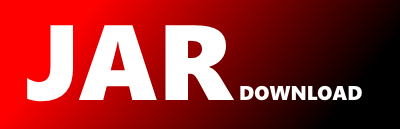
com.couchbase.CBArray Maven / Gradle / Ivy
/*
* // Copyright (c) 2015 Couchbase, Inc.
* // Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
* // except in compliance with the License. You may obtain a copy of the License at
* // http://www.apache.org/licenses/LICENSE-2.0
* // Unless required by applicable law or agreed to in writing, software distributed under the
* // License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* // either express or implied. See the License for the specific language governing permissions
* // and limitations under the License.
*/
package com.couchbase;
import org.boon.json.JsonFactory;
import java.sql.*;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by davec on 2015-07-21.
*/
class CBArray implements Array
{
private String baseType;
private Object []array;
private String jsonArray;
@SuppressWarnings("unchecked")
private static final Map typeMap = new HashMap();
static
{
typeMap.put("byte" ,"number");
typeMap.put("Byte" ,"number");
typeMap.put("short","number");
typeMap.put("Short","number");
typeMap.put("int","number");
typeMap.put("Integer","number");
typeMap.put("long","number");
typeMap.put("Long","number");
typeMap.put("BigDecimal", "number");
typeMap.put("Boolean","Boolean");
typeMap.put("String", "string");
}
CBArray(List arrayList)
{
if (arrayList == null )
{
array=null;
jsonArray=null;
}
else
{
array = arrayList.toArray();
jsonArray = JsonFactory.toJson(array);
}
}
CBArray(String typeName, Object[] array)
{
this.array = array;
baseType = typeName;
jsonArray = JsonFactory.toJson(array);
}
/**
* Retrieves the SQL type name of the elements in
* the array designated by this Array
object.
* If the elements are a built-in type, it returns
* the database-specific type name of the elements.
* If the elements are a user-defined type (UDT),
* this method returns the fully-qualified SQL type name.
*
* @return a String
that is the database-specific
* name for a built-in base type; or the fully-qualified SQL type
* name for a base type that is a UDT
* @throws SQLException if an error occurs while attempting
* to access the type name
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public String getBaseTypeName() throws SQLException
{
return baseType;
}
/**
* Retrieves the JDBC type of the elements in the array designated
* by this Array
object.
*
* @return a constant from the class {@link Types} that is
* the type code for the elements in the array designated by this
* Array
object
* @throws SQLException if an error occurs while attempting
* to access the base type
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public int getBaseType() throws SQLException
{
String jsonType = typeMap.get(baseType);
if (jsonType == null )
{
return Types.JAVA_OBJECT;
}
else if (jsonType.compareTo("number") == 0)
{
return Types.NUMERIC;
}
else if (jsonType.compareTo("Boolean") == 0)
{
return Types.BOOLEAN;
}
else if (jsonType.compareTo("String") == 0)
{
return Types.VARCHAR;
}
else return Types.JAVA_OBJECT;
}
/**
* Retrieves the contents of the SQL ARRAY
value designated
* by this
* Array
object in the form of an array in the Java
* programming language. This version of the method getArray
* uses the type map associated with the connection for customizations of
* the type mappings.
*
* Note: When getArray
is used to materialize
* a base type that maps to a primitive data type, then it is
* implementation-defined whether the array returned is an array of
* that primitive data type or an array of Object
.
*
* @return an array in the Java programming language that contains
* the ordered elements of the SQL ARRAY
value
* designated by this Array
object
* @throws SQLException if an error occurs while attempting to
* access the array
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public Object getArray() throws SQLException
{
return array;
}
/**
* Retrieves the contents of the SQL ARRAY
value designated by this
* Array
object.
* This method uses
* the specified map
for type map customizations
* unless the base type of the array does not match a user-defined
* type in map
, in which case it
* uses the standard mapping. This version of the method
* getArray
uses either the given type map or the standard mapping;
* it never uses the type map associated with the connection.
*
* Note: When getArray
is used to materialize
* a base type that maps to a primitive data type, then it is
* implementation-defined whether the array returned is an array of
* that primitive data type or an array of Object
.
*
* @param map a java.util.Map
object that contains mappings
* of SQL type names to classes in the Java programming language
* @return an array in the Java programming language that contains the ordered
* elements of the SQL array designated by this object
* @throws SQLException if an error occurs while attempting to
* access the array
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public Object getArray(Map> map) throws SQLException
{
throw CBDriver.notImplemented(CBArray.class,"getArray");
}
/**
* Retrieves a slice of the SQL ARRAY
* value designated by this Array
object, beginning with the
* specified index
and containing up to count
* successive elements of the SQL array. This method uses the type map
* associated with the connection for customizations of the type mappings.
*
* Note: When getArray
is used to materialize
* a base type that maps to a primitive data type, then it is
* implementation-defined whether the array returned is an array of
* that primitive data type or an array of Object
.
*
* @param index the array index of the first element to retrieve;
* the first element is at index 1
* @param count the number of successive SQL array elements to retrieve
* @return an array containing up to count
consecutive elements
* of the SQL array, beginning with element index
* @throws SQLException if an error occurs while attempting to
* access the array
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public Object getArray(long index, int count) throws SQLException
{
Object []newArray = new Object[count];
for (int i=(int)index, j=0; j < count;j++)
{
newArray[j] = array[i++];
}
return newArray;
}
/**
* Retrieves a slice of the SQL ARRAY
value
* designated by this Array
object, beginning with the specified
* index
and containing up to count
* successive elements of the SQL array.
*
* This method uses
* the specified map
for type map customizations
* unless the base type of the array does not match a user-defined
* type in map
, in which case it
* uses the standard mapping. This version of the method
* getArray
uses either the given type map or the standard mapping;
* it never uses the type map associated with the connection.
*
* Note: When getArray
is used to materialize
* a base type that maps to a primitive data type, then it is
* implementation-defined whether the array returned is an array of
* that primitive data type or an array of Object
.
*
* @param index the array index of the first element to retrieve;
* the first element is at index 1
* @param count the number of successive SQL array elements to
* retrieve
* @param map a java.util.Map
object
* that contains SQL type names and the classes in
* the Java programming language to which they are mapped
* @return an array containing up to count
* consecutive elements of the SQL ARRAY
value designated by this
* Array
object, beginning with element
* index
* @throws SQLException if an error occurs while attempting to
* access the array
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public Object getArray(long index, int count, Map> map) throws SQLException
{
throw CBDriver.notImplemented(CBArray.class,"getArray");
}
/**
* Retrieves a result set that contains the elements of the SQL
* ARRAY
value
* designated by this Array
object. If appropriate,
* the elements of the array are mapped using the connection's type
* map; otherwise, the standard mapping is used.
*
* The result set contains one row for each array element, with
* two columns in each row. The second column stores the element
* value; the first column stores the index into the array for
* that element (with the first array element being at index 1).
* The rows are in ascending order corresponding to
* the order of the indices.
*
* @return a {@link ResultSet} object containing one row for each
* of the elements in the array designated by this Array
* object, with the rows in ascending order based on the indices.
* @throws SQLException if an error occurs while attempting to
* access the array
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public ResultSet getResultSet() throws SQLException
{
throw CBDriver.notImplemented(CBArray.class,"getResultSet");
}
/**
* Retrieves a result set that contains the elements of the SQL
* ARRAY
value designated by this Array
object.
* This method uses
* the specified map
for type map customizations
* unless the base type of the array does not match a user-defined
* type in map
, in which case it
* uses the standard mapping. This version of the method
* getResultSet
uses either the given type map or the standard mapping;
* it never uses the type map associated with the connection.
*
* The result set contains one row for each array element, with
* two columns in each row. The second column stores the element
* value; the first column stores the index into the array for
* that element (with the first array element being at index 1).
* The rows are in ascending order corresponding to
* the order of the indices.
*
* @param map contains the mapping of SQL user-defined types to
* classes in the Java programming language
* @return a ResultSet
object containing one row for each
* of the elements in the array designated by this Array
* object, with the rows in ascending order based on the indices.
* @throws SQLException if an error occurs while attempting to
* access the array
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public ResultSet getResultSet(Map> map) throws SQLException
{
throw CBDriver.notImplemented(CBArray.class,"getResultSet");
}
/**
* Retrieves a result set holding the elements of the subarray that
* starts at index index
and contains up to
* count
successive elements. This method uses
* the connection's type map to map the elements of the array if
* the map contains an entry for the base type. Otherwise, the
* standard mapping is used.
*
* The result set has one row for each element of the SQL array
* designated by this object, with the first row containing the
* element at index index
. The result set has
* up to count
rows in ascending order based on the
* indices. Each row has two columns: The second column stores
* the element value; the first column stores the index into the
* array for that element.
*
* @param index the array index of the first element to retrieve;
* the first element is at index 1
* @param count the number of successive SQL array elements to retrieve
* @return a ResultSet
object containing up to
* count
consecutive elements of the SQL array
* designated by this Array
object, starting at
* index index
.
* @throws SQLException if an error occurs while attempting to
* access the array
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public ResultSet getResultSet(long index, int count) throws SQLException
{
throw CBDriver.notImplemented(CBArray.class,"getResultSet");
}
/**
* Retrieves a result set holding the elements of the subarray that
* starts at index index
and contains up to
* count
successive elements.
* This method uses
* the specified map
for type map customizations
* unless the base type of the array does not match a user-defined
* type in map
, in which case it
* uses the standard mapping. This version of the method
* getResultSet
uses either the given type map or the standard mapping;
* it never uses the type map associated with the connection.
*
* The result set has one row for each element of the SQL array
* designated by this object, with the first row containing the
* element at index index
. The result set has
* up to count
rows in ascending order based on the
* indices. Each row has two columns: The second column stores
* the element value; the first column stores the index into the
* array for that element.
*
* @param index the array index of the first element to retrieve;
* the first element is at index 1
* @param count the number of successive SQL array elements to retrieve
* @param map the Map
object that contains the mapping
* of SQL type names to classes in the Java(tm) programming language
* @return a ResultSet
object containing up to
* count
consecutive elements of the SQL array
* designated by this Array
object, starting at
* index index
.
* @throws SQLException if an error occurs while attempting to
* access the array
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.2
*/
@Override
public ResultSet getResultSet(long index, int count, Map> map) throws SQLException
{
throw CBDriver.notImplemented(CBArray.class,"getResultSet");
}
/**
* This method frees the Array
object and releases the resources that
* it holds. The object is invalid once the free
* method is called.
*
* After free
has been called, any attempt to invoke a
* method other than free
will result in a SQLException
* being thrown. If free
is called multiple times, the subsequent
* calls to free
are treated as a no-op.
*
* @throws SQLException if an error occurs releasing
* the Array's resources
* @throws SQLFeatureNotSupportedException if the JDBC driver does not support
* this method
* @since 1.6
*/
@Override
public void free() throws SQLException
{
baseType=null;
array=null;
jsonArray=null;
}
String getJsonArray()
{
return jsonArray;
}
@Override
public boolean equals(Object o)
{
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CBArray cbArray = (CBArray) o;
// Probably incorrect - comparing Object[] arrays with Arrays.equals
return Arrays.equals(array, cbArray.array);
}
@Override
public int hashCode()
{
return Arrays.hashCode(array);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy