {
return null;
}
Map sortedMap = new LinkedHashMap();
List> entryList = new ArrayList>(map.entrySet());
Collections.sort(entryList, new Comparator>() {
public int compare(Map.Entry o1, Map.Entry o2) {
return String.valueOf(o1.getValue()).compareTo(String.valueOf(o2.getValue()));
}
});
Iterator> iter = entryList.iterator();
Map.Entry tmpEntry = null;
while (iter.hasNext()) {
tmpEntry = iter.next();
sortedMap.put(tmpEntry.getKey(), tmpEntry.getValue());
}
return sortedMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy