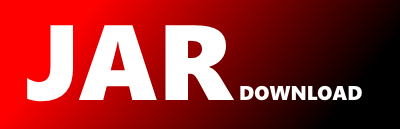
generated.Shiporder Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.03.31 at 04:48:39 AM UTC
//
package generated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import com.github.jcustenborder.kafka.connect.xml.Connectable;
import com.github.jcustenborder.kafka.connect.xml.ConnectableHelper;
import org.apache.kafka.connect.data.Decimal;
import org.apache.kafka.connect.data.Schema;
import org.apache.kafka.connect.data.SchemaBuilder;
import org.apache.kafka.connect.data.Struct;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="orderperson" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="shipto">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="address" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="city" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="country" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="item" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="title" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="note" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="quantity" type="{http://www.w3.org/2001/XMLSchema}positiveInteger"/>
* <element name="price" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* <attribute name="orderid" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"orderperson",
"shipto",
"item"
})
@XmlRootElement(name = "shiporder")
public class Shiporder
implements Connectable
{
@XmlElement(required = true)
protected String orderperson;
@XmlElement(required = true)
protected Shiporder.Shipto shipto;
@XmlElement(required = true)
protected List item;
@XmlAttribute(name = "orderid", required = true)
protected String orderid;
public final static Schema CONNECT_SCHEMA;
static {
SchemaBuilder builder = SchemaBuilder.struct();
builder.name("generated.Shiporder");
builder.optional();
SchemaBuilder fieldBuilder;
fieldBuilder = SchemaBuilder.string();
builder.field("orderperson", fieldBuilder.build());
builder.field("shipto", Shiporder.Shipto.CONNECT_SCHEMA);
fieldBuilder = SchemaBuilder.array(Shiporder.Item.CONNECT_SCHEMA);
builder.field("item", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("orderid", fieldBuilder.build());
CONNECT_SCHEMA = builder.build();
}
/**
* Gets the value of the orderperson property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrderperson() {
return orderperson;
}
/**
* Sets the value of the orderperson property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrderperson(String value) {
this.orderperson = value;
}
/**
* Gets the value of the shipto property.
*
* @return
* possible object is
* {@link Shiporder.Shipto }
*
*/
public Shiporder.Shipto getShipto() {
return shipto;
}
/**
* Sets the value of the shipto property.
*
* @param value
* allowed object is
* {@link Shiporder.Shipto }
*
*/
public void setShipto(Shiporder.Shipto value) {
this.shipto = value;
}
/**
* Gets the value of the item property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the item property.
*
*
* For example, to add a new item, do as follows:
*
* getItem().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Shiporder.Item }
*
*
*/
public List getItem() {
if (item == null) {
item = new ArrayList();
}
return this.item;
}
/**
* Gets the value of the orderid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrderid() {
return orderid;
}
/**
* Sets the value of the orderid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrderid(String value) {
this.orderid = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Shiporder that = ((Shiporder) object);
{
String leftOrderperson;
leftOrderperson = this.getOrderperson();
String rightOrderperson;
rightOrderperson = that.getOrderperson();
if (this.orderperson!= null) {
if (that.orderperson!= null) {
if (!leftOrderperson.equals(rightOrderperson)) {
return false;
}
} else {
return false;
}
} else {
if (that.orderperson!= null) {
return false;
}
}
}
{
Shiporder.Shipto leftShipto;
leftShipto = this.getShipto();
Shiporder.Shipto rightShipto;
rightShipto = that.getShipto();
if (this.shipto!= null) {
if (that.shipto!= null) {
if (!leftShipto.equals(rightShipto)) {
return false;
}
} else {
return false;
}
} else {
if (that.shipto!= null) {
return false;
}
}
}
{
List leftItem;
leftItem = (((this.item!= null)&&(!this.item.isEmpty()))?this.getItem():null);
List rightItem;
rightItem = (((that.item!= null)&&(!that.item.isEmpty()))?that.getItem():null);
if ((this.item!= null)&&(!this.item.isEmpty())) {
if ((that.item!= null)&&(!that.item.isEmpty())) {
if (!leftItem.equals(rightItem)) {
return false;
}
} else {
return false;
}
} else {
if ((that.item!= null)&&(!that.item.isEmpty())) {
return false;
}
}
}
{
String leftOrderid;
leftOrderid = this.getOrderid();
String rightOrderid;
rightOrderid = that.getOrderid();
if (this.orderid!= null) {
if (that.orderid!= null) {
if (!leftOrderid.equals(rightOrderid)) {
return false;
}
} else {
return false;
}
} else {
if (that.orderid!= null) {
return false;
}
}
}
return true;
}
@Override
public Struct toStruct() {
Struct struct = new Struct(CONNECT_SCHEMA);
ConnectableHelper.toString(struct, "orderperson", this.orderperson);
ConnectableHelper.toStruct(struct, "shipto", this.shipto);
ConnectableHelper.toArray(struct, "item", this.item);
ConnectableHelper.toString(struct, "orderid", this.orderid);
return struct;
}
@Override
public void fromStruct(Struct struct) {
this.orderperson = ConnectableHelper.fromString(struct, "orderperson");
this.shipto = ConnectableHelper.fromStruct(struct, "shipto", Shiporder.Shipto.class);
this.item = ConnectableHelper.fromArray(struct, "item", Shiporder.Item.class);
this.orderid = ConnectableHelper.fromString(struct, "orderid");
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="title" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="note" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="quantity" type="{http://www.w3.org/2001/XMLSchema}positiveInteger"/>
* <element name="price" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"title",
"note",
"quantity",
"price"
})
public static class Item implements Connectable
{
@XmlElement(required = true)
protected String title;
protected String note;
@XmlElement(required = true)
@XmlSchemaType(name = "positiveInteger")
protected BigInteger quantity;
@XmlElement(required = true)
protected BigDecimal price;
public final static Schema CONNECT_SCHEMA;
static {
SchemaBuilder builder = SchemaBuilder.struct();
builder.name("generated.Item");
builder.optional();
SchemaBuilder fieldBuilder;
fieldBuilder = SchemaBuilder.string();
builder.field("title", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
fieldBuilder.optional();
builder.field("note", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
builder.field("quantity", fieldBuilder.build());
fieldBuilder = Decimal.builder(12);
builder.field("price", fieldBuilder.build());
CONNECT_SCHEMA = builder.build();
}
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTitle(String value) {
this.title = value;
}
/**
* Gets the value of the note property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNote() {
return note;
}
/**
* Sets the value of the note property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNote(String value) {
this.note = value;
}
/**
* Gets the value of the quantity property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getQuantity() {
return quantity;
}
/**
* Sets the value of the quantity property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setQuantity(BigInteger value) {
this.quantity = value;
}
/**
* Gets the value of the price property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getPrice() {
return price;
}
/**
* Sets the value of the price property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setPrice(BigDecimal value) {
this.price = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Shiporder.Item that = ((Shiporder.Item) object);
{
String leftTitle;
leftTitle = this.getTitle();
String rightTitle;
rightTitle = that.getTitle();
if (this.title!= null) {
if (that.title!= null) {
if (!leftTitle.equals(rightTitle)) {
return false;
}
} else {
return false;
}
} else {
if (that.title!= null) {
return false;
}
}
}
{
String leftNote;
leftNote = this.getNote();
String rightNote;
rightNote = that.getNote();
if (this.note!= null) {
if (that.note!= null) {
if (!leftNote.equals(rightNote)) {
return false;
}
} else {
return false;
}
} else {
if (that.note!= null) {
return false;
}
}
}
{
BigInteger leftQuantity;
leftQuantity = this.getQuantity();
BigInteger rightQuantity;
rightQuantity = that.getQuantity();
if (this.quantity!= null) {
if (that.quantity!= null) {
if (!leftQuantity.equals(rightQuantity)) {
return false;
}
} else {
return false;
}
} else {
if (that.quantity!= null) {
return false;
}
}
}
{
BigDecimal leftPrice;
leftPrice = this.getPrice();
BigDecimal rightPrice;
rightPrice = that.getPrice();
if (this.price!= null) {
if (that.price!= null) {
if (!leftPrice.equals(rightPrice)) {
return false;
}
} else {
return false;
}
} else {
if (that.price!= null) {
return false;
}
}
}
return true;
}
@Override
public Struct toStruct() {
Struct struct = new Struct(CONNECT_SCHEMA);
ConnectableHelper.toString(struct, "title", this.title);
ConnectableHelper.toString(struct, "note", this.note);
ConnectableHelper.toInt64(struct, "quantity", this.quantity);
ConnectableHelper.toDecimal(struct, "price", this.price);
return struct;
}
@Override
public void fromStruct(Struct struct) {
this.title = ConnectableHelper.fromString(struct, "title");
this.note = ConnectableHelper.fromString(struct, "note");
this.quantity = ConnectableHelper.fromInt64BigInteger(struct, "quantity");
this.price = ConnectableHelper.fromDecimal(struct, "price");
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="address" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="city" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="country" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"name",
"address",
"city",
"country"
})
public static class Shipto implements Connectable
{
@XmlElement(required = true)
protected String name;
@XmlElement(required = true)
protected String address;
@XmlElement(required = true)
protected String city;
@XmlElement(required = true)
protected String country;
public final static Schema CONNECT_SCHEMA;
static {
SchemaBuilder builder = SchemaBuilder.struct();
builder.name("generated.Shipto");
builder.optional();
SchemaBuilder fieldBuilder;
fieldBuilder = SchemaBuilder.string();
builder.field("name", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("address", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("city", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("country", fieldBuilder.build());
CONNECT_SCHEMA = builder.build();
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the address property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAddress() {
return address;
}
/**
* Sets the value of the address property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAddress(String value) {
this.address = value;
}
/**
* Gets the value of the city property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCity() {
return city;
}
/**
* Sets the value of the city property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCity(String value) {
this.city = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCountry() {
return country;
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCountry(String value) {
this.country = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Shiporder.Shipto that = ((Shiporder.Shipto) object);
{
String leftName;
leftName = this.getName();
String rightName;
rightName = that.getName();
if (this.name!= null) {
if (that.name!= null) {
if (!leftName.equals(rightName)) {
return false;
}
} else {
return false;
}
} else {
if (that.name!= null) {
return false;
}
}
}
{
String leftAddress;
leftAddress = this.getAddress();
String rightAddress;
rightAddress = that.getAddress();
if (this.address!= null) {
if (that.address!= null) {
if (!leftAddress.equals(rightAddress)) {
return false;
}
} else {
return false;
}
} else {
if (that.address!= null) {
return false;
}
}
}
{
String leftCity;
leftCity = this.getCity();
String rightCity;
rightCity = that.getCity();
if (this.city!= null) {
if (that.city!= null) {
if (!leftCity.equals(rightCity)) {
return false;
}
} else {
return false;
}
} else {
if (that.city!= null) {
return false;
}
}
}
{
String leftCountry;
leftCountry = this.getCountry();
String rightCountry;
rightCountry = that.getCountry();
if (this.country!= null) {
if (that.country!= null) {
if (!leftCountry.equals(rightCountry)) {
return false;
}
} else {
return false;
}
} else {
if (that.country!= null) {
return false;
}
}
}
return true;
}
@Override
public Struct toStruct() {
Struct struct = new Struct(CONNECT_SCHEMA);
ConnectableHelper.toString(struct, "name", this.name);
ConnectableHelper.toString(struct, "address", this.address);
ConnectableHelper.toString(struct, "city", this.city);
ConnectableHelper.toString(struct, "country", this.country);
return struct;
}
@Override
public void fromStruct(Struct struct) {
this.name = ConnectableHelper.fromString(struct, "name");
this.address = ConnectableHelper.fromString(struct, "address");
this.city = ConnectableHelper.fromString(struct, "city");
this.country = ConnectableHelper.fromString(struct, "country");
}
}
}