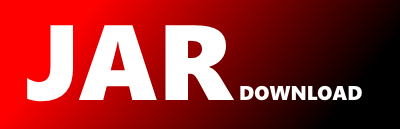
com.couchbase.jdbc.core.CouchResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdbc-cb Show documentation
Show all versions of jdbc-cb Show documentation
A JDBC driver for the Couchbase database, based on the N1QL query language.
The newest version!
/*
* // Copyright (c) 2015 Couchbase, Inc.
* // Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
* // except in compliance with the License. You may obtain a copy of the License at
* // http://www.apache.org/licenses/LICENSE-2.0
* // Unless required by applicable law or agreed to in writing, software distributed under the
* // License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* // either express or implied. See the License for the specific language governing permissions
* // and limitations under the License.
*/
package com.couchbase.jdbc.core;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.atomic.AtomicBoolean;
/**
* Created by davec on 2015-06-23.
*/
public class CouchResponse
{
AtomicBoolean fieldsInitialized = new AtomicBoolean(false);
Map signature=null;
ArrayList fields;
List errors;
List warnings;
CouchMetrics metrics;
String requestId;
String status;
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy