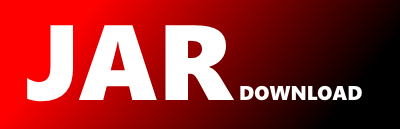
enumerations.BookForm Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.03.31 at 04:48:39 AM UTC
//
package enumerations;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import com.github.jcustenborder.kafka.connect.xml.Connectable;
import com.github.jcustenborder.kafka.connect.xml.ConnectableHelper;
import org.apache.kafka.connect.data.Schema;
import org.apache.kafka.connect.data.SchemaBuilder;
import org.apache.kafka.connect.data.Struct;
/**
* Java class for BookForm complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="BookForm">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="review" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="bookType" type="{urn:enumerations}BookType"/>
* </sequence>
* <attribute name="id" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "BookForm", propOrder = {
"review",
"bookType"
})
public class BookForm implements Connectable
{
@XmlElement(required = true)
protected String review;
@XmlElement(required = true)
@XmlSchemaType(name = "NCName")
protected BookType bookType;
@XmlAttribute(name = "id")
protected String id;
public final static Schema CONNECT_SCHEMA;
static {
SchemaBuilder builder = SchemaBuilder.struct();
builder.name("enumerations.BookForm");
builder.optional();
SchemaBuilder fieldBuilder;
fieldBuilder = SchemaBuilder.string();
builder.field("review", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("bookType", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
fieldBuilder.optional();
builder.field("id", fieldBuilder.build());
CONNECT_SCHEMA = builder.build();
}
/**
* Gets the value of the review property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReview() {
return review;
}
/**
* Sets the value of the review property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReview(String value) {
this.review = value;
}
/**
* Gets the value of the bookType property.
*
* @return
* possible object is
* {@link BookType }
*
*/
public BookType getBookType() {
return bookType;
}
/**
* Sets the value of the bookType property.
*
* @param value
* allowed object is
* {@link BookType }
*
*/
public void setBookType(BookType value) {
this.bookType = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final BookForm that = ((BookForm) object);
{
String leftReview;
leftReview = this.getReview();
String rightReview;
rightReview = that.getReview();
if (this.review!= null) {
if (that.review!= null) {
if (!leftReview.equals(rightReview)) {
return false;
}
} else {
return false;
}
} else {
if (that.review!= null) {
return false;
}
}
}
{
BookType leftBookType;
leftBookType = this.getBookType();
BookType rightBookType;
rightBookType = that.getBookType();
if (this.bookType!= null) {
if (that.bookType!= null) {
if (!leftBookType.equals(rightBookType)) {
return false;
}
} else {
return false;
}
} else {
if (that.bookType!= null) {
return false;
}
}
}
{
String leftId;
leftId = this.getId();
String rightId;
rightId = that.getId();
if (this.id!= null) {
if (that.id!= null) {
if (!leftId.equals(rightId)) {
return false;
}
} else {
return false;
}
} else {
if (that.id!= null) {
return false;
}
}
}
return true;
}
@Override
public Struct toStruct() {
Struct struct = new Struct(CONNECT_SCHEMA);
ConnectableHelper.toString(struct, "review", this.review);
ConnectableHelper.fromEnum(struct, "bookType", this.bookType);
ConnectableHelper.toString(struct, "id", this.id);
return struct;
}
@Override
public void fromStruct(Struct struct) {
this.review = ConnectableHelper.fromString(struct, "review");
this.bookType = ConnectableHelper.toEnum(struct, "bookType", BookType.class);
this.id = ConnectableHelper.fromString(struct, "id");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy