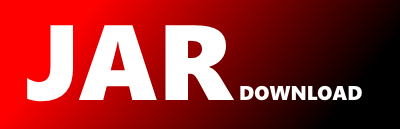
com.github.jcustenborder.netty.syslog.ImmutableSyslogRequest Maven / Gradle / Ivy
package com.github.jcustenborder.netty.syslog;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.net.InetAddress;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Generated;
/**
* Immutable implementation of {@link SyslogRequest}.
*
* Use the builder to create immutable instances:
* {@code ImmutableSyslogRequest.builder()}.
*/
@SuppressWarnings({"all"})
@Generated({"Immutables.generator", "SyslogRequest"})
final class ImmutableSyslogRequest implements SyslogRequest {
private final LocalDateTime receivedDate;
private final InetAddress remoteAddress;
private final String rawMessage;
private ImmutableSyslogRequest(
LocalDateTime receivedDate,
InetAddress remoteAddress,
String rawMessage) {
this.receivedDate = receivedDate;
this.remoteAddress = remoteAddress;
this.rawMessage = rawMessage;
}
/**
* @return The value of the {@code receivedDate} attribute
*/
@JsonProperty(required = true)
@Override
public LocalDateTime receivedDate() {
return receivedDate;
}
/**
* @return The value of the {@code remoteAddress} attribute
*/
@JsonProperty(required = true)
@Override
public InetAddress remoteAddress() {
return remoteAddress;
}
/**
* @return The value of the {@code rawMessage} attribute
*/
@JsonProperty(required = true)
@Override
public String rawMessage() {
return rawMessage;
}
/**
* Copy the current immutable object by setting a value for the {@link SyslogRequest#receivedDate() receivedDate} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for receivedDate
* @return A modified copy of the {@code this} object
*/
public final ImmutableSyslogRequest withReceivedDate(LocalDateTime value) {
if (this.receivedDate == value) return this;
LocalDateTime newValue = Objects.requireNonNull(value, "receivedDate");
return new ImmutableSyslogRequest(newValue, this.remoteAddress, this.rawMessage);
}
/**
* Copy the current immutable object by setting a value for the {@link SyslogRequest#remoteAddress() remoteAddress} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for remoteAddress
* @return A modified copy of the {@code this} object
*/
public final ImmutableSyslogRequest withRemoteAddress(InetAddress value) {
if (this.remoteAddress == value) return this;
InetAddress newValue = Objects.requireNonNull(value, "remoteAddress");
return new ImmutableSyslogRequest(this.receivedDate, newValue, this.rawMessage);
}
/**
* Copy the current immutable object by setting a value for the {@link SyslogRequest#rawMessage() rawMessage} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for rawMessage
* @return A modified copy of the {@code this} object
*/
public final ImmutableSyslogRequest withRawMessage(String value) {
if (this.rawMessage.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "rawMessage");
return new ImmutableSyslogRequest(this.receivedDate, this.remoteAddress, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableSyslogRequest} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableSyslogRequest
&& equalTo((ImmutableSyslogRequest) another);
}
private boolean equalTo(ImmutableSyslogRequest another) {
return receivedDate.equals(another.receivedDate)
&& remoteAddress.equals(another.remoteAddress)
&& rawMessage.equals(another.rawMessage);
}
/**
* Computes a hash code from attributes: {@code receivedDate}, {@code remoteAddress}, {@code rawMessage}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + receivedDate.hashCode();
h += (h << 5) + remoteAddress.hashCode();
h += (h << 5) + rawMessage.hashCode();
return h;
}
/**
* Prints the immutable value {@code SyslogRequest} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "SyslogRequest{"
+ "receivedDate=" + receivedDate
+ ", remoteAddress=" + remoteAddress
+ ", rawMessage=" + rawMessage
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements SyslogRequest {
LocalDateTime receivedDate;
InetAddress remoteAddress;
String rawMessage;
@JsonProperty(required = true)
public void setReceivedDate(LocalDateTime receivedDate) {
this.receivedDate = receivedDate;
}
@JsonProperty(required = true)
public void setRemoteAddress(InetAddress remoteAddress) {
this.remoteAddress = remoteAddress;
}
@JsonProperty(required = true)
public void setRawMessage(String rawMessage) {
this.rawMessage = rawMessage;
}
@Override
public LocalDateTime receivedDate() { throw new UnsupportedOperationException(); }
@Override
public InetAddress remoteAddress() { throw new UnsupportedOperationException(); }
@Override
public String rawMessage() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableSyslogRequest fromJson(Json json) {
ImmutableSyslogRequest.Builder builder = ImmutableSyslogRequest.builder();
if (json.receivedDate != null) {
builder.receivedDate(json.receivedDate);
}
if (json.remoteAddress != null) {
builder.remoteAddress(json.remoteAddress);
}
if (json.rawMessage != null) {
builder.rawMessage(json.rawMessage);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link SyslogRequest} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SyslogRequest instance
*/
public static ImmutableSyslogRequest copyOf(SyslogRequest instance) {
if (instance instanceof ImmutableSyslogRequest) {
return (ImmutableSyslogRequest) instance;
}
return ImmutableSyslogRequest.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableSyslogRequest ImmutableSyslogRequest}.
* @return A new ImmutableSyslogRequest builder
*/
public static ImmutableSyslogRequest.Builder builder() {
return new ImmutableSyslogRequest.Builder();
}
/**
* Builds instances of type {@link ImmutableSyslogRequest ImmutableSyslogRequest}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_RECEIVED_DATE = 0x1L;
private static final long INIT_BIT_REMOTE_ADDRESS = 0x2L;
private static final long INIT_BIT_RAW_MESSAGE = 0x4L;
private long initBits = 0x7L;
private LocalDateTime receivedDate;
private InetAddress remoteAddress;
private String rawMessage;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code SyslogRequest} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SyslogRequest instance) {
Objects.requireNonNull(instance, "instance");
receivedDate(instance.receivedDate());
remoteAddress(instance.remoteAddress());
rawMessage(instance.rawMessage());
return this;
}
/**
* Initializes the value for the {@link SyslogRequest#receivedDate() receivedDate} attribute.
* @param receivedDate The value for receivedDate
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty(required = true)
public final Builder receivedDate(LocalDateTime receivedDate) {
this.receivedDate = Objects.requireNonNull(receivedDate, "receivedDate");
initBits &= ~INIT_BIT_RECEIVED_DATE;
return this;
}
/**
* Initializes the value for the {@link SyslogRequest#remoteAddress() remoteAddress} attribute.
* @param remoteAddress The value for remoteAddress
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty(required = true)
public final Builder remoteAddress(InetAddress remoteAddress) {
this.remoteAddress = Objects.requireNonNull(remoteAddress, "remoteAddress");
initBits &= ~INIT_BIT_REMOTE_ADDRESS;
return this;
}
/**
* Initializes the value for the {@link SyslogRequest#rawMessage() rawMessage} attribute.
* @param rawMessage The value for rawMessage
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty(required = true)
public final Builder rawMessage(String rawMessage) {
this.rawMessage = Objects.requireNonNull(rawMessage, "rawMessage");
initBits &= ~INIT_BIT_RAW_MESSAGE;
return this;
}
/**
* Builds a new {@link ImmutableSyslogRequest ImmutableSyslogRequest}.
* @return An immutable instance of SyslogRequest
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableSyslogRequest build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableSyslogRequest(receivedDate, remoteAddress, rawMessage);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList();
if ((initBits & INIT_BIT_RECEIVED_DATE) != 0) attributes.add("receivedDate");
if ((initBits & INIT_BIT_REMOTE_ADDRESS) != 0) attributes.add("remoteAddress");
if ((initBits & INIT_BIT_RAW_MESSAGE) != 0) attributes.add("rawMessage");
return "Cannot build SyslogRequest, some of required attributes are not set " + attributes;
}
}
}