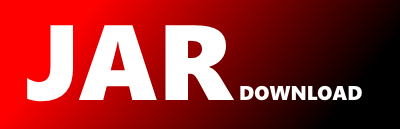
file.util.properties.PropertiesLoader Maven / Gradle / Ivy
package file.util.properties;
import java.io.IOException;
import java.nio.file.FileSystemNotFoundException;
import java.util.Properties;
import javax.enterprise.context.RequestScoped;
import file.util.verifier.StringVerifier;
/**
* This class allow to load properties from file properties.
*
* @author Jhonathan Camacho
*
*/
@RequestScoped
public class PropertiesLoader {
/***
* Verify if the property file exists in the project.
*
* @param propertiesName
* is the property file name.
* @return true if the properties file exists and false otherwise.
*/
public boolean hasPropertiesFile(String propertiesName) {
ClassLoader loader = Thread.currentThread().getContextClassLoader();
return loader.getResourceAsStream(propertiesName) != null;
}
/***
* Load a specific property file into memory, if it
* exists.
*
* @param propertiesName
* is the property file name.
* @return the properties file if exists and FileSystemNotFoundException if
* not.
*/
public Properties getPropertiesFile(String propertiesName) {
Properties properties = null;
try {
properties = new Properties();
ClassLoader loader = Thread.currentThread().getContextClassLoader();
properties.load(loader.getResourceAsStream(propertiesName));
} catch (IOException e) {
throw new FileSystemNotFoundException("Property file '" + propertiesName + "' not found in the classpath.");
}
return properties;
}
/***
* Searchs a specific property in a propertie file, if
* it exists.
*
* @param properties
* the properties file.
* @param propertieKey
* the name of the specific property in a properties file.
* @return the value of the specific property.
*/
public String getProperty(Properties properties, String propertieKey) {
verifyIfKeyExists(properties, propertieKey);
return properties.getProperty(propertieKey);
}
private boolean verifyIfKeyExists(Properties properties, String propertieKey) {
if (StringVerifier.isBlanck(propertieKey))
throw new IllegalArgumentException("The key '" + propertieKey + "' is blanck.");
if (!properties.containsKey(propertieKey))
throw new IllegalArgumentException("The key '" + propertieKey + "' doesn't exist.");
return true;
}
/***
* Verify if a specific property has a specific value.
*
* @param properties
* the properties file.
* @param propertieValue
* the value of the propertie.
* @return the true if value exists and IllegalArgumentException if not.
*/
public boolean verifyIfValueExists(Properties properties, String propertieValue) {
if (StringVerifier.isBlanck(getProperty(properties, propertieValue)))
throw new IllegalArgumentException("The value '" + propertieValue + "' doesn't exist.");
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy