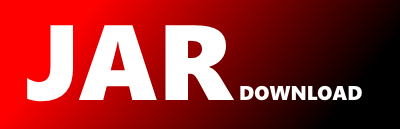
jiconfont.javafx.CatalogPane Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jiconfont-javafx Show documentation
Show all versions of jiconfont-javafx Show documentation
jIconFont-JavaFX is a API to provide icons generated from any IconFont. These icons can be used in
JavaFX.
package jiconfont.javafx;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.control.*;
import javafx.scene.input.MouseEvent;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import jiconfont.IconCode;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Copyright (c) 2016 jIconFont
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
public class CatalogPane extends BorderPane {
private Label iconUnderMouse;
private HBox configPane;
private ColorPicker iconColorPicker;
private ColorPicker backgroundColorPicker;
private Spinner spinner;
private CheckBox checkBox;
private Map icons = new HashMap<>();
public CatalogPane() {
setBackground(new Background(new BackgroundFill(Color.WHITE, null, null)));
BorderPane.setMargin(getConfigPane(), new Insets(5, 5, 5, 5));
BorderPane.setMargin(getIconUnderMouse(), new Insets(0, 5, 5, 5));
setTop(getConfigPane());
setBottom(getIconUnderMouse());
update();
}
public Label getIconUnderMouse() {
if (iconUnderMouse == null) {
iconUnderMouse = new Label();
}
return iconUnderMouse;
}
public ColorPicker getIconColorPicker() {
if (iconColorPicker == null) {
iconColorPicker = new ColorPicker();
iconColorPicker.setValue(Color.BLACK);
iconColorPicker.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
update();
}
});
}
return iconColorPicker;
}
public ColorPicker getBackgroundColorPicker() {
if (backgroundColorPicker == null) {
backgroundColorPicker = new ColorPicker();
backgroundColorPicker.setValue(Color.WHITE);
backgroundColorPicker.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
update();
}
});
}
return backgroundColorPicker;
}
public Spinner getSpinner() {
if (spinner == null) {
spinner = new Spinner<>();
spinner.setEditable(true);
SpinnerValueFactory svf =
new SpinnerValueFactory.IntegerSpinnerValueFactory(12, 48);
svf.setValue(new Integer(25));
spinner.setValueFactory(svf);
spinner.valueProperty().addListener(new ChangeListener() {
@Override
public void changed(ObservableValue extends Integer> observable,
Integer oldValue, Integer newValue) {
update();
}
});
}
return spinner;
}
public CheckBox getCheckBox() {
if (checkBox == null) {
checkBox = new CheckBox("Show icon name");
checkBox.setSelected(true);
checkBox.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
update();
}
});
}
return checkBox;
}
public HBox getConfigPane() {
if (configPane == null) {
configPane = new HBox();
configPane.setSpacing(3);
configPane.setAlignment(Pos.CENTER_LEFT);
configPane.getChildren().addAll(getCheckBox(), new Label("Icon size:"),
getSpinner(), new Label("Icon color:"), getIconColorPicker(),
new Label("Background:"), getBackgroundColorPicker());
HBox.setMargin(getCheckBox(), new Insets(0, 10, 0, 0));
HBox.setMargin(getSpinner(), new Insets(0, 10, 0, 0));
HBox.setMargin(getIconColorPicker(), new Insets(0, 10, 0, 0));
}
return configPane;
}
public final void update() {
VBox vBox = new VBox();
vBox.setFillWidth(true);
vBox.setBackground(new Background(
new BackgroundFill(getBackgroundColorPicker().getValue(), null, null)));
for (IconCollection iconCollection : getIcons().values()) {
Label fontFamilyTitle = new Label(iconCollection.getName());
fontFamilyTitle.setTextFill(getIconColorPicker().getValue());
fontFamilyTitle.setStyle("-fx-font-size: 22px;");
VBox.setMargin(fontFamilyTitle, new Insets(10, 0, 10, 20));
vBox.getChildren().addAll(fontFamilyTitle);
TilePane pane = new TilePane();
pane.setBorder(new Border(new BorderStroke(Color.TRANSPARENT,
BorderStrokeStyle.SOLID, null, new BorderWidths(5, 5, 5, 5))));
pane.setHgap(5);
pane.setVgap(10);
for (final IconCode icon : iconCollection.getIcons()) {
IconBuilderFX iconBuilderFX =
IconBuilderFX.newIcon(icon).setSize(getSpinner().getValue())
.setColor(getIconColorPicker().getValue());
Label label = null;
if (getCheckBox().isSelected()) {
label = new Label(icon.name());
label.setTextFill(getIconColorPicker().getValue());
label.setStyle("-fx-font-size: 9px;");
label.setContentDisplay(ContentDisplay.TOP);
iconBuilderFX.setGraphic(label);
} else {
label = iconBuilderFX.buildLabel();
}
label.setOnMouseEntered(new EventHandler() {
@Override
public void handle(MouseEvent event) {
getIconUnderMouse().setText("Icon: " + icon.name());
}
});
label.setOnMouseExited(new EventHandler() {
@Override
public void handle(MouseEvent event) {
getIconUnderMouse().setText("");
}
});
pane.getChildren().add(label);
}
vBox.getChildren().addAll(pane);
}
ScrollPane sp = new ScrollPane(vBox);
sp.setVbarPolicy(ScrollPane.ScrollBarPolicy.ALWAYS);
sp.setFitToWidth(true);
sp.setFitToHeight(true);
BorderPane.setMargin(sp, new Insets(5, 5, 5, 5));
setCenter(sp);
}
public void register(IconCode icon) {
IconCollection iconCollection = icons.get(icon.getFontFamily());
if (iconCollection == null) {
iconCollection = new IconCollection(icon.getClass().getSimpleName());
icons.put(icon.getFontFamily(), iconCollection);
}
iconCollection.add(icon);
}
public Map getIcons() {
return icons;
}
private static class IconCollection {
private String name;
private List icons;
public IconCollection(String name) {
this.name = name;
this.icons = new ArrayList<>();
}
public void add(IconCode icon) {
this.icons.add(icon);
}
public List getIcons() {
return icons;
}
public String getName() {
return name;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy