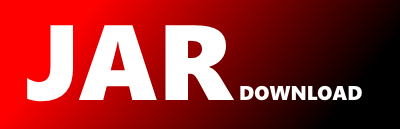
simpletypes.SimpleTypes Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.03.31 at 04:48:39 AM UTC
//
package simpletypes;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Arrays;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.HexBinaryAdapter;
import javax.xml.bind.annotation.adapters.NormalizedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.datatype.XMLGregorianCalendar;
import com.github.jcustenborder.kafka.connect.xml.Connectable;
import com.github.jcustenborder.kafka.connect.xml.ConnectableHelper;
import org.apache.kafka.connect.data.Date;
import org.apache.kafka.connect.data.Decimal;
import org.apache.kafka.connect.data.Schema;
import org.apache.kafka.connect.data.SchemaBuilder;
import org.apache.kafka.connect.data.Struct;
import org.apache.kafka.connect.data.Time;
import org.apache.kafka.connect.data.Timestamp;
/**
* Java class for simpleTypes complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="simpleTypes">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="test_anyURI" type="{http://www.w3.org/2001/XMLSchema}anyURI"/>
* <element name="test_base64Binary" type="{http://www.w3.org/2001/XMLSchema}base64Binary"/>
* <element name="test_boolean" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="test_byte" type="{http://www.w3.org/2001/XMLSchema}byte"/>
* <element name="test_date" type="{http://www.w3.org/2001/XMLSchema}date"/>
* <element name="test_dateTime" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="test_decimal" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* <element name="test_double" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="test_ENTITY" type="{http://www.w3.org/2001/XMLSchema}ENTITY"/>
* <element name="test_float" type="{http://www.w3.org/2001/XMLSchema}float"/>
* <element name="test_gDay" type="{http://www.w3.org/2001/XMLSchema}gDay"/>
* <element name="test_gMonth" type="{http://www.w3.org/2001/XMLSchema}gMonth"/>
* <element name="test_gYear" type="{http://www.w3.org/2001/XMLSchema}gYear"/>
* <element name="test_hexBinary" type="{http://www.w3.org/2001/XMLSchema}hexBinary"/>
* <element name="test_int" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="test_integer" type="{http://www.w3.org/2001/XMLSchema}integer"/>
* <element name="test_long" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="test_Name" type="{http://www.w3.org/2001/XMLSchema}Name"/>
* <element name="test_NCName" type="{http://www.w3.org/2001/XMLSchema}NCName"/>
* <element name="test_negativeInteger" type="{http://www.w3.org/2001/XMLSchema}negativeInteger"/>
* <element name="test_nonNegativeInteger" type="{http://www.w3.org/2001/XMLSchema}nonNegativeInteger"/>
* <element name="test_nonPositiveInteger" type="{http://www.w3.org/2001/XMLSchema}nonPositiveInteger"/>
* <element name="test_normalizedString" type="{http://www.w3.org/2001/XMLSchema}normalizedString"/>
* <element name="test_positiveInteger" type="{http://www.w3.org/2001/XMLSchema}positiveInteger"/>
* <element name="test_short" type="{http://www.w3.org/2001/XMLSchema}short"/>
* <element name="test_string" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="test_time" type="{http://www.w3.org/2001/XMLSchema}time"/>
* <element name="test_token" type="{http://www.w3.org/2001/XMLSchema}token"/>
* <element name="test_unsignedByte" type="{http://www.w3.org/2001/XMLSchema}unsignedByte"/>
* <element name="test_unsignedInt" type="{http://www.w3.org/2001/XMLSchema}unsignedInt"/>
* <element name="test_unsignedLong" type="{http://www.w3.org/2001/XMLSchema}unsignedLong"/>
* <element name="test_unsignedShort" type="{http://www.w3.org/2001/XMLSchema}unsignedShort"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "simpleTypes", propOrder = {
"testAnyURI",
"testBase64Binary",
"testBoolean",
"testByte",
"testDate",
"testDateTime",
"testDecimal",
"testDouble",
"testENTITY",
"testFloat",
"testGDay",
"testGMonth",
"testGYear",
"testHexBinary",
"testInt",
"testInteger",
"testLong",
"testName",
"testNCName",
"testNegativeInteger",
"testNonNegativeInteger",
"testNonPositiveInteger",
"testNormalizedString",
"testPositiveInteger",
"testShort",
"testString",
"testTime",
"testToken",
"testUnsignedByte",
"testUnsignedInt",
"testUnsignedLong",
"testUnsignedShort"
})
public class SimpleTypes
implements Connectable
{
@XmlElement(name = "test_anyURI", required = true)
@XmlSchemaType(name = "anyURI")
protected String testAnyURI;
@XmlElement(name = "test_base64Binary", required = true)
protected byte[] testBase64Binary;
@XmlElement(name = "test_boolean")
protected boolean testBoolean;
@XmlElement(name = "test_byte")
protected byte testByte;
@XmlElement(name = "test_date", required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar testDate;
@XmlElement(name = "test_dateTime", required = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar testDateTime;
@XmlElement(name = "test_decimal", required = true)
protected BigDecimal testDecimal;
@XmlElement(name = "test_double")
protected double testDouble;
@XmlElement(name = "test_ENTITY", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "ENTITY")
protected String testENTITY;
@XmlElement(name = "test_float")
protected float testFloat;
@XmlElement(name = "test_gDay", required = true)
@XmlSchemaType(name = "gDay")
protected XMLGregorianCalendar testGDay;
@XmlElement(name = "test_gMonth", required = true)
@XmlSchemaType(name = "gMonth")
protected XMLGregorianCalendar testGMonth;
@XmlElement(name = "test_gYear", required = true)
@XmlSchemaType(name = "gYear")
protected XMLGregorianCalendar testGYear;
@XmlElement(name = "test_hexBinary", required = true, type = String.class)
@XmlJavaTypeAdapter(HexBinaryAdapter.class)
@XmlSchemaType(name = "hexBinary")
protected byte[] testHexBinary;
@XmlElement(name = "test_int")
protected int testInt;
@XmlElement(name = "test_integer", required = true)
protected BigInteger testInteger;
@XmlElement(name = "test_long")
protected long testLong;
@XmlElement(name = "test_Name", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "Name")
protected String testName;
@XmlElement(name = "test_NCName", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NCName")
protected String testNCName;
@XmlElement(name = "test_negativeInteger", required = true)
@XmlSchemaType(name = "negativeInteger")
protected BigInteger testNegativeInteger;
@XmlElement(name = "test_nonNegativeInteger", required = true)
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger testNonNegativeInteger;
@XmlElement(name = "test_nonPositiveInteger", required = true)
@XmlSchemaType(name = "nonPositiveInteger")
protected BigInteger testNonPositiveInteger;
@XmlElement(name = "test_normalizedString", required = true)
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String testNormalizedString;
@XmlElement(name = "test_positiveInteger", required = true)
@XmlSchemaType(name = "positiveInteger")
protected BigInteger testPositiveInteger;
@XmlElement(name = "test_short")
protected short testShort;
@XmlElement(name = "test_string", required = true)
protected String testString;
@XmlElement(name = "test_time", required = true)
@XmlSchemaType(name = "time")
protected XMLGregorianCalendar testTime;
@XmlElement(name = "test_token", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
protected String testToken;
@XmlElement(name = "test_unsignedByte")
@XmlSchemaType(name = "unsignedByte")
protected short testUnsignedByte;
@XmlElement(name = "test_unsignedInt")
@XmlSchemaType(name = "unsignedInt")
protected long testUnsignedInt;
@XmlElement(name = "test_unsignedLong", required = true)
@XmlSchemaType(name = "unsignedLong")
protected BigInteger testUnsignedLong;
@XmlElement(name = "test_unsignedShort")
@XmlSchemaType(name = "unsignedShort")
protected int testUnsignedShort;
public final static Schema CONNECT_SCHEMA;
static {
SchemaBuilder builder = SchemaBuilder.struct();
builder.name("simpletypes.SimpleTypes");
builder.optional();
SchemaBuilder fieldBuilder;
fieldBuilder = SchemaBuilder.string();
builder.field("test_anyURI", fieldBuilder.build());
fieldBuilder = SchemaBuilder.bytes();
builder.field("test_base64Binary", fieldBuilder.build());
fieldBuilder = SchemaBuilder.bool();
fieldBuilder.optional();
builder.field("test_boolean", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int8();
fieldBuilder.optional();
builder.field("test_byte", fieldBuilder.build());
fieldBuilder = Date.builder();
builder.field("test_date", fieldBuilder.build());
fieldBuilder = Timestamp.builder();
builder.field("test_dateTime", fieldBuilder.build());
fieldBuilder = Decimal.builder(12);
builder.field("test_decimal", fieldBuilder.build());
fieldBuilder = SchemaBuilder.float64();
fieldBuilder.optional();
builder.field("test_double", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("test_ENTITY", fieldBuilder.build());
fieldBuilder = SchemaBuilder.float32();
fieldBuilder.optional();
builder.field("test_float", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int32();
builder.field("test_gDay", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int32();
builder.field("test_gMonth", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int32();
builder.field("test_gYear", fieldBuilder.build());
fieldBuilder = SchemaBuilder.bytes();
builder.field("test_hexBinary", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int32();
fieldBuilder.optional();
builder.field("test_int", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
builder.field("test_integer", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
fieldBuilder.optional();
builder.field("test_long", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("test_Name", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("test_NCName", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
builder.field("test_negativeInteger", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
builder.field("test_nonNegativeInteger", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
builder.field("test_nonPositiveInteger", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("test_normalizedString", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
builder.field("test_positiveInteger", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int16();
fieldBuilder.optional();
builder.field("test_short", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("test_string", fieldBuilder.build());
fieldBuilder = Time.builder();
builder.field("test_time", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
builder.field("test_token", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int16();
fieldBuilder.optional();
builder.field("test_unsignedByte", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
fieldBuilder.optional();
builder.field("test_unsignedInt", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
builder.field("test_unsignedLong", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int32();
fieldBuilder.optional();
builder.field("test_unsignedShort", fieldBuilder.build());
CONNECT_SCHEMA = builder.build();
}
/**
* Gets the value of the testAnyURI property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTestAnyURI() {
return testAnyURI;
}
/**
* Sets the value of the testAnyURI property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTestAnyURI(String value) {
this.testAnyURI = value;
}
/**
* Gets the value of the testBase64Binary property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getTestBase64Binary() {
return testBase64Binary;
}
/**
* Sets the value of the testBase64Binary property.
*
* @param value
* allowed object is
* byte[]
*/
public void setTestBase64Binary(byte[] value) {
this.testBase64Binary = value;
}
/**
* Gets the value of the testBoolean property.
*
*/
public boolean isTestBoolean() {
return testBoolean;
}
/**
* Sets the value of the testBoolean property.
*
*/
public void setTestBoolean(boolean value) {
this.testBoolean = value;
}
/**
* Gets the value of the testByte property.
*
*/
public byte getTestByte() {
return testByte;
}
/**
* Sets the value of the testByte property.
*
*/
public void setTestByte(byte value) {
this.testByte = value;
}
/**
* Gets the value of the testDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTestDate() {
return testDate;
}
/**
* Sets the value of the testDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTestDate(XMLGregorianCalendar value) {
this.testDate = value;
}
/**
* Gets the value of the testDateTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTestDateTime() {
return testDateTime;
}
/**
* Sets the value of the testDateTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTestDateTime(XMLGregorianCalendar value) {
this.testDateTime = value;
}
/**
* Gets the value of the testDecimal property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTestDecimal() {
return testDecimal;
}
/**
* Sets the value of the testDecimal property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTestDecimal(BigDecimal value) {
this.testDecimal = value;
}
/**
* Gets the value of the testDouble property.
*
*/
public double getTestDouble() {
return testDouble;
}
/**
* Sets the value of the testDouble property.
*
*/
public void setTestDouble(double value) {
this.testDouble = value;
}
/**
* Gets the value of the testENTITY property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTestENTITY() {
return testENTITY;
}
/**
* Sets the value of the testENTITY property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTestENTITY(String value) {
this.testENTITY = value;
}
/**
* Gets the value of the testFloat property.
*
*/
public float getTestFloat() {
return testFloat;
}
/**
* Sets the value of the testFloat property.
*
*/
public void setTestFloat(float value) {
this.testFloat = value;
}
/**
* Gets the value of the testGDay property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTestGDay() {
return testGDay;
}
/**
* Sets the value of the testGDay property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTestGDay(XMLGregorianCalendar value) {
this.testGDay = value;
}
/**
* Gets the value of the testGMonth property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTestGMonth() {
return testGMonth;
}
/**
* Sets the value of the testGMonth property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTestGMonth(XMLGregorianCalendar value) {
this.testGMonth = value;
}
/**
* Gets the value of the testGYear property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTestGYear() {
return testGYear;
}
/**
* Sets the value of the testGYear property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTestGYear(XMLGregorianCalendar value) {
this.testGYear = value;
}
/**
* Gets the value of the testHexBinary property.
*
* @return
* possible object is
* {@link String }
*
*/
public byte[] getTestHexBinary() {
return testHexBinary;
}
/**
* Sets the value of the testHexBinary property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTestHexBinary(byte[] value) {
this.testHexBinary = value;
}
/**
* Gets the value of the testInt property.
*
*/
public int getTestInt() {
return testInt;
}
/**
* Sets the value of the testInt property.
*
*/
public void setTestInt(int value) {
this.testInt = value;
}
/**
* Gets the value of the testInteger property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTestInteger() {
return testInteger;
}
/**
* Sets the value of the testInteger property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTestInteger(BigInteger value) {
this.testInteger = value;
}
/**
* Gets the value of the testLong property.
*
*/
public long getTestLong() {
return testLong;
}
/**
* Sets the value of the testLong property.
*
*/
public void setTestLong(long value) {
this.testLong = value;
}
/**
* Gets the value of the testName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTestName() {
return testName;
}
/**
* Sets the value of the testName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTestName(String value) {
this.testName = value;
}
/**
* Gets the value of the testNCName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTestNCName() {
return testNCName;
}
/**
* Sets the value of the testNCName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTestNCName(String value) {
this.testNCName = value;
}
/**
* Gets the value of the testNegativeInteger property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTestNegativeInteger() {
return testNegativeInteger;
}
/**
* Sets the value of the testNegativeInteger property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTestNegativeInteger(BigInteger value) {
this.testNegativeInteger = value;
}
/**
* Gets the value of the testNonNegativeInteger property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTestNonNegativeInteger() {
return testNonNegativeInteger;
}
/**
* Sets the value of the testNonNegativeInteger property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTestNonNegativeInteger(BigInteger value) {
this.testNonNegativeInteger = value;
}
/**
* Gets the value of the testNonPositiveInteger property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTestNonPositiveInteger() {
return testNonPositiveInteger;
}
/**
* Sets the value of the testNonPositiveInteger property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTestNonPositiveInteger(BigInteger value) {
this.testNonPositiveInteger = value;
}
/**
* Gets the value of the testNormalizedString property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTestNormalizedString() {
return testNormalizedString;
}
/**
* Sets the value of the testNormalizedString property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTestNormalizedString(String value) {
this.testNormalizedString = value;
}
/**
* Gets the value of the testPositiveInteger property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTestPositiveInteger() {
return testPositiveInteger;
}
/**
* Sets the value of the testPositiveInteger property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTestPositiveInteger(BigInteger value) {
this.testPositiveInteger = value;
}
/**
* Gets the value of the testShort property.
*
*/
public short getTestShort() {
return testShort;
}
/**
* Sets the value of the testShort property.
*
*/
public void setTestShort(short value) {
this.testShort = value;
}
/**
* Gets the value of the testString property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTestString() {
return testString;
}
/**
* Sets the value of the testString property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTestString(String value) {
this.testString = value;
}
/**
* Gets the value of the testTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTestTime() {
return testTime;
}
/**
* Sets the value of the testTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTestTime(XMLGregorianCalendar value) {
this.testTime = value;
}
/**
* Gets the value of the testToken property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTestToken() {
return testToken;
}
/**
* Sets the value of the testToken property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTestToken(String value) {
this.testToken = value;
}
/**
* Gets the value of the testUnsignedByte property.
*
*/
public short getTestUnsignedByte() {
return testUnsignedByte;
}
/**
* Sets the value of the testUnsignedByte property.
*
*/
public void setTestUnsignedByte(short value) {
this.testUnsignedByte = value;
}
/**
* Gets the value of the testUnsignedInt property.
*
*/
public long getTestUnsignedInt() {
return testUnsignedInt;
}
/**
* Sets the value of the testUnsignedInt property.
*
*/
public void setTestUnsignedInt(long value) {
this.testUnsignedInt = value;
}
/**
* Gets the value of the testUnsignedLong property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTestUnsignedLong() {
return testUnsignedLong;
}
/**
* Sets the value of the testUnsignedLong property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTestUnsignedLong(BigInteger value) {
this.testUnsignedLong = value;
}
/**
* Gets the value of the testUnsignedShort property.
*
*/
public int getTestUnsignedShort() {
return testUnsignedShort;
}
/**
* Sets the value of the testUnsignedShort property.
*
*/
public void setTestUnsignedShort(int value) {
this.testUnsignedShort = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final SimpleTypes that = ((SimpleTypes) object);
{
String leftTestAnyURI;
leftTestAnyURI = this.getTestAnyURI();
String rightTestAnyURI;
rightTestAnyURI = that.getTestAnyURI();
if (this.testAnyURI!= null) {
if (that.testAnyURI!= null) {
if (!leftTestAnyURI.equals(rightTestAnyURI)) {
return false;
}
} else {
return false;
}
} else {
if (that.testAnyURI!= null) {
return false;
}
}
}
{
byte[] leftTestBase64Binary;
leftTestBase64Binary = this.getTestBase64Binary();
byte[] rightTestBase64Binary;
rightTestBase64Binary = that.getTestBase64Binary();
if (this.testBase64Binary!= null) {
if (that.testBase64Binary!= null) {
if (!Arrays.equals(leftTestBase64Binary, rightTestBase64Binary)) {
return false;
}
} else {
return false;
}
} else {
if (that.testBase64Binary!= null) {
return false;
}
}
}
{
boolean leftTestBoolean;
leftTestBoolean = this.isTestBoolean();
boolean rightTestBoolean;
rightTestBoolean = that.isTestBoolean();
if (leftTestBoolean!= rightTestBoolean) {
return false;
}
}
{
byte leftTestByte;
leftTestByte = this.getTestByte();
byte rightTestByte;
rightTestByte = that.getTestByte();
if (leftTestByte!= rightTestByte) {
return false;
}
}
{
XMLGregorianCalendar leftTestDate;
leftTestDate = this.getTestDate();
XMLGregorianCalendar rightTestDate;
rightTestDate = that.getTestDate();
if (this.testDate!= null) {
if (that.testDate!= null) {
if (!leftTestDate.equals(rightTestDate)) {
return false;
}
} else {
return false;
}
} else {
if (that.testDate!= null) {
return false;
}
}
}
{
XMLGregorianCalendar leftTestDateTime;
leftTestDateTime = this.getTestDateTime();
XMLGregorianCalendar rightTestDateTime;
rightTestDateTime = that.getTestDateTime();
if (this.testDateTime!= null) {
if (that.testDateTime!= null) {
if (!leftTestDateTime.equals(rightTestDateTime)) {
return false;
}
} else {
return false;
}
} else {
if (that.testDateTime!= null) {
return false;
}
}
}
{
BigDecimal leftTestDecimal;
leftTestDecimal = this.getTestDecimal();
BigDecimal rightTestDecimal;
rightTestDecimal = that.getTestDecimal();
if (this.testDecimal!= null) {
if (that.testDecimal!= null) {
if (!leftTestDecimal.equals(rightTestDecimal)) {
return false;
}
} else {
return false;
}
} else {
if (that.testDecimal!= null) {
return false;
}
}
}
{
double leftTestDouble;
leftTestDouble = this.getTestDouble();
double rightTestDouble;
rightTestDouble = that.getTestDouble();
if (Double.doubleToLongBits(leftTestDouble)!= Double.doubleToLongBits(rightTestDouble)) {
return false;
}
}
{
String leftTestENTITY;
leftTestENTITY = this.getTestENTITY();
String rightTestENTITY;
rightTestENTITY = that.getTestENTITY();
if (this.testENTITY!= null) {
if (that.testENTITY!= null) {
if (!leftTestENTITY.equals(rightTestENTITY)) {
return false;
}
} else {
return false;
}
} else {
if (that.testENTITY!= null) {
return false;
}
}
}
{
float leftTestFloat;
leftTestFloat = this.getTestFloat();
float rightTestFloat;
rightTestFloat = that.getTestFloat();
if (leftTestFloat!= rightTestFloat) {
return false;
}
}
{
XMLGregorianCalendar leftTestGDay;
leftTestGDay = this.getTestGDay();
XMLGregorianCalendar rightTestGDay;
rightTestGDay = that.getTestGDay();
if (this.testGDay!= null) {
if (that.testGDay!= null) {
if (!leftTestGDay.equals(rightTestGDay)) {
return false;
}
} else {
return false;
}
} else {
if (that.testGDay!= null) {
return false;
}
}
}
{
XMLGregorianCalendar leftTestGMonth;
leftTestGMonth = this.getTestGMonth();
XMLGregorianCalendar rightTestGMonth;
rightTestGMonth = that.getTestGMonth();
if (this.testGMonth!= null) {
if (that.testGMonth!= null) {
if (!leftTestGMonth.equals(rightTestGMonth)) {
return false;
}
} else {
return false;
}
} else {
if (that.testGMonth!= null) {
return false;
}
}
}
{
XMLGregorianCalendar leftTestGYear;
leftTestGYear = this.getTestGYear();
XMLGregorianCalendar rightTestGYear;
rightTestGYear = that.getTestGYear();
if (this.testGYear!= null) {
if (that.testGYear!= null) {
if (!leftTestGYear.equals(rightTestGYear)) {
return false;
}
} else {
return false;
}
} else {
if (that.testGYear!= null) {
return false;
}
}
}
{
byte[] leftTestHexBinary;
leftTestHexBinary = this.getTestHexBinary();
byte[] rightTestHexBinary;
rightTestHexBinary = that.getTestHexBinary();
if (this.testHexBinary!= null) {
if (that.testHexBinary!= null) {
if (!Arrays.equals(leftTestHexBinary, rightTestHexBinary)) {
return false;
}
} else {
return false;
}
} else {
if (that.testHexBinary!= null) {
return false;
}
}
}
{
int leftTestInt;
leftTestInt = this.getTestInt();
int rightTestInt;
rightTestInt = that.getTestInt();
if (leftTestInt!= rightTestInt) {
return false;
}
}
{
BigInteger leftTestInteger;
leftTestInteger = this.getTestInteger();
BigInteger rightTestInteger;
rightTestInteger = that.getTestInteger();
if (this.testInteger!= null) {
if (that.testInteger!= null) {
if (!leftTestInteger.equals(rightTestInteger)) {
return false;
}
} else {
return false;
}
} else {
if (that.testInteger!= null) {
return false;
}
}
}
{
long leftTestLong;
leftTestLong = this.getTestLong();
long rightTestLong;
rightTestLong = that.getTestLong();
if (leftTestLong!= rightTestLong) {
return false;
}
}
{
String leftTestName;
leftTestName = this.getTestName();
String rightTestName;
rightTestName = that.getTestName();
if (this.testName!= null) {
if (that.testName!= null) {
if (!leftTestName.equals(rightTestName)) {
return false;
}
} else {
return false;
}
} else {
if (that.testName!= null) {
return false;
}
}
}
{
String leftTestNCName;
leftTestNCName = this.getTestNCName();
String rightTestNCName;
rightTestNCName = that.getTestNCName();
if (this.testNCName!= null) {
if (that.testNCName!= null) {
if (!leftTestNCName.equals(rightTestNCName)) {
return false;
}
} else {
return false;
}
} else {
if (that.testNCName!= null) {
return false;
}
}
}
{
BigInteger leftTestNegativeInteger;
leftTestNegativeInteger = this.getTestNegativeInteger();
BigInteger rightTestNegativeInteger;
rightTestNegativeInteger = that.getTestNegativeInteger();
if (this.testNegativeInteger!= null) {
if (that.testNegativeInteger!= null) {
if (!leftTestNegativeInteger.equals(rightTestNegativeInteger)) {
return false;
}
} else {
return false;
}
} else {
if (that.testNegativeInteger!= null) {
return false;
}
}
}
{
BigInteger leftTestNonNegativeInteger;
leftTestNonNegativeInteger = this.getTestNonNegativeInteger();
BigInteger rightTestNonNegativeInteger;
rightTestNonNegativeInteger = that.getTestNonNegativeInteger();
if (this.testNonNegativeInteger!= null) {
if (that.testNonNegativeInteger!= null) {
if (!leftTestNonNegativeInteger.equals(rightTestNonNegativeInteger)) {
return false;
}
} else {
return false;
}
} else {
if (that.testNonNegativeInteger!= null) {
return false;
}
}
}
{
BigInteger leftTestNonPositiveInteger;
leftTestNonPositiveInteger = this.getTestNonPositiveInteger();
BigInteger rightTestNonPositiveInteger;
rightTestNonPositiveInteger = that.getTestNonPositiveInteger();
if (this.testNonPositiveInteger!= null) {
if (that.testNonPositiveInteger!= null) {
if (!leftTestNonPositiveInteger.equals(rightTestNonPositiveInteger)) {
return false;
}
} else {
return false;
}
} else {
if (that.testNonPositiveInteger!= null) {
return false;
}
}
}
{
String leftTestNormalizedString;
leftTestNormalizedString = this.getTestNormalizedString();
String rightTestNormalizedString;
rightTestNormalizedString = that.getTestNormalizedString();
if (this.testNormalizedString!= null) {
if (that.testNormalizedString!= null) {
if (!leftTestNormalizedString.equals(rightTestNormalizedString)) {
return false;
}
} else {
return false;
}
} else {
if (that.testNormalizedString!= null) {
return false;
}
}
}
{
BigInteger leftTestPositiveInteger;
leftTestPositiveInteger = this.getTestPositiveInteger();
BigInteger rightTestPositiveInteger;
rightTestPositiveInteger = that.getTestPositiveInteger();
if (this.testPositiveInteger!= null) {
if (that.testPositiveInteger!= null) {
if (!leftTestPositiveInteger.equals(rightTestPositiveInteger)) {
return false;
}
} else {
return false;
}
} else {
if (that.testPositiveInteger!= null) {
return false;
}
}
}
{
short leftTestShort;
leftTestShort = this.getTestShort();
short rightTestShort;
rightTestShort = that.getTestShort();
if (leftTestShort!= rightTestShort) {
return false;
}
}
{
String leftTestString;
leftTestString = this.getTestString();
String rightTestString;
rightTestString = that.getTestString();
if (this.testString!= null) {
if (that.testString!= null) {
if (!leftTestString.equals(rightTestString)) {
return false;
}
} else {
return false;
}
} else {
if (that.testString!= null) {
return false;
}
}
}
{
XMLGregorianCalendar leftTestTime;
leftTestTime = this.getTestTime();
XMLGregorianCalendar rightTestTime;
rightTestTime = that.getTestTime();
if (this.testTime!= null) {
if (that.testTime!= null) {
if (!leftTestTime.equals(rightTestTime)) {
return false;
}
} else {
return false;
}
} else {
if (that.testTime!= null) {
return false;
}
}
}
{
String leftTestToken;
leftTestToken = this.getTestToken();
String rightTestToken;
rightTestToken = that.getTestToken();
if (this.testToken!= null) {
if (that.testToken!= null) {
if (!leftTestToken.equals(rightTestToken)) {
return false;
}
} else {
return false;
}
} else {
if (that.testToken!= null) {
return false;
}
}
}
{
short leftTestUnsignedByte;
leftTestUnsignedByte = this.getTestUnsignedByte();
short rightTestUnsignedByte;
rightTestUnsignedByte = that.getTestUnsignedByte();
if (leftTestUnsignedByte!= rightTestUnsignedByte) {
return false;
}
}
{
long leftTestUnsignedInt;
leftTestUnsignedInt = this.getTestUnsignedInt();
long rightTestUnsignedInt;
rightTestUnsignedInt = that.getTestUnsignedInt();
if (leftTestUnsignedInt!= rightTestUnsignedInt) {
return false;
}
}
{
BigInteger leftTestUnsignedLong;
leftTestUnsignedLong = this.getTestUnsignedLong();
BigInteger rightTestUnsignedLong;
rightTestUnsignedLong = that.getTestUnsignedLong();
if (this.testUnsignedLong!= null) {
if (that.testUnsignedLong!= null) {
if (!leftTestUnsignedLong.equals(rightTestUnsignedLong)) {
return false;
}
} else {
return false;
}
} else {
if (that.testUnsignedLong!= null) {
return false;
}
}
}
{
int leftTestUnsignedShort;
leftTestUnsignedShort = this.getTestUnsignedShort();
int rightTestUnsignedShort;
rightTestUnsignedShort = that.getTestUnsignedShort();
if (leftTestUnsignedShort!= rightTestUnsignedShort) {
return false;
}
}
return true;
}
@Override
public Struct toStruct() {
Struct struct = new Struct(CONNECT_SCHEMA);
ConnectableHelper.toString(struct, "test_anyURI", this.testAnyURI);
ConnectableHelper.toBytes(struct, "test_base64Binary", this.testBase64Binary);
ConnectableHelper.toBoolean(struct, "test_boolean", this.testBoolean);
ConnectableHelper.toInt8(struct, "test_byte", this.testByte);
ConnectableHelper.toDate(struct, "test_date", this.testDate);
ConnectableHelper.toDateTime(struct, "test_dateTime", this.testDateTime);
ConnectableHelper.toDecimal(struct, "test_decimal", this.testDecimal);
ConnectableHelper.toFloat64(struct, "test_double", this.testDouble);
ConnectableHelper.toString(struct, "test_ENTITY", this.testENTITY);
ConnectableHelper.toFloat32(struct, "test_float", this.testFloat);
ConnectableHelper.toXmlgDay(struct, "test_gDay", this.testGDay);
ConnectableHelper.toXmlgMonth(struct, "test_gMonth", this.testGMonth);
ConnectableHelper.toXmlgYear(struct, "test_gYear", this.testGYear);
ConnectableHelper.toBytes(struct, "test_hexBinary", this.testHexBinary);
ConnectableHelper.toInt32(struct, "test_int", this.testInt);
ConnectableHelper.toInt64(struct, "test_integer", this.testInteger);
ConnectableHelper.toInt64(struct, "test_long", this.testLong);
ConnectableHelper.toString(struct, "test_Name", this.testName);
ConnectableHelper.toString(struct, "test_NCName", this.testNCName);
ConnectableHelper.toInt64(struct, "test_negativeInteger", this.testNegativeInteger);
ConnectableHelper.toInt64(struct, "test_nonNegativeInteger", this.testNonNegativeInteger);
ConnectableHelper.toInt64(struct, "test_nonPositiveInteger", this.testNonPositiveInteger);
ConnectableHelper.toString(struct, "test_normalizedString", this.testNormalizedString);
ConnectableHelper.toInt64(struct, "test_positiveInteger", this.testPositiveInteger);
ConnectableHelper.toInt16(struct, "test_short", this.testShort);
ConnectableHelper.toString(struct, "test_string", this.testString);
ConnectableHelper.toTime(struct, "test_time", this.testTime);
ConnectableHelper.toString(struct, "test_token", this.testToken);
ConnectableHelper.toInt16(struct, "test_unsignedByte", this.testUnsignedByte);
ConnectableHelper.toInt64(struct, "test_unsignedInt", this.testUnsignedInt);
ConnectableHelper.toInt64(struct, "test_unsignedLong", this.testUnsignedLong);
ConnectableHelper.toInt32(struct, "test_unsignedShort", this.testUnsignedShort);
return struct;
}
@Override
public void fromStruct(Struct struct) {
this.testAnyURI = ConnectableHelper.fromString(struct, "test_anyURI");
this.testBase64Binary = ConnectableHelper.fromBytes(struct, "test_base64Binary");
this.testBoolean = ConnectableHelper.fromBoolean(struct, "test_boolean");
this.testByte = ConnectableHelper.fromInt8(struct, "test_byte");
this.testDate = ConnectableHelper.fromDate(struct, "test_date");
this.testDateTime = ConnectableHelper.fromDateTime(struct, "test_dateTime");
this.testDecimal = ConnectableHelper.fromDecimal(struct, "test_decimal");
this.testDouble = ConnectableHelper.fromFloat64(struct, "test_double");
this.testENTITY = ConnectableHelper.fromString(struct, "test_ENTITY");
this.testFloat = ConnectableHelper.fromFloat32(struct, "test_float");
this.testGDay = ConnectableHelper.fromXmlgDay(struct, "test_gDay");
this.testGMonth = ConnectableHelper.fromXmlgMonth(struct, "test_gMonth");
this.testGYear = ConnectableHelper.fromXmlgYear(struct, "test_gYear");
this.testHexBinary = ConnectableHelper.fromBytes(struct, "test_hexBinary");
this.testInt = ConnectableHelper.fromInt32(struct, "test_int");
this.testInteger = ConnectableHelper.fromInt64BigInteger(struct, "test_integer");
this.testLong = ConnectableHelper.fromInt64(struct, "test_long");
this.testName = ConnectableHelper.fromString(struct, "test_Name");
this.testNCName = ConnectableHelper.fromString(struct, "test_NCName");
this.testNegativeInteger = ConnectableHelper.fromInt64BigInteger(struct, "test_negativeInteger");
this.testNonNegativeInteger = ConnectableHelper.fromInt64BigInteger(struct, "test_nonNegativeInteger");
this.testNonPositiveInteger = ConnectableHelper.fromInt64BigInteger(struct, "test_nonPositiveInteger");
this.testNormalizedString = ConnectableHelper.fromString(struct, "test_normalizedString");
this.testPositiveInteger = ConnectableHelper.fromInt64BigInteger(struct, "test_positiveInteger");
this.testShort = ConnectableHelper.fromInt16(struct, "test_short");
this.testString = ConnectableHelper.fromString(struct, "test_string");
this.testTime = ConnectableHelper.fromTime(struct, "test_time");
this.testToken = ConnectableHelper.fromString(struct, "test_token");
this.testUnsignedByte = ConnectableHelper.fromInt16(struct, "test_unsignedByte");
this.testUnsignedInt = ConnectableHelper.fromInt64(struct, "test_unsignedInt");
this.testUnsignedLong = ConnectableHelper.fromInt64BigInteger(struct, "test_unsignedLong");
this.testUnsignedShort = ConnectableHelper.fromInt32(struct, "test_unsignedShort");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy