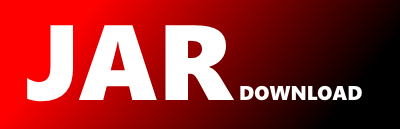
books.BookForm Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.03.31 at 04:48:39 AM UTC
//
package books;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
import com.github.jcustenborder.kafka.connect.xml.Connectable;
import com.github.jcustenborder.kafka.connect.xml.ConnectableHelper;
import org.apache.kafka.connect.data.Date;
import org.apache.kafka.connect.data.Decimal;
import org.apache.kafka.connect.data.Schema;
import org.apache.kafka.connect.data.SchemaBuilder;
import org.apache.kafka.connect.data.Struct;
/**
* Java class for BookForm complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="BookForm">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="test_boolean" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="author" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="title" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="genre" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="price" type="{http://www.w3.org/2001/XMLSchema}float"/>
* <element name="pub_date" type="{http://www.w3.org/2001/XMLSchema}date"/>
* <element name="review" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="note" type="{http://www.w3.org/2001/XMLSchema}int" maxOccurs="unbounded" minOccurs="0"/>
* <element name="score" type="{urn:books}score" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="id" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "BookForm", propOrder = {
"testBoolean",
"author",
"title",
"genre",
"price",
"pubDate",
"review",
"note",
"score"
})
public class BookForm implements Connectable
{
@XmlElement(name = "test_boolean")
protected boolean testBoolean;
@XmlElement(required = true)
protected String author;
@XmlElement(required = true)
protected String title;
@XmlElement(required = true)
protected String genre;
protected float price;
@XmlElement(name = "pub_date", required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar pubDate;
protected List review;
@XmlElement(type = Integer.class)
protected List note;
protected List score;
@XmlAttribute(name = "id")
protected String id;
public final static Schema CONNECT_SCHEMA;
static {
SchemaBuilder builder = SchemaBuilder.struct();
builder.name("books.BookForm");
builder.optional();
SchemaBuilder fieldBuilder;
fieldBuilder = SchemaBuilder.bool();
fieldBuilder.optional();
builder.field("test_boolean", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("author", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("title", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("genre", fieldBuilder.build());
fieldBuilder = SchemaBuilder.float32();
fieldBuilder.optional();
builder.field("price", fieldBuilder.build());
fieldBuilder = Date.builder();
builder.field("pub_date", fieldBuilder.build());
fieldBuilder = SchemaBuilder.array(Schema.STRING_SCHEMA);
fieldBuilder.optional();
builder.field("review", fieldBuilder.build());
fieldBuilder = SchemaBuilder.array(Schema.INT32_SCHEMA);
fieldBuilder.optional();
builder.field("note", fieldBuilder.build());
fieldBuilder = SchemaBuilder.array(Decimal.builder(12).build());
fieldBuilder.optional();
builder.field("score", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
fieldBuilder.optional();
builder.field("id", fieldBuilder.build());
CONNECT_SCHEMA = builder.build();
}
/**
* Gets the value of the testBoolean property.
*
*/
public boolean isTestBoolean() {
return testBoolean;
}
/**
* Sets the value of the testBoolean property.
*
*/
public void setTestBoolean(boolean value) {
this.testBoolean = value;
}
/**
* Gets the value of the author property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAuthor() {
return author;
}
/**
* Sets the value of the author property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAuthor(String value) {
this.author = value;
}
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTitle(String value) {
this.title = value;
}
/**
* Gets the value of the genre property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGenre() {
return genre;
}
/**
* Sets the value of the genre property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGenre(String value) {
this.genre = value;
}
/**
* Gets the value of the price property.
*
*/
public float getPrice() {
return price;
}
/**
* Sets the value of the price property.
*
*/
public void setPrice(float value) {
this.price = value;
}
/**
* Gets the value of the pubDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getPubDate() {
return pubDate;
}
/**
* Sets the value of the pubDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setPubDate(XMLGregorianCalendar value) {
this.pubDate = value;
}
/**
* Gets the value of the review property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the review property.
*
*
* For example, to add a new item, do as follows:
*
* getReview().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getReview() {
if (review == null) {
review = new ArrayList();
}
return this.review;
}
/**
* Gets the value of the note property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the note property.
*
*
* For example, to add a new item, do as follows:
*
* getNote().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Integer }
*
*
*/
public List getNote() {
if (note == null) {
note = new ArrayList();
}
return this.note;
}
/**
* Gets the value of the score property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the score property.
*
*
* For example, to add a new item, do as follows:
*
* getScore().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BigDecimal }
*
*
*/
public List getScore() {
if (score == null) {
score = new ArrayList();
}
return this.score;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final BookForm that = ((BookForm) object);
{
boolean leftTestBoolean;
leftTestBoolean = this.isTestBoolean();
boolean rightTestBoolean;
rightTestBoolean = that.isTestBoolean();
if (leftTestBoolean!= rightTestBoolean) {
return false;
}
}
{
String leftAuthor;
leftAuthor = this.getAuthor();
String rightAuthor;
rightAuthor = that.getAuthor();
if (this.author!= null) {
if (that.author!= null) {
if (!leftAuthor.equals(rightAuthor)) {
return false;
}
} else {
return false;
}
} else {
if (that.author!= null) {
return false;
}
}
}
{
String leftTitle;
leftTitle = this.getTitle();
String rightTitle;
rightTitle = that.getTitle();
if (this.title!= null) {
if (that.title!= null) {
if (!leftTitle.equals(rightTitle)) {
return false;
}
} else {
return false;
}
} else {
if (that.title!= null) {
return false;
}
}
}
{
String leftGenre;
leftGenre = this.getGenre();
String rightGenre;
rightGenre = that.getGenre();
if (this.genre!= null) {
if (that.genre!= null) {
if (!leftGenre.equals(rightGenre)) {
return false;
}
} else {
return false;
}
} else {
if (that.genre!= null) {
return false;
}
}
}
{
float leftPrice;
leftPrice = this.getPrice();
float rightPrice;
rightPrice = that.getPrice();
if (leftPrice!= rightPrice) {
return false;
}
}
{
XMLGregorianCalendar leftPubDate;
leftPubDate = this.getPubDate();
XMLGregorianCalendar rightPubDate;
rightPubDate = that.getPubDate();
if (this.pubDate!= null) {
if (that.pubDate!= null) {
if (!leftPubDate.equals(rightPubDate)) {
return false;
}
} else {
return false;
}
} else {
if (that.pubDate!= null) {
return false;
}
}
}
{
List leftReview;
leftReview = (((this.review!= null)&&(!this.review.isEmpty()))?this.getReview():null);
List rightReview;
rightReview = (((that.review!= null)&&(!that.review.isEmpty()))?that.getReview():null);
if ((this.review!= null)&&(!this.review.isEmpty())) {
if ((that.review!= null)&&(!that.review.isEmpty())) {
if (!leftReview.equals(rightReview)) {
return false;
}
} else {
return false;
}
} else {
if ((that.review!= null)&&(!that.review.isEmpty())) {
return false;
}
}
}
{
List leftNote;
leftNote = (((this.note!= null)&&(!this.note.isEmpty()))?this.getNote():null);
List rightNote;
rightNote = (((that.note!= null)&&(!that.note.isEmpty()))?that.getNote():null);
if ((this.note!= null)&&(!this.note.isEmpty())) {
if ((that.note!= null)&&(!that.note.isEmpty())) {
if (!leftNote.equals(rightNote)) {
return false;
}
} else {
return false;
}
} else {
if ((that.note!= null)&&(!that.note.isEmpty())) {
return false;
}
}
}
{
List leftScore;
leftScore = (((this.score!= null)&&(!this.score.isEmpty()))?this.getScore():null);
List rightScore;
rightScore = (((that.score!= null)&&(!that.score.isEmpty()))?that.getScore():null);
if ((this.score!= null)&&(!this.score.isEmpty())) {
if ((that.score!= null)&&(!that.score.isEmpty())) {
if (!leftScore.equals(rightScore)) {
return false;
}
} else {
return false;
}
} else {
if ((that.score!= null)&&(!that.score.isEmpty())) {
return false;
}
}
}
{
String leftId;
leftId = this.getId();
String rightId;
rightId = that.getId();
if (this.id!= null) {
if (that.id!= null) {
if (!leftId.equals(rightId)) {
return false;
}
} else {
return false;
}
} else {
if (that.id!= null) {
return false;
}
}
}
return true;
}
@Override
public Struct toStruct() {
Struct struct = new Struct(CONNECT_SCHEMA);
ConnectableHelper.toBoolean(struct, "test_boolean", this.testBoolean);
ConnectableHelper.toString(struct, "author", this.author);
ConnectableHelper.toString(struct, "title", this.title);
ConnectableHelper.toString(struct, "genre", this.genre);
ConnectableHelper.toFloat32(struct, "price", this.price);
ConnectableHelper.toDate(struct, "pub_date", this.pubDate);
ConnectableHelper.toArray(struct, "review", this.review);
ConnectableHelper.toArray(struct, "note", this.note);
ConnectableHelper.toArray(struct, "score", this.score);
ConnectableHelper.toString(struct, "id", this.id);
return struct;
}
@Override
public void fromStruct(Struct struct) {
this.testBoolean = ConnectableHelper.fromBoolean(struct, "test_boolean");
this.author = ConnectableHelper.fromString(struct, "author");
this.title = ConnectableHelper.fromString(struct, "title");
this.genre = ConnectableHelper.fromString(struct, "genre");
this.price = ConnectableHelper.fromFloat32(struct, "price");
this.pubDate = ConnectableHelper.fromDate(struct, "pub_date");
this.review = ConnectableHelper.fromArray(struct, "review", String.class);
this.note = ConnectableHelper.fromArray(struct, "note", Integer.class);
this.score = ConnectableHelper.fromArray(struct, "score", BigDecimal.class);
this.id = ConnectableHelper.fromString(struct, "id");
}
}