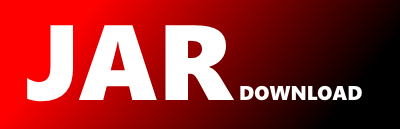
com.github.jmchilton.blend4j.BaseClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blend4j Show documentation
Show all versions of blend4j Show documentation
blend4j is a JVM partial reimplemenation of the Python library bioblend (http://bioblend.readthedocs.org/en/latest/) for interacting with Galaxy, CloudMan, and BioCloudCentral.
package com.github.jmchilton.blend4j;
import com.github.jmchilton.blend4j.exceptions.ResponseException;
import com.github.jmchilton.blend4j.exceptions.SerializationException;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.multipart.BodyPart;
import com.sun.jersey.multipart.FormDataBodyPart;
import com.sun.jersey.multipart.FormDataMultiPart;
import com.sun.jersey.multipart.file.FileDataBodyPart;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response.Status.Family;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.type.TypeReference;
public class BaseClient {
protected final ObjectMapper mapper = new ObjectMapper();
protected final WebResource webResource;
public BaseClient(final WebResource baseWebResource,
final String module) {
this.webResource = baseWebResource.path(module);
}
protected ClientResponse create(final Object object) {
return create(object, true);
}
protected ClientResponse create(final Object object, final boolean checkResponse) {
final ClientResponse response = create(getWebResource(), object, checkResponse);
return response;
}
protected ClientResponse create(final WebResource webResource, final Object object) {
return create(webResource, object, true);
}
protected ClientResponse create(final WebResource webResource, final Object object, final boolean checkResponse) {
final ClientResponse response = webResource.type(MediaType.APPLICATION_JSON).accept(MediaType.APPLICATION_JSON).post(ClientResponse.class, object);
if(checkResponse) {
this.checkResponse(response);
}
return response;
}
protected List get(final WebResource webResource, final TypeReference> typeReference) {
final String json = getJson(webResource);
return readJson(json, typeReference);
}
protected List get(final TypeReference> typeReference) {
return get(getWebResource(), typeReference);
}
protected ClientResponse getResponse(final WebResource webResource) {
return getResponse(webResource, true);
}
protected ClientResponse getResponse(final WebResource webResource, final boolean checkResponse) {
final ClientResponse response = webResource.accept(MediaType.APPLICATION_JSON).get(ClientResponse.class);
if(checkResponse) {
this.checkResponse(response);
}
return response;
}
protected String getJson(final WebResource webResource) {
return getJson(webResource, true);
}
protected String getJson(final WebResource webResource, final boolean checkResponse) {
final ClientResponse response = getResponse(webResource, checkResponse);
final String json = response.getEntity(String.class);
return json;
}
protected void checkResponse(final ClientResponse response) {
final Family family = response.getClientResponseStatus().getFamily();
if(family != Family.SUCCESSFUL) {
final ResponseException exception = buildResponseException(response);
throw exception;
}
}
protected ResponseException buildResponseException(final ClientResponse clientResponse) {
final ResponseException exception = new ResponseException(clientResponse);
return exception;
}
protected WebResource getWebResource() {
return webResource;
}
protected WebResource getWebResource(final String id) {
return path(id);
}
protected WebResource getWebResourceContents(final String id) {
return getWebResource(id).path("contents");
}
protected WebResource path(final String path) {
return getWebResource().path(path);
}
protected TypeReference> listTypeReference(final Class clazz) {
return new TypeReference>() {
};
}
protected ClientResponse multipartPost(final WebResource resource, final Map fields, final Iterable bodyParts) {
final WebResource.Builder builder = resource.type(MediaType.MULTIPART_FORM_DATA_TYPE);
final FormDataMultiPart multiPart = new FormDataMultiPart();
for(final Map.Entry fieldEntry : fields.entrySet()) {
final FormDataBodyPart bp = new FormDataBodyPart(fieldEntry.getKey(), fieldEntry.getValue(), MediaType.APPLICATION_JSON_TYPE);
multiPart.bodyPart(bp);
}
for(final BodyPart bodyPart : bodyParts) {
bodyPart.setMediaType(MediaType.APPLICATION_OCTET_STREAM_TYPE);
multiPart.bodyPart(bodyPart);
}
return builder.post(ClientResponse.class, multiPart);
}
protected Iterable prepareUpload(final File file) {
return prepareUploads(Arrays.asList(file));
}
protected Iterable prepareUploads(final Iterable files) {
final List bodyParts = new ArrayList();
for(final File file : files) {
final String paramName = "files_0|file_data";
final FileDataBodyPart fdbp = new FileDataBodyPart(paramName, file);
bodyParts.add(fdbp);
}
return bodyParts;
}
protected T readJson(final String json, final TypeReference typeReference) {
try {
return mapper.readValue(json, typeReference);
} catch(IOException e) {
throw new SerializationException(e);
}
}
protected T readJson(final String json, final Class clazz) {
try {
return mapper.readValue(json, clazz);
} catch(IOException e) {
throw new SerializationException(e);
}
}
protected T read(final ClientResponse response, final TypeReference typeReference) {
final String json = response.getEntity(String.class);
return readJson(json, typeReference);
}
protected T read(final ClientResponse response, final Class clazz) {
final String json = response.getEntity(String.class);
return readJson(json, clazz);
}
protected T show(final String id, Class clazz) {
return getWebResource(id).get(clazz);
}
protected String write(final Object object) {
try {
return mapper.writer().writeValueAsString(object);
} catch(IOException e) {
throw new SerializationException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy