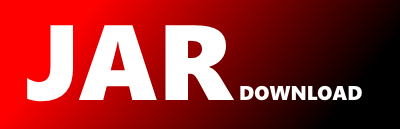
com.github.jmchilton.blend4j.galaxy.ToolShedRepositoriesClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blend4j Show documentation
Show all versions of blend4j Show documentation
blend4j is a JVM partial reimplemenation of the Python library bioblend (http://bioblend.readthedocs.org/en/latest/) for interacting with Galaxy, CloudMan, and BioCloudCentral.
package com.github.jmchilton.blend4j.galaxy;
import com.github.jmchilton.blend4j.galaxy.beans.InstallableRepositoryRevision;
import com.github.jmchilton.blend4j.galaxy.beans.InstalledRepository;
import com.github.jmchilton.blend4j.galaxy.beans.RepositoryInstall;
import com.github.jmchilton.blend4j.galaxy.beans.RepositoryWorkflow;
import com.github.jmchilton.blend4j.galaxy.beans.Workflow;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
import java.util.HashMap;
import java.util.List;
import org.codehaus.jackson.type.TypeReference;
class ToolShedRepositoriesClientImpl extends Client implements ToolShedRepositoriesClient {
private static final TypeReference> TOOL_SHED_REPOSITORY_LIST_TYPE_REFERENCE = new TypeReference>() {
};
private static final TypeReference> TOOL_SHED_REPOSITORY_WORKFLOW_LIST_TYPE_REFERENCE =
new TypeReference>() {
};
private static final TypeReference> WORKFLOW_LIST_TYPE_REFERENCE =
new TypeReference>() {
};
ToolShedRepositoriesClientImpl(GalaxyInstanceImpl galaxyInstance) {
super(galaxyInstance, "tool_shed_repositories");
}
public List getRepositories() {
return super.get(TOOL_SHED_REPOSITORY_LIST_TYPE_REFERENCE);
}
public InstalledRepository showRepository(final String toolShedId) {
return super.show(toolShedId, InstalledRepository.class);
}
public ClientResponse installRepositoryRequest(final RepositoryInstall install) {
final WebResource resource = super.webResource.path("new").path("install_repository_revision");
return super.create(resource, install);
}
public List installRepository(final RepositoryInstall install) {
final ClientResponse response = this.installRepositoryRequest(install);
return super.read(response, TOOL_SHED_REPOSITORY_LIST_TYPE_REFERENCE);
}
public ClientResponse repairRepositoryRequest(final InstallableRepositoryRevision repositoryIdentifier) {
final WebResource resource = super.webResource.path("repair_repository_revision");
return super.create(resource, repositoryIdentifier);
}
public ClientResponse exportedWorkflowsRequest(String toolShedId) {
final WebResource resource = super.webResource.path(toolShedId).path("exported_workflows");
return resource.get(ClientResponse.class);
}
public List exportedWorkflows(String toolShedId) {
return super.read(this.exportedWorkflowsRequest(toolShedId),
TOOL_SHED_REPOSITORY_WORKFLOW_LIST_TYPE_REFERENCE);
}
public ClientResponse importWorkflowRequest(String toolShedId, int index) {
final WebResource resource = super.webResource.path(toolShedId).path("import_workflow");
final HashMap postObject = new HashMap();
postObject.put("index", index);
return super.create(resource, postObject);
}
public Workflow importWorkflow(String toolShedId, int index) {
final ClientResponse response = this.importWorkflowRequest(toolShedId, index);
return response.getEntity(Workflow.class);
}
public ClientResponse importWorkflowsRequest(String toolShedId) {
final WebResource resource = super.webResource.path(toolShedId).path("import_workflows");
return super.create(resource);
}
public List importWorkflows(String toolShedId) {
return read(importWorkflowsRequest(toolShedId), WORKFLOW_LIST_TYPE_REFERENCE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy