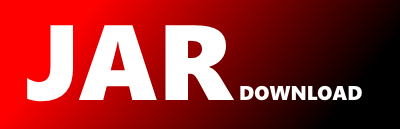
com.github.jhonyscamacho.exception.handler.JsfExceptionHandler Maven / Gradle / Ivy
package com.github.jhonyscamacho.exception.handler;
import java.io.IOException;
import java.util.Iterator;
import javax.faces.FacesException;
import javax.faces.application.ViewExpiredException;
import javax.faces.context.ExceptionHandler;
import javax.faces.context.ExceptionHandlerWrapper;
import javax.faces.context.ExternalContext;
import javax.faces.context.FacesContext;
import javax.faces.event.ExceptionQueuedEvent;
import javax.faces.event.ExceptionQueuedEventContext;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
public class JsfExceptionHandler extends ExceptionHandlerWrapper {
private static Log log = LogFactory.getLog(JsfExceptionHandler.class);
private ExceptionHandler wrapped;
public JsfExceptionHandler(ExceptionHandler wrapped) {
this.wrapped = wrapped;
}
@Override
public ExceptionHandler getWrapped() {
return this.wrapped;
}
@Override
public void handle() throws FacesException {
Iterator events = getUnhandledExceptionQueuedEvents().iterator();
while (events.hasNext()) {
ExceptionQueuedEvent event = events.next();
ExceptionQueuedEventContext context = (ExceptionQueuedEventContext) event.getSource();
Throwable exception = context.getException();
boolean handled = false;
try {
if (exception instanceof ViewExpiredException) {
handled = true;
redirect("/");
} else {
handled = true;
log.error("Erro de Sistema: " + exception.getMessage(), exception);
redirect("/404.html");
}
} finally {
if (handled) {
events.remove();
}
}
}
getWrapped().handle();
}
private void redirect(String page) {
try {
FacesContext facesContext = FacesContext.getCurrentInstance();
ExternalContext externalContext = facesContext.getExternalContext();
String contextPath = externalContext.getRequestContextPath();
externalContext.redirect(contextPath + page);
facesContext.responseComplete();
} catch (IOException e) {
throw new FacesException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy