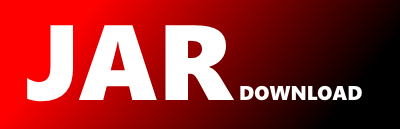
file.contract.FileManager Maven / Gradle / Ivy
package file.contract;
import java.io.File;
import java.io.Serializable;
/**
* This interface is responsible for defining the methods that allow
* manipulating files in a specific repository.
*
* @author Jhonathan Camacho
*
*/
public interface FileManager extends Serializable {
/**
* Inserts or updates a file in the directory.
*
* @param fileName
* is the file name.
* @param format
* is the file format.
* @param file
* is the file to be inserted or updated.
*
* @return true if the insert or update operation was successful and false
* otherwise.
*/
public boolean insert(String fileName, String format, byte[] file);
/**
* Remove a file in the directory.
*
* @param fileName
* is the file name.
*
* @return true if the removal was successful and false otherwise.
*/
public boolean remove(String fileName);
/**
* Returns, in byte format, the file that corresponds to the fileName
* parameter.
*
* @param fileName
* is the file name.
*
* @return if the file that have the given file name or null if there is no
* file with the given name.
*/
public byte[] fileToByte(String fileName);
/**
* Returns the file that corresponds to the fileName parameter.
*
* @param fileName
* is the file name.
*
* @return if the file that have the given file name or null if there is no
* file with the given name.
*/
public File getFile(String fileName);
/**
* Verify if the file exists in the directory.
*
* @param fileName is the
* file name.
*
* @return true if the file exists and false otherwise.
*/
public boolean fileExists(String fileName);
/**
* Returns the path of the repository.
*
* @return the repository path.
*/
public String getRepositoryPath();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy