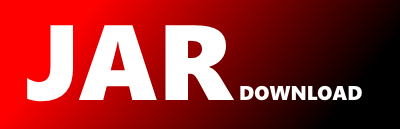
com.github.jlangch.venice.docgen.cheatsheet.snippets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of venice Show documentation
Show all versions of venice Show documentation
Venice, a Lisp implemented in Java
**Eval
import com.github.jlangch.venice.Venice;
public class Example {
public static void main(String[] args) {
final Venice venice = new Venice();
final Long result = (Long)venice.eval("(+ 1 2)");
}
}
**Passing parameters
import com.github.jlangch.venice.Parameters;
import com.github.jlangch.venice.Venice;
public class Example {
public static void main(String[] args) {
Venice venice = new Venice();
final Long result = (Long)venice.eval(
"(+ x y 3)",
Parameters.of("x", 6, "y", 3L));
}
}
**Dealing with Java objects
import java.awt.Point;
import com.github.jlangch.venice.Parameters;
import com.github.jlangch.venice.Venice;
public class Example {
public static void main(String[] args) {
Venice venice = new Venice();
// returns a string: "Point=(x: 100.0, y: 200.0)"
String ret = (String)venice.eval(
"(let [x (:x point) \n" +
" y (:y point)] \n" +
" (str \"Point=(x: \" x \", y: \" y \")\")) ",
Parameters.of("point", new Point(100, 200)));
// returns a java.awt.Point: [x=110,y=220]
Point point = (Point)venice.eval(
"(. :java.awt.Point :new (+ x 10) (+ y 20))",
Parameters.of("x", 100, "y", 200));
}
}
**Precompiling
import com.github.jlangch.venice.IPreCompiled;
import com.github.jlangch.venice.Parameters;
import com.github.jlangch.venice.Venice;
public class Example {
public static void main(String[] args) {
Venice venice = new Venice();
IPreCompiled precompiled = venice.precompile("example", "(+ 1 x)");
for(int ii=0; ii<100; ii++) {
venice.eval(precompiled, Parameters.of("x", ii));
}
}
}
**Java Interop
import java.time.ZonedDateTime;
import com.github.jlangch.venice.Venice;
public class Example {
public static void main(String[] args) {
Venice venice = new Venice();
Long val = (Long)venice.eval("(. :java.lang.Math :min 20 30)");
ZonedDateTime ts = (ZonedDateTime)venice.eval(
"(. (. :java.time.ZonedDateTime :now) :plusDays 5)");
}
}
**Sandbox
import com.github.jlangch.venice.SecurityException;
import com.github.jlangch.venice.Venice;
import com.github.jlangch.venice.javainterop.SandboxInterceptor;
import com.github.jlangch.venice.javainterop.SandboxRules;
public class SandboxExample {
public static void main(final String[] args) {
final SandboxInterceptor sandbox =
new SandboxRules()
// Venice functions: blacklist all unsafe functions
.rejectAllUnsafeFunctions()
// Venice functions: whitelist rules for print functions to offset
// blacklist rules by individual functions
.whitelistVeniceFunctions("*print*")
.sandbox();
final Venice venice = new Venice(sandbox);
// => OK, 'println' is part of the unsafe functions, but enabled by the 2nd rule
venice.eval("(println 100)");
// => FAIL, 'read-line' is part of the unsafe functions
try {
venice.eval("(read-line)");
}
catch(SecurityException ex) {
System.out.println("REJECTED: (read-line)");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy