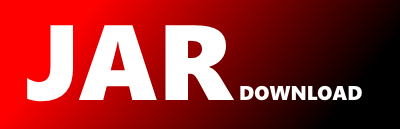
test.BookForm Maven / Gradle / Ivy
The newest version!
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.09.09 at 03:21:19 PM UTC
//
package test;
import java.math.BigDecimal;
import java.math.BigInteger;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
import com.github.jcustenborder.kafka.connect.xml.Connectable;
import com.github.jcustenborder.kafka.connect.xml.ConnectableHelper;
import org.apache.kafka.connect.data.Date;
import org.apache.kafka.connect.data.Decimal;
import org.apache.kafka.connect.data.Schema;
import org.apache.kafka.connect.data.SchemaBuilder;
import org.apache.kafka.connect.data.Struct;
import org.apache.kafka.connect.data.Timestamp;
/**
* Java class for BookForm complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="BookForm">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="testBoolean" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="testByte" type="{http://www.w3.org/2001/XMLSchema}byte"/>
* <element name="testDate" type="{http://www.w3.org/2001/XMLSchema}date"/>
* <element name="testDateTime" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="testDecimal" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* <element name="testDouble" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="testFloat" type="{http://www.w3.org/2001/XMLSchema}float"/>
* <element name="testInt" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="testInteger" type="{http://www.w3.org/2001/XMLSchema}integer"/>
* <element name="testShort" type="{http://www.w3.org/2001/XMLSchema}short"/>
* </sequence>
* <attribute name="id" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "BookForm", propOrder = {
"testBoolean",
"testByte",
"testDate",
"testDateTime",
"testDecimal",
"testDouble",
"testFloat",
"testInt",
"testInteger",
"testShort"
})
public class BookForm
implements Connectable
{
protected boolean testBoolean;
protected byte testByte;
@XmlElement(required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar testDate;
@XmlElement(required = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar testDateTime;
@XmlElement(required = true)
protected BigDecimal testDecimal;
protected double testDouble;
protected float testFloat;
protected int testInt;
@XmlElement(required = true)
protected BigInteger testInteger;
protected short testShort;
@XmlAttribute(name = "id")
protected String id;
public final static Schema CONNECT_SCHEMA;
static {
SchemaBuilder builder = SchemaBuilder.struct();
builder.name("test.BookForm");
builder.optional();
SchemaBuilder fieldBuilder;
fieldBuilder = SchemaBuilder.bool();
fieldBuilder.optional();
builder.field("testBoolean", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int8();
fieldBuilder.optional();
builder.field("testByte", fieldBuilder.build());
fieldBuilder = Date.builder();
builder.field("testDate", fieldBuilder.build());
fieldBuilder = Timestamp.builder();
builder.field("testDateTime", fieldBuilder.build());
fieldBuilder = Decimal.builder(12);
builder.field("testDecimal", fieldBuilder.build());
fieldBuilder = SchemaBuilder.float64();
fieldBuilder.optional();
builder.field("testDouble", fieldBuilder.build());
fieldBuilder = SchemaBuilder.float32();
fieldBuilder.optional();
builder.field("testFloat", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int32();
fieldBuilder.optional();
builder.field("testInt", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int64();
builder.field("testInteger", fieldBuilder.build());
fieldBuilder = SchemaBuilder.int16();
fieldBuilder.optional();
builder.field("testShort", fieldBuilder.build());
fieldBuilder = SchemaBuilder.string();
fieldBuilder.optional();
builder.field("id", fieldBuilder.build());
CONNECT_SCHEMA = builder.build();
}
/**
* Gets the value of the testBoolean property.
*
*/
public boolean isTestBoolean() {
return testBoolean;
}
/**
* Sets the value of the testBoolean property.
*
*/
public void setTestBoolean(boolean value) {
this.testBoolean = value;
}
/**
* Gets the value of the testByte property.
*
*/
public byte getTestByte() {
return testByte;
}
/**
* Sets the value of the testByte property.
*
*/
public void setTestByte(byte value) {
this.testByte = value;
}
/**
* Gets the value of the testDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTestDate() {
return testDate;
}
/**
* Sets the value of the testDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTestDate(XMLGregorianCalendar value) {
this.testDate = value;
}
/**
* Gets the value of the testDateTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTestDateTime() {
return testDateTime;
}
/**
* Sets the value of the testDateTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTestDateTime(XMLGregorianCalendar value) {
this.testDateTime = value;
}
/**
* Gets the value of the testDecimal property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTestDecimal() {
return testDecimal;
}
/**
* Sets the value of the testDecimal property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTestDecimal(BigDecimal value) {
this.testDecimal = value;
}
/**
* Gets the value of the testDouble property.
*
*/
public double getTestDouble() {
return testDouble;
}
/**
* Sets the value of the testDouble property.
*
*/
public void setTestDouble(double value) {
this.testDouble = value;
}
/**
* Gets the value of the testFloat property.
*
*/
public float getTestFloat() {
return testFloat;
}
/**
* Sets the value of the testFloat property.
*
*/
public void setTestFloat(float value) {
this.testFloat = value;
}
/**
* Gets the value of the testInt property.
*
*/
public int getTestInt() {
return testInt;
}
/**
* Sets the value of the testInt property.
*
*/
public void setTestInt(int value) {
this.testInt = value;
}
/**
* Gets the value of the testInteger property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTestInteger() {
return testInteger;
}
/**
* Sets the value of the testInteger property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTestInteger(BigInteger value) {
this.testInteger = value;
}
/**
* Gets the value of the testShort property.
*
*/
public short getTestShort() {
return testShort;
}
/**
* Sets the value of the testShort property.
*
*/
public void setTestShort(short value) {
this.testShort = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final BookForm that = ((BookForm) object);
{
boolean leftTestBoolean;
leftTestBoolean = this.isTestBoolean();
boolean rightTestBoolean;
rightTestBoolean = that.isTestBoolean();
if (leftTestBoolean!= rightTestBoolean) {
return false;
}
}
{
byte leftTestByte;
leftTestByte = this.getTestByte();
byte rightTestByte;
rightTestByte = that.getTestByte();
if (leftTestByte!= rightTestByte) {
return false;
}
}
{
XMLGregorianCalendar leftTestDate;
leftTestDate = this.getTestDate();
XMLGregorianCalendar rightTestDate;
rightTestDate = that.getTestDate();
if (this.testDate!= null) {
if (that.testDate!= null) {
if (!leftTestDate.equals(rightTestDate)) {
return false;
}
} else {
return false;
}
} else {
if (that.testDate!= null) {
return false;
}
}
}
{
XMLGregorianCalendar leftTestDateTime;
leftTestDateTime = this.getTestDateTime();
XMLGregorianCalendar rightTestDateTime;
rightTestDateTime = that.getTestDateTime();
if (this.testDateTime!= null) {
if (that.testDateTime!= null) {
if (!leftTestDateTime.equals(rightTestDateTime)) {
return false;
}
} else {
return false;
}
} else {
if (that.testDateTime!= null) {
return false;
}
}
}
{
BigDecimal leftTestDecimal;
leftTestDecimal = this.getTestDecimal();
BigDecimal rightTestDecimal;
rightTestDecimal = that.getTestDecimal();
if (this.testDecimal!= null) {
if (that.testDecimal!= null) {
if (!leftTestDecimal.equals(rightTestDecimal)) {
return false;
}
} else {
return false;
}
} else {
if (that.testDecimal!= null) {
return false;
}
}
}
{
double leftTestDouble;
leftTestDouble = this.getTestDouble();
double rightTestDouble;
rightTestDouble = that.getTestDouble();
if (Double.doubleToLongBits(leftTestDouble)!= Double.doubleToLongBits(rightTestDouble)) {
return false;
}
}
{
float leftTestFloat;
leftTestFloat = this.getTestFloat();
float rightTestFloat;
rightTestFloat = that.getTestFloat();
if (leftTestFloat!= rightTestFloat) {
return false;
}
}
{
int leftTestInt;
leftTestInt = this.getTestInt();
int rightTestInt;
rightTestInt = that.getTestInt();
if (leftTestInt!= rightTestInt) {
return false;
}
}
{
BigInteger leftTestInteger;
leftTestInteger = this.getTestInteger();
BigInteger rightTestInteger;
rightTestInteger = that.getTestInteger();
if (this.testInteger!= null) {
if (that.testInteger!= null) {
if (!leftTestInteger.equals(rightTestInteger)) {
return false;
}
} else {
return false;
}
} else {
if (that.testInteger!= null) {
return false;
}
}
}
{
short leftTestShort;
leftTestShort = this.getTestShort();
short rightTestShort;
rightTestShort = that.getTestShort();
if (leftTestShort!= rightTestShort) {
return false;
}
}
{
String leftId;
leftId = this.getId();
String rightId;
rightId = that.getId();
if (this.id!= null) {
if (that.id!= null) {
if (!leftId.equals(rightId)) {
return false;
}
} else {
return false;
}
} else {
if (that.id!= null) {
return false;
}
}
}
return true;
}
@Override
public Struct toStruct() {
Struct struct = new Struct(CONNECT_SCHEMA);
ConnectableHelper.toBoolean(struct, "testBoolean", this.testBoolean);
ConnectableHelper.toInt8(struct, "testByte", this.testByte);
ConnectableHelper.toDate(struct, "testDate", this.testDate);
ConnectableHelper.toDateTime(struct, "testDateTime", this.testDateTime);
ConnectableHelper.toDecimal(struct, "testDecimal", this.testDecimal);
ConnectableHelper.toFloat64(struct, "testDouble", this.testDouble);
ConnectableHelper.toFloat32(struct, "testFloat", this.testFloat);
ConnectableHelper.toInt32(struct, "testInt", this.testInt);
ConnectableHelper.toInt64(struct, "testInteger", this.testInteger);
ConnectableHelper.toInt16(struct, "testShort", this.testShort);
ConnectableHelper.toString(struct, "id", this.id);
return struct;
}
@Override
public void fromStruct(Struct struct) {
this.testBoolean = ConnectableHelper.fromBoolean(struct, "testBoolean");
this.testByte = ConnectableHelper.fromInt8(struct, "testByte");
this.testDate = ConnectableHelper.fromDate(struct, "testDate");
this.testDateTime = ConnectableHelper.fromDateTime(struct, "testDateTime");
this.testDecimal = ConnectableHelper.fromDecimal(struct, "testDecimal");
this.testDouble = ConnectableHelper.fromFloat64(struct, "testDouble");
this.testFloat = ConnectableHelper.fromFloat32(struct, "testFloat");
this.testInt = ConnectableHelper.fromInt32(struct, "testInt");
this.testInteger = ConnectableHelper.fromInt64BigInteger(struct, "testInteger");
this.testShort = ConnectableHelper.fromInt16(struct, "testShort");
this.id = ConnectableHelper.fromString(struct, "id");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy