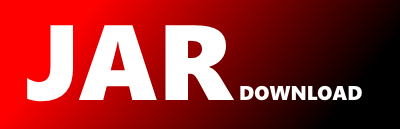
com.github.jknack.handlebars.internal.HbsLexer Maven / Gradle / Ivy
// Generated from com/github/jknack/handlebars/internal/HbsLexer.g4 by ANTLR 4.0
package com.github.jknack.handlebars.internal;
import org.antlr.v4.runtime.Lexer;
import org.antlr.v4.runtime.CharStream;
import org.antlr.v4.runtime.Token;
import org.antlr.v4.runtime.TokenStream;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.misc.*;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class HbsLexer extends Lexer {
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
TEXT=1, START_COMMENT=2, START_AMP=3, START_T=4, UNLESS=5, START_BLOCK=6,
START_DELIM=7, START_PARTIAL=8, END_BLOCK=9, START=10, SPACE=11, NL=12,
END_DELIM=13, WS_DELIM=14, DELIM=15, PATH=16, WS_PATH=17, END_T=18, END=19,
DOUBLE_STRING=20, SINGLE_STRING=21, EQ=22, INT=23, BOOLEAN=24, ELSE=25,
QID=26, WS=27, END_COMMENT=28, COMMENT_CHAR=29;
public static final int SET_DELIMS = 1;
public static final int PARTIAL = 2;
public static final int VAR = 3;
public static final int COMMENTS = 4;
public static String[] modeNames = {
"DEFAULT_MODE", "SET_DELIMS", "PARTIAL", "VAR", "COMMENTS"
};
public static final String[] tokenNames = {
"",
"TEXT", "START_COMMENT", "START_AMP", "START_T", "UNLESS", "START_BLOCK",
"START_DELIM", "START_PARTIAL", "END_BLOCK", "START", "SPACE", "NL", "END_DELIM",
"WS_DELIM", "DELIM", "PATH", "WS_PATH", "END_T", "END", "DOUBLE_STRING",
"SINGLE_STRING", "'='", "INT", "BOOLEAN", "'else'", "QID", "WS", "END_COMMENT",
"COMMENT_CHAR"
};
public static final String[] ruleNames = {
"TEXT", "START_COMMENT", "START_AMP", "START_T", "UNLESS", "START_BLOCK",
"START_DELIM", "START_PARTIAL", "END_BLOCK", "START", "SPACE", "NL", "END_DELIM",
"WS_DELIM", "DELIM", "PATH", "PATH_SEGMENT", "WS_PATH", "END_T", "END",
"DOUBLE_STRING", "SINGLE_STRING", "EQ", "INT", "BOOLEAN", "ELSE", "QID",
"ID_SEPARATOR", "ID", "ID_START", "ID_SUFFIX", "ID_ESCAPE", "ID_PART",
"WS", "END_COMMENT", "COMMENT_CHAR"
};
// Some default values
String start = "{{";
String end = "}}";
public HbsLexer(CharStream input, String start, String end) {
this(input);
this.start = start;
this.end = end;
}
private boolean consumeUntil(final String token) {
int offset = 0;
while(!isEOF(offset) && !ahead(token, offset) &&
!Character.isWhitespace(_input.LA(offset + 1))) {
offset+=1;
}
if (offset == 0) {
return false;
}
// Since we found the text, increase the CharStream's index.
_input.seek(_input.index() + offset - 1);
getInterpreter().setCharPositionInLine(_tokenStartCharPositionInLine + offset - 1);
return true;
}
private boolean tryToken(final String text) {
if (ahead(text)) {
// Since we found the text, increase the CharStream's index.
_input.seek(_input.index() + text.length() - 1);
getInterpreter().setCharPositionInLine(_tokenStartCharPositionInLine + text.length() - 1);
return true;
}
return false;
}
private boolean isEOF(final int offset) {
return _input.LA(offset + 1) == EOF;
}
private boolean ahead(final String text) {
return ahead(text, 0);
}
private boolean ahead(final String text, int offset) {
// See if `text` is ahead in the CharStream.
for (int i = 0; i < text.length(); i++) {
int ch = _input.LA(i + offset + 1);
if (ch != text.charAt(i)) {
// Nope, we didn't find `text`.
return false;
}
}
return true;
}
public HbsLexer(CharStream input) {
super(input);
_interp = new LexerATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
@Override
public String getGrammarFileName() { return "HbsLexer.g4"; }
@Override
public String[] getTokenNames() { return tokenNames; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String[] getModeNames() { return modeNames; }
@Override
public ATN getATN() { return _ATN; }
@Override
public void action(RuleContext _localctx, int ruleIndex, int actionIndex) {
switch (ruleIndex) {
case 0: TEXT_action((RuleContext)_localctx, actionIndex); break;
case 1: START_COMMENT_action((RuleContext)_localctx, actionIndex); break;
case 2: START_AMP_action((RuleContext)_localctx, actionIndex); break;
case 3: START_T_action((RuleContext)_localctx, actionIndex); break;
case 4: UNLESS_action((RuleContext)_localctx, actionIndex); break;
case 5: START_BLOCK_action((RuleContext)_localctx, actionIndex); break;
case 6: START_DELIM_action((RuleContext)_localctx, actionIndex); break;
case 7: START_PARTIAL_action((RuleContext)_localctx, actionIndex); break;
case 8: END_BLOCK_action((RuleContext)_localctx, actionIndex); break;
case 9: START_action((RuleContext)_localctx, actionIndex); break;
case 12: END_DELIM_action((RuleContext)_localctx, actionIndex); break;
case 15: PATH_action((RuleContext)_localctx, actionIndex); break;
case 17: WS_PATH_action((RuleContext)_localctx, actionIndex); break;
case 18: END_T_action((RuleContext)_localctx, actionIndex); break;
case 19: END_action((RuleContext)_localctx, actionIndex); break;
case 33: WS_action((RuleContext)_localctx, actionIndex); break;
case 34: END_COMMENT_action((RuleContext)_localctx, actionIndex); break;
}
}
private void WS_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 14: skip(); break;
}
}
private void END_BLOCK_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 7: pushMode(VAR); break;
}
}
private void START_T_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 2: pushMode(VAR); break;
}
}
private void END_COMMENT_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 15: popMode(); break;
}
}
private void START_BLOCK_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 4: pushMode(VAR); break;
}
}
private void PATH_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 10: _mode = VAR; break;
}
}
private void START_DELIM_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 5: pushMode(SET_DELIMS); break;
}
}
private void END_DELIM_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 9: popMode(); break;
}
}
private void START_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 8: pushMode(VAR); break;
}
}
private void WS_PATH_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 11: skip(); break;
}
}
private void START_PARTIAL_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 6: pushMode(PARTIAL); break;
}
}
private void TEXT_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
}
}
private void END_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 13: _mode = DEFAULT_MODE; break;
}
}
private void END_T_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 12: popMode(); break;
}
}
private void UNLESS_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 3: pushMode(VAR); break;
}
}
private void START_AMP_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 1: pushMode(VAR); break;
}
}
private void START_COMMENT_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 0: pushMode(COMMENTS); break;
}
}
@Override
public boolean sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
case 0: return TEXT_sempred((RuleContext)_localctx, predIndex);
case 1: return START_COMMENT_sempred((RuleContext)_localctx, predIndex);
case 2: return START_AMP_sempred((RuleContext)_localctx, predIndex);
case 3: return START_T_sempred((RuleContext)_localctx, predIndex);
case 4: return UNLESS_sempred((RuleContext)_localctx, predIndex);
case 5: return START_BLOCK_sempred((RuleContext)_localctx, predIndex);
case 6: return START_DELIM_sempred((RuleContext)_localctx, predIndex);
case 7: return START_PARTIAL_sempred((RuleContext)_localctx, predIndex);
case 8: return END_BLOCK_sempred((RuleContext)_localctx, predIndex);
case 9: return START_sempred((RuleContext)_localctx, predIndex);
case 12: return END_DELIM_sempred((RuleContext)_localctx, predIndex);
case 18: return END_T_sempred((RuleContext)_localctx, predIndex);
case 19: return END_sempred((RuleContext)_localctx, predIndex);
case 34: return END_COMMENT_sempred((RuleContext)_localctx, predIndex);
}
return true;
}
private boolean END_BLOCK_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 8: return tryToken(start + "/");
}
return true;
}
private boolean START_T_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 3: return tryToken(start + "{");
}
return true;
}
private boolean END_COMMENT_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 13: return tryToken(end);
}
return true;
}
private boolean START_BLOCK_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 5: return tryToken(start + "#");
}
return true;
}
private boolean START_DELIM_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 6: return tryToken(start + "=");
}
return true;
}
private boolean END_DELIM_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 10: return tryToken("=" + end);
}
return true;
}
private boolean START_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 9: return tryToken(start);
}
return true;
}
private boolean START_PARTIAL_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 7: return tryToken(start + ">");
}
return true;
}
private boolean TEXT_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 0: return consumeUntil(start);
}
return true;
}
private boolean END_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 12: return tryToken(end);
}
return true;
}
private boolean UNLESS_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 4: return tryToken(start + "^");
}
return true;
}
private boolean END_T_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 11: return tryToken("}" + end);
}
return true;
}
private boolean START_AMP_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 2: return tryToken(start + "&");
}
return true;
}
private boolean START_COMMENT_sempred(RuleContext _localctx, int predIndex) {
switch (predIndex) {
case 1: return tryToken(start + "!");
}
return true;
}
public static final String _serializedATN =
"\2\4\37\u011a\b\1\b\1\b\1\b\1\b\1\4\2\t\2\4\3\t\3\4\4\t\4\4\5\t\5\4\6"+
"\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13\t\13\4\f\t\f\4\r\t\r\4\16\t"+
"\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22\4\23\t\23\4\24\t\24\4\25\t"+
"\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31\4\32\t\32\4\33\t\33\4\34\t"+
"\34\4\35\t\35\4\36\t\36\4\37\t\37\4 \t \4!\t!\4\"\t\"\4#\t#\4$\t$\4%\t"+
"%\3\2\3\2\3\2\3\3\3\3\3\3\3\3\3\3\3\4\3\4\3\4\3\4\3\4\3\5\3\5\3\5\3\5"+
"\3\5\3\6\3\6\3\6\3\6\3\6\3\7\3\7\3\7\3\7\3\7\3\b\3\b\3\b\3\b\3\b\3\t\3"+
"\t\3\t\3\t\3\t\3\n\3\n\3\n\3\n\3\n\3\13\3\13\3\13\3\13\3\13\3\f\6\f\u0081"+
"\n\f\r\f\16\f\u0082\3\r\5\r\u0086\n\r\3\r\3\r\5\r\u008a\n\r\3\16\3\16"+
"\3\16\3\16\3\16\3\17\3\17\3\20\3\20\3\21\3\21\3\21\3\21\3\21\5\21\u009a"+
"\n\21\3\21\3\21\3\22\6\22\u009f\n\22\r\22\16\22\u00a0\3\23\3\23\3\23\3"+
"\23\3\24\3\24\3\24\3\24\3\24\3\25\3\25\3\25\3\25\3\25\3\26\3\26\3\26\3"+
"\26\7\26\u00b5\n\26\f\26\16\26\u00b8\13\26\3\26\3\26\3\27\3\27\3\27\3"+
"\27\7\27\u00c0\n\27\f\27\16\27\u00c3\13\27\3\27\3\27\3\30\3\30\3\31\6"+
"\31\u00ca\n\31\r\31\16\31\u00cb\3\32\3\32\3\32\3\32\3\32\3\32\3\32\3\32"+
"\3\32\5\32\u00d7\n\32\3\33\3\33\3\33\3\33\3\33\3\34\3\34\3\34\3\34\3\34"+
"\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34"+
"\3\34\3\34\5\34\u00f3\n\34\3\35\3\35\3\36\3\36\7\36\u00f9\n\36\f\36\16"+
"\36\u00fc\13\36\3\37\3\37\3 \3 \3 \5 \u0103\n \3!\3!\3!\6!\u0108\n!\r"+
"!\16!\u0109\3!\3!\3\"\3\"\3#\3#\3#\3#\3$\3$\3$\3$\3$\3%\3%\5\u00b6\u00c1"+
"\u0109&\7\3\1\t\4\2\13\5\3\r\6\4\17\7\5\21\b\6\23\t\7\25\n\b\27\13\t\31"+
"\f\n\33\r\1\35\16\1\37\17\13!\20\1#\21\1%\22\f\'\2\1)\23\r+\24\16-\25"+
"\17/\26\1\61\27\1\63\30\1\65\31\1\67\32\19\33\1;\34\1=\2\1?\2\1A\2\1C"+
"\2\1E\2\1G\2\1I\35\20K\36\21M\37\1\7\2\3\4\5\6\f\4\13\13\"\"\5\13\f\17"+
"\17\"\"\b&&))/\3\2"+
"\2\2\u00f6\u00fa\5A\37\2\u00f7\u00f9\5C \2\u00f8\u00f7\3\2\2\2\u00f9\u00fc"+
"\3\2\2\2\u00fa\u00f8\3\2\2\2\u00fa\u00fb\3\2\2\2\u00fb@\3\2\2\2\u00fc"+
"\u00fa\3\2\2\2\u00fd\u00fe\t\t\2\2\u00feB\3\2\2\2\u00ff\u0103\5E!\2\u0100"+
"\u0103\5A\37\2\u0101\u0103\5G\"\2\u0102\u00ff\3\2\2\2\u0102\u0100\3\2"+
"\2\2\u0102\u0101\3\2\2\2\u0103D\3\2\2\2\u0104\u0105\7\60\2\2\u0105\u0107"+
"\7]\2\2\u0106\u0108\13\2\2\2\u0107\u0106\3\2\2\2\u0108\u0109\3\2\2\2\u0109"+
"\u010a\3\2\2\2\u0109\u0107\3\2\2\2\u010a\u010b\3\2\2\2\u010b\u010c\7_"+
"\2\2\u010cF\3\2\2\2\u010d\u010e\t\n\2\2\u010eH\3\2\2\2\u010f\u0110\t\13"+
"\2\2\u0110\u0111\3\2\2\2\u0111\u0112\b#\20\2\u0112J\3\2\2\2\u0113\u0114"+
"\6$\17\2\u0114\u0115\13\2\2\2\u0115\u0116\3\2\2\2\u0116\u0117\b$\21\2"+
"\u0117L\3\2\2\2\u0118\u0119\13\2\2\2\u0119N\3\2\2\2\26\2\3\4\5\6\u0082"+
"\u0085\u0089\u0099\u00a0\u00b4\u00b6\u00bf\u00c1\u00cb\u00d6\u00f2\u00fa"+
"\u0102\u0109";
public static final ATN _ATN =
ATNSimulator.deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy