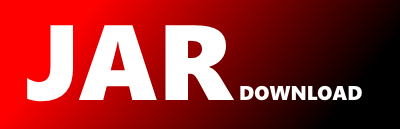
coo.base.util.BeanUtils Maven / Gradle / Ivy
The newest version!
package coo.base.util;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import coo.base.constants.Chars;
import coo.base.exception.UncheckedException;
/**
* Bean工具类。用于直接操作类、对象的属性或方法。
*/
public abstract class BeanUtils {
/**
* 获取类中指定名称的属性,支持多层级。
*
* @param targetClass
* 类
* @param fieldName
* 属性名
* @return 返回对应的属性,如果没找到返回null。
*/
public static Field findField(Class> targetClass, String fieldName) {
Assert.notNull(targetClass);
Assert.notBlank(fieldName);
if (fieldName.contains(".")) {
return findNestedField(targetClass, fieldName);
} else {
return findDirectField(targetClass, fieldName);
}
}
/**
* 获取类中注有指定标注的属性集合。
*
* @param targetClass
* 类
* @param annotationClassOnField
* 标注
* @return 返回注有指定标注的属性集合。
*/
public static List findField(Class> targetClass,
Class extends Annotation> annotationClassOnField) {
Assert.notNull(targetClass);
Assert.notNull(annotationClassOnField);
List fields = new ArrayList();
for (Field field : getAllDeclaredField(targetClass)) {
if (field.isAnnotationPresent(annotationClassOnField)) {
fields.add(field);
}
}
return fields;
}
/**
* 获取对象中指定属性的值。
*
* @param target
* 对象
* @param field
* 属性
* @return 返回对象中指定属性的值。
*/
public static Object getField(Object target, Field field) {
if (field == null) {
return null;
}
try {
boolean accessible = field.isAccessible();
field.setAccessible(true);
Object result = field.get(target);
field.setAccessible(accessible);
return processHibernateLazyField(result);
} catch (Exception e) {
throw new UncheckedException("获取对象的属性[" + field.getName() + "]值失败",
e);
}
}
/**
* 获取对象中指定属性的值,支持多层级。
*
* @param target
* 对象
* @param fieldName
* 属性名
* @return 返回对象中指定属性的值。
*/
public static Object getField(Object target, String fieldName) {
Assert.notNull(target);
Assert.notBlank(fieldName);
if (fieldName.contains(".")) {
return getNestedField(target, fieldName);
} else {
return getDirectField(target, fieldName);
}
}
/**
* 设置对象中指定属性的值。
*
* @param target
* 对象
* @param field
* 属性
* @param value
* 值
*/
public static void setField(Object target, Field field, Object value) {
try {
boolean accessible = field.isAccessible();
field.setAccessible(true);
field.set(target, value);
field.setAccessible(accessible);
} catch (Exception e) {
throw new IllegalStateException("设置对象的属性[" + field.getName()
+ "]值失败", e);
}
}
/**
* 设置对象中指定属性的值,支持多层级。
*
* @param target
* 对象
* @param fieldName
* 属性名
* @param value
* 值
*/
public static void setField(Object target, String fieldName, Object value) {
if (fieldName.contains(".")) {
setNestedField(target, fieldName, value);
} else {
setDirectField(target, fieldName, value);
}
}
/**
* 递归获取类的Field,直到其父类为Object类。子类的Field排列在父类之前。
*
* @param targetClass
* 类
* @param excludeFieldNames
* 排除的属性名称
* @return 返回Field列表。
*/
public static List getAllDeclaredField(Class> targetClass,
String... excludeFieldNames) {
List fields = new ArrayList();
for (Field field : targetClass.getDeclaredFields()) {
if (CollectionUtils.contains(excludeFieldNames, field.getName())) {
continue;
}
fields.add(field);
}
Class> parentClass = targetClass.getSuperclass();
if (parentClass != Object.class) {
fields.addAll(getAllDeclaredField(parentClass, excludeFieldNames));
}
return fields;
}
/**
* 复制两个对象相同Field的值,忽略源对象中为null的Field。
*
* @param source
* 源对象
* @param target
* 目标对象
*/
public static void copyFields(Object source, Object target) {
copyFields(source, target, null, null);
}
/**
* 复制两个对象相同Field的值,忽略源对象中为null的Field。
*
* @param source
* 源对象
* @param target
* 目标对象
* @param excludeFields
* 不复制的Field的名称,多个名称之间用“,”分割
*/
public static void copyFields(Object source, Object target,
String excludeFields) {
copyFields(source, target, null, excludeFields);
}
/**
* 复制两个对象相同Field的值,忽略源对象中为null的Field,但如果指定了要复制的Field,则为null时该Field也复制。
*
* @param source
* 源对象
* @param target
* 目标对象
* @param includeFields
* 要复制的Field的名称,多个名称之间用“,”分割
* @param excludeFields
* 不复制的Field的名称,多个名称之间用“,”分割
*/
public static void copyFields(Object source, Object target,
String includeFields, String excludeFields) {
String[] includeFieldNames = new String[] {};
if (!StringUtils.isBlank(includeFields)) {
includeFieldNames = includeFields.split(Chars.COMMA);
}
String[] excludeFieldNames = new String[] {};
if (!StringUtils.isBlank(excludeFields)) {
excludeFieldNames = excludeFields.split(Chars.COMMA);
}
for (Field field : getAllDeclaredField(source.getClass(),
excludeFieldNames)) {
if (CollectionUtils.contains(includeFieldNames, field.getName())) {
copyField(source, target, field.getName(), true);
} else {
copyField(source, target, field.getName(), false);
}
}
}
/**
* 复制两个对象指定Field的值。
*
* @param source
* 源对象
* @param target
* 目标对象
* @param fieldName
* Field的名称
* @param containedNull
* 是否复制null值
*/
@SuppressWarnings("unchecked")
public static void copyField(Object source, Object target,
String fieldName, Boolean containedNull) {
Object sourceFieldValue = getField(source, fieldName);
Boolean needCopy = isFieldNeedCopy(source, target, fieldName);
if (sourceFieldValue == null && !containedNull) {
needCopy = false;
}
if (needCopy) {
// 处理Collection类型的属性
if (sourceFieldValue != null
&& Collection.class.isAssignableFrom(sourceFieldValue
.getClass())) {
if (!((Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy