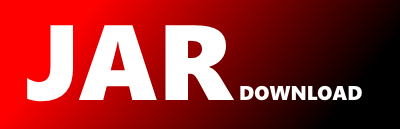
examples.SampleRGui Maven / Gradle / Ivy
package examples;
import com.github.rcaller.rstuff.RCaller;
import com.github.rcaller.rstuff.RCode;
import java.awt.event.KeyEvent;
import java.io.ByteArrayOutputStream;
public class SampleRGui extends javax.swing.JFrame {
RCaller caller;
RCode code;
ByteArrayOutputStream byteOutput;
/**
* Creates new form SampleRGui
*/
public SampleRGui() {
initComponents();
caller = RCaller.create();
code = RCode.create();
caller.setRCode(code);
byteOutput = new ByteArrayOutputStream();
caller.redirectROutputToStream(byteOutput);
code.addRCode("a<-1");
code.addRCode("version");
caller.runAndReturnResultOnline("a");
String result = byteOutput.toString();
jTextArea1.append(result);
byteOutput.reset();
this.setVisible(true);
jTextField1.requestFocus();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
jScrollPane1 = new javax.swing.JScrollPane();
jTextArea1 = new javax.swing.JTextArea();
jTextField1 = new javax.swing.JTextField();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("R GUI - RCaller");
jTextArea1.setColumns(20);
jTextArea1.setFont(new java.awt.Font("Monospaced", 0, 14)); // NOI18N
jTextArea1.setRows(5);
jScrollPane1.setViewportView(jTextArea1);
jTextField1.setFont(new java.awt.Font("Monospaced", 0, 14)); // NOI18N
jTextField1.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
jTextField1KeyPressed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 677, Short.MAX_VALUE)
.addComponent(jTextField1))
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 372, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jTextField1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
);
pack();
}// //GEN-END:initComponents
private void jTextField1KeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_jTextField1KeyPressed
if(evt.getKeyCode() == KeyEvent.VK_ENTER){
code.clearOnline();
code.addRCode(jTextField1.getText());
caller.runAndReturnResultOnline("a");
jTextField1.setText("");
jTextArea1.append(byteOutput.toString());
byteOutput.reset();
}
}//GEN-LAST:event_jTextField1KeyPressed
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(SampleRGui.class.getName()).log(java.util.logging.Level.SEVERE, null, ex.getMessage());
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(SampleRGui.class.getName()).log(java.util.logging.Level.SEVERE, null, ex.getMessage());
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(SampleRGui.class.getName()).log(java.util.logging.Level.SEVERE, null, ex.getMessage());
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(SampleRGui.class.getName()).log(java.util.logging.Level.SEVERE, null, ex.getMessage());
}
//
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new SampleRGui().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTextArea jTextArea1;
private javax.swing.JTextField jTextField1;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy