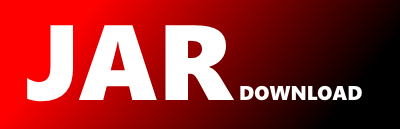
com.github.jcustenborder.kafka.connect.vertica.PoolOfPools Maven / Gradle / Ivy
/**
* Copyright © 2017 Jeremy Custenborder ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.jcustenborder.kafka.connect.vertica;
import com.google.common.collect.ComparisonChain;
import com.google.common.collect.Maps;
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.sql.DataSource;
import java.util.Map;
import java.util.Objects;
class PoolOfPools {
private static final Logger log = LoggerFactory.getLogger(PoolOfPools.class);
private static final Map DATASOURCES = Maps.newConcurrentMap();
static class Key implements Comparable {
final String host;
final int port;
final String username;
final String database;
Key(String host, int port, String username, String database) {
this.host = host;
this.port = port;
this.username = username;
this.database = database;
}
@Override
public int compareTo(Key that) {
return ComparisonChain.start()
.compare(this.host, that.host)
.compare(this.port, that.port)
.compare(this.username, that.username)
.compare(this.database, that.database)
.result();
}
@Override
public int hashCode() {
return Objects.hash(this.host, this.port, this.username, this.database);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Key) {
return 0 == compareTo((Key) obj);
} else {
return false;
}
}
}
static Key of(VerticaSinkConnectorConfig config) {
return new Key(config.host, config.port, config.username, config.database);
}
public static DataSource get(final VerticaSinkConnectorConfig config) {
Key key = of(config);
return DATASOURCES.computeIfAbsent(key, k -> {
String jdbcUrl = String.format(
"jdbc:vertica://%s:%s/%s",
config.host,
config.port,
config.database);
log.info("Creating connection pool for {}", jdbcUrl);
HikariConfig hikariConfig = new HikariConfig();
hikariConfig.setJdbcUrl(jdbcUrl);
hikariConfig.setUsername(config.username);
hikariConfig.setPassword(config.password);
hikariConfig.setDataSourceClassName(com.vertica.jdbc.DataSource.class.getName());
hikariConfig.setAutoCommit(false);
hikariConfig.addDataSourceProperty("database", config.database);
hikariConfig.addDataSourceProperty("host", config.host);
return new HikariDataSource(hikariConfig);
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy