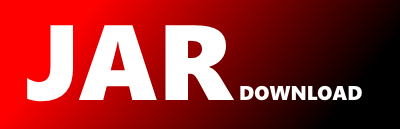
org.libsodium.jni.Sodium Maven / Gradle / Ivy
The newest version!
/* ----------------------------------------------------------------------------
* This file was automatically generated by SWIG (http://www.swig.org).
* Version 3.0.12
*
* Do not make changes to this file unless you know what you are doing--modify
* the SWIG interface file instead.
* ----------------------------------------------------------------------------- */
package org.libsodium.jni;
public class Sodium {
public static int sodium_init() {
return SodiumJNI.sodium_init();
}
public static byte[] sodium_version_string() {
return SodiumJNI.sodium_version_string();
}
public static void randombytes(byte[] dst_buf, int buf_len) {
SodiumJNI.randombytes(dst_buf, buf_len);
}
public static int randombytes_random() {
return SodiumJNI.randombytes_random();
}
public static int randombytes_uniform(int upper_bound) {
return SodiumJNI.randombytes_uniform(upper_bound);
}
public static void randombytes_buf(byte[] buff, int buff_len) {
SodiumJNI.randombytes_buf(buff, buff_len);
}
public static int randombytes_close() {
return SodiumJNI.randombytes_close();
}
public static void randombytes_stir() {
SodiumJNI.randombytes_stir();
}
public static void sodium_increment(byte[] src_dst_number, int number_len) {
SodiumJNI.sodium_increment(src_dst_number, number_len);
}
public static int crypto_secretbox_keybytes() {
return SodiumJNI.crypto_secretbox_keybytes();
}
public static int crypto_secretbox_noncebytes() {
return SodiumJNI.crypto_secretbox_noncebytes();
}
public static int crypto_secretbox_macbytes() {
return SodiumJNI.crypto_secretbox_macbytes();
}
public static int crypto_secretbox_zerobytes() {
return SodiumJNI.crypto_secretbox_zerobytes();
}
public static int crypto_secretbox_boxzerobytes() {
return SodiumJNI.crypto_secretbox_boxzerobytes();
}
public static byte[] crypto_secretbox_primitive() {
return SodiumJNI.crypto_secretbox_primitive();
}
public static int crypto_secretbox_easy(byte[] dst_cipher, byte[] src_plain, int plain_len, byte[] nonce, byte[] secret_key) {
return SodiumJNI.crypto_secretbox_easy(dst_cipher, src_plain, plain_len, nonce, secret_key);
}
public static int crypto_secretbox_open_easy(byte[] dst_plain, byte[] src_cipher, int cipher_len, byte[] nonce, byte[] secret_key) {
return SodiumJNI.crypto_secretbox_open_easy(dst_plain, src_cipher, cipher_len, nonce, secret_key);
}
public static int crypto_secretbox_detached(byte[] dst_cipher, byte[] mac, byte[] src_plain, int plain_len, byte[] nonce, byte[] secretkey) {
return SodiumJNI.crypto_secretbox_detached(dst_cipher, mac, src_plain, plain_len, nonce, secretkey);
}
public static int crypto_secretbox_open_detached(byte[] dst_plain, byte[] src_cipher, byte[] mac, int cipher_len, byte[] nonce, byte[] secretkey) {
return SodiumJNI.crypto_secretbox_open_detached(dst_plain, src_cipher, mac, cipher_len, nonce, secretkey);
}
public static int crypto_scalarmult_bytes() {
return SodiumJNI.crypto_scalarmult_bytes();
}
public static int crypto_scalarmult_scalarbytes() {
return SodiumJNI.crypto_scalarmult_scalarbytes();
}
public static byte[] crypto_scalarmult_primitive() {
return SodiumJNI.crypto_scalarmult_primitive();
}
public static int crypto_scalarmult_base(byte[] q, byte[] n) {
return SodiumJNI.crypto_scalarmult_base(q, n);
}
public static int crypto_scalarmult(byte[] q, byte[] n, byte[] p) {
return SodiumJNI.crypto_scalarmult(q, n, p);
}
public static int crypto_box_seedbytes() {
return SodiumJNI.crypto_box_seedbytes();
}
public static int crypto_box_publickeybytes() {
return SodiumJNI.crypto_box_publickeybytes();
}
public static int crypto_box_secretkeybytes() {
return SodiumJNI.crypto_box_secretkeybytes();
}
public static int crypto_box_noncebytes() {
return SodiumJNI.crypto_box_noncebytes();
}
public static int crypto_box_macbytes() {
return SodiumJNI.crypto_box_macbytes();
}
public static byte[] crypto_box_primitive() {
return SodiumJNI.crypto_box_primitive();
}
public static int crypto_box_keypair(byte[] dst_public_Key, byte[] dst_private_key) {
return SodiumJNI.crypto_box_keypair(dst_public_Key, dst_private_key);
}
public static int crypto_box_seed_keypair(byte[] dst_public_key, byte[] dst_private_key, byte[] src_seed) {
return SodiumJNI.crypto_box_seed_keypair(dst_public_key, dst_private_key, src_seed);
}
public static int crypto_box_easy(byte[] dst_cipher, byte[] src_plain, int plain_len, byte[] nonce, byte[] remote_public_key, byte[] local_private_key) {
return SodiumJNI.crypto_box_easy(dst_cipher, src_plain, plain_len, nonce, remote_public_key, local_private_key);
}
public static int crypto_box_open_easy(byte[] dst_plain, byte[] src_cipher, int cipher_len, byte[] nonce, byte[] remote_public_key, byte[] local_private_key) {
return SodiumJNI.crypto_box_open_easy(dst_plain, src_cipher, cipher_len, nonce, remote_public_key, local_private_key);
}
public static int crypto_box_detached(byte[] dst_cipher, byte[] dst_mac, byte[] src_plain, int plain_len, byte[] nonces, byte[] remote_public_key, byte[] local_private_key) {
return SodiumJNI.crypto_box_detached(dst_cipher, dst_mac, src_plain, plain_len, nonces, remote_public_key, local_private_key);
}
public static int crypto_box_open_detached(byte[] dst_plain, byte[] src_cipher, byte[] src_mac, int cipher_len, byte[] nonce, byte[] remote_public_key, byte[] local_private_key) {
return SodiumJNI.crypto_box_open_detached(dst_plain, src_cipher, src_mac, cipher_len, nonce, remote_public_key, local_private_key);
}
public static int crypto_box_beforenmbytes() {
return SodiumJNI.crypto_box_beforenmbytes();
}
public static int crypto_box_beforenm(byte[] dst_shared_key, byte[] remote_public_key, byte[] local_private_key) {
return SodiumJNI.crypto_box_beforenm(dst_shared_key, remote_public_key, local_private_key);
}
public static int crypto_box_easy_afternm(byte[] dst_cipher, byte[] src_plain, int plain_len, byte[] nonce, byte[] shared_key) {
return SodiumJNI.crypto_box_easy_afternm(dst_cipher, src_plain, plain_len, nonce, shared_key);
}
public static int crypto_box_open_easy_afternm(byte[] dst_plain, byte[] src_cipher, int cipher_len, byte[] nonce, byte[] shared_key) {
return SodiumJNI.crypto_box_open_easy_afternm(dst_plain, src_cipher, cipher_len, nonce, shared_key);
}
public static int crypto_box_detached_afternm(byte[] dst_cipher, byte[] dst_mac, byte[] src_plain, int plain_len, byte[] nonce, byte[] shared_key) {
return SodiumJNI.crypto_box_detached_afternm(dst_cipher, dst_mac, src_plain, plain_len, nonce, shared_key);
}
public static int crypto_box_open_detached_afternm(byte[] dst_plain, byte[] src_cipher, byte[] src_mac, int cipher_len, byte[] nonce, byte[] shared_key) {
return SodiumJNI.crypto_box_open_detached_afternm(dst_plain, src_cipher, src_mac, cipher_len, nonce, shared_key);
}
public static int crypto_box_sealbytes() {
return SodiumJNI.crypto_box_sealbytes();
}
public static int crypto_box_seal(byte[] dst_cipher, byte[] src_plain, int plain_len, byte[] remote_public_key) {
return SodiumJNI.crypto_box_seal(dst_cipher, src_plain, plain_len, remote_public_key);
}
public static int crypto_box_seal_open(byte[] dst_plain, byte[] src_cipher, int cipher_len, byte[] local_public_key, byte[] local_private_key) {
return SodiumJNI.crypto_box_seal_open(dst_plain, src_cipher, cipher_len, local_public_key, local_private_key);
}
public static int crypto_box_zerobytes() {
return SodiumJNI.crypto_box_zerobytes();
}
public static int crypto_box_boxzerobytes() {
return SodiumJNI.crypto_box_boxzerobytes();
}
public static int crypto_box(byte[] dst_cipher, byte[] src_msg, int msg_len, byte[] src_nonce, byte[] src_pub, byte[] src_secret) {
return SodiumJNI.crypto_box(dst_cipher, src_msg, msg_len, src_nonce, src_pub, src_secret);
}
public static int crypto_box_open(byte[] dst_msg, byte[] src_cipher, int cipher_len, byte[] src_nonce, byte[] src_pub, byte[] src_secret) {
return SodiumJNI.crypto_box_open(dst_msg, src_cipher, cipher_len, src_nonce, src_pub, src_secret);
}
public static int crypto_box_afternm(byte[] dst_cipher, byte[] src_msg, int msg_len, byte[] src_nonce, byte[] src_key) {
return SodiumJNI.crypto_box_afternm(dst_cipher, src_msg, msg_len, src_nonce, src_key);
}
public static int crypto_box_open_afternm(byte[] dst_msg, byte[] src_cipher, int cipher_len, byte[] src_nonce, byte[] src_key) {
return SodiumJNI.crypto_box_open_afternm(dst_msg, src_cipher, cipher_len, src_nonce, src_key);
}
public static int crypto_sign_bytes() {
return SodiumJNI.crypto_sign_bytes();
}
public static int crypto_sign_seedbytes() {
return SodiumJNI.crypto_sign_seedbytes();
}
public static int crypto_sign_publickeybytes() {
return SodiumJNI.crypto_sign_publickeybytes();
}
public static int crypto_sign_secretkeybytes() {
return SodiumJNI.crypto_sign_secretkeybytes();
}
public static byte[] crypto_sign_primitive() {
return SodiumJNI.crypto_sign_primitive();
}
public static int crypto_sign_keypair(byte[] dst_public_Key, byte[] dst_private_key) {
return SodiumJNI.crypto_sign_keypair(dst_public_Key, dst_private_key);
}
public static int crypto_sign_seed_keypair(byte[] dst_public_Key, byte[] dst_private_key, byte[] src_seed) {
return SodiumJNI.crypto_sign_seed_keypair(dst_public_Key, dst_private_key, src_seed);
}
public static int crypto_sign(byte[] dst_signed_msg, int[] signed_msg_len, byte[] src_msg, int msg_len, byte[] local_private_key) {
return SodiumJNI.crypto_sign(dst_signed_msg, signed_msg_len, src_msg, msg_len, local_private_key);
}
public static int crypto_sign_open(byte[] dst_msg, int[] msg_len, byte[] src_signed_msg, int signed_msg_len, byte[] remote_public_key) {
return SodiumJNI.crypto_sign_open(dst_msg, msg_len, src_signed_msg, signed_msg_len, remote_public_key);
}
public static int crypto_sign_detached(byte[] dst_signature, int[] signature_len, byte[] src_msg, int msg_len, byte[] local_private_key) {
return SodiumJNI.crypto_sign_detached(dst_signature, signature_len, src_msg, msg_len, local_private_key);
}
public static int crypto_sign_verify_detached(byte[] src_signature, byte[] src_msg, int msg_len, byte[] remote_public_key) {
return SodiumJNI.crypto_sign_verify_detached(src_signature, src_msg, msg_len, remote_public_key);
}
public static int crypto_sign_ed25519_sk_to_seed(byte[] dst_seed, byte[] src_private_key) {
return SodiumJNI.crypto_sign_ed25519_sk_to_seed(dst_seed, src_private_key);
}
public static int crypto_sign_ed25519_sk_to_pk(byte[] dst_public_key, byte[] src_private_key) {
return SodiumJNI.crypto_sign_ed25519_sk_to_pk(dst_public_key, src_private_key);
}
public static int crypto_generichash_bytes() {
return SodiumJNI.crypto_generichash_bytes();
}
public static int crypto_generichash_bytes_min() {
return SodiumJNI.crypto_generichash_bytes_min();
}
public static int crypto_generichash_bytes_max() {
return SodiumJNI.crypto_generichash_bytes_max();
}
public static int crypto_generichash_keybytes() {
return SodiumJNI.crypto_generichash_keybytes();
}
public static int crypto_generichash_keybytes_min() {
return SodiumJNI.crypto_generichash_keybytes_min();
}
public static int crypto_generichash_keybytes_max() {
return SodiumJNI.crypto_generichash_keybytes_max();
}
public static byte[] crypto_generichash_primitive() {
return SodiumJNI.crypto_generichash_primitive();
}
public static int crypto_generichash(byte[] dst_hash, int dst_len, byte[] src_input, int input_len, byte[] src_key, int key_len) {
return SodiumJNI.crypto_generichash(dst_hash, dst_len, src_input, input_len, src_key, key_len);
}
public static int crypto_generichash_statebytes() {
return SodiumJNI.crypto_generichash_statebytes();
}
public static int crypto_generichash_init(byte[] state, byte[] src_key, int key_len, int out_len) {
return SodiumJNI.crypto_generichash_init(state, src_key, key_len, out_len);
}
public static int crypto_generichash_update(byte[] state, byte[] src_input, int input_len) {
return SodiumJNI.crypto_generichash_update(state, src_input, input_len);
}
public static int crypto_generichash_final(byte[] state, byte[] dst_out, int out_len) {
return SodiumJNI.crypto_generichash_final(state, dst_out, out_len);
}
public static int crypto_shorthash_bytes() {
return SodiumJNI.crypto_shorthash_bytes();
}
public static int crypto_shorthash_keybytes() {
return SodiumJNI.crypto_shorthash_keybytes();
}
public static byte[] crypto_shorthash_primitive() {
return SodiumJNI.crypto_shorthash_primitive();
}
public static int crypto_shorthash(byte[] dst_out, byte[] src_input, int input_len, byte[] src_key) {
return SodiumJNI.crypto_shorthash(dst_out, src_input, input_len, src_key);
}
public static int crypto_auth_bytes() {
return SodiumJNI.crypto_auth_bytes();
}
public static int crypto_auth_keybytes() {
return SodiumJNI.crypto_auth_keybytes();
}
public static byte[] crypto_auth_primitive() {
return SodiumJNI.crypto_auth_primitive();
}
public static int crypto_auth(byte[] dst_mac, byte[] src_input, int input_len, byte[] src_key) {
return SodiumJNI.crypto_auth(dst_mac, src_input, input_len, src_key);
}
public static int crypto_auth_verify(byte[] src_mac, byte[] src_input, int input_len, byte[] src_key) {
return SodiumJNI.crypto_auth_verify(src_mac, src_input, input_len, src_key);
}
public static int crypto_onetimeauth_bytes() {
return SodiumJNI.crypto_onetimeauth_bytes();
}
public static int crypto_onetimeauth_keybytes() {
return SodiumJNI.crypto_onetimeauth_keybytes();
}
public static byte[] crypto_onetimeauth_primitive() {
return SodiumJNI.crypto_onetimeauth_primitive();
}
public static int crypto_onetimeauth(byte[] dst_out, byte[] src_input, int input_len, byte[] src_key) {
return SodiumJNI.crypto_onetimeauth(dst_out, src_input, input_len, src_key);
}
public static int crypto_onetimeauth_verify(byte[] src_mac, byte[] src_input, int input_len, byte[] src_key) {
return SodiumJNI.crypto_onetimeauth_verify(src_mac, src_input, input_len, src_key);
}
public static int crypto_onetimeauth_statebytes() {
return SodiumJNI.crypto_onetimeauth_statebytes();
}
public static int crypto_onetimeauth_init(byte[] dst_state, byte[] src_key) {
return SodiumJNI.crypto_onetimeauth_init(dst_state, src_key);
}
public static int crypto_onetimeauth_update(byte[] dst_state, byte[] src_input, int input_len) {
return SodiumJNI.crypto_onetimeauth_update(dst_state, src_input, input_len);
}
public static int crypto_onetimeauth_final(byte[] final_state, byte[] dst_out) {
return SodiumJNI.crypto_onetimeauth_final(final_state, dst_out);
}
public static int crypto_kx_keypair(byte[] pk, byte[] sk) {
return SodiumJNI.crypto_kx_keypair(pk, sk);
}
public static int crypto_kx_seed_keypair(byte[] pk, byte[] sk, byte[] seed) {
return SodiumJNI.crypto_kx_seed_keypair(pk, sk, seed);
}
public static int crypto_kx_client_session_keys(byte[] rx, byte[] tx, byte[] client_pk, byte[] client_sk, byte[] server_pk) {
return SodiumJNI.crypto_kx_client_session_keys(rx, tx, client_pk, client_sk, server_pk);
}
public static int crypto_kx_server_session_keys(byte[] rx, byte[] tx, byte[] server_pk, byte[] server_sk, byte[] client_pk) {
return SodiumJNI.crypto_kx_server_session_keys(rx, tx, server_pk, server_sk, client_pk);
}
public static int crypto_kdf_bytes_min() {
return SodiumJNI.crypto_kdf_bytes_min();
}
public static int crypto_kdf_bytes_max() {
return SodiumJNI.crypto_kdf_bytes_max();
}
public static int crypto_kdf_keybytes() {
return SodiumJNI.crypto_kdf_keybytes();
}
public static int crypto_kdf_contextbytes() {
return SodiumJNI.crypto_kdf_contextbytes();
}
public static byte[] crypto_kdf_primitive() {
return SodiumJNI.crypto_kdf_primitive();
}
public static int crypto_kdf_derive_from_key(byte[] subkey, int subkey_len, int subkey_id, byte[] ctx, byte[] key) {
return SodiumJNI.crypto_kdf_derive_from_key(subkey, subkey_len, subkey_id, ctx, key);
}
public static void crypto_kdf_keygen(byte[] key) {
SodiumJNI.crypto_kdf_keygen(key);
}
public static int crypto_aead_chacha20poly1305_keybytes() {
return SodiumJNI.crypto_aead_chacha20poly1305_keybytes();
}
public static int crypto_aead_chacha20poly1305_nsecbytes() {
return SodiumJNI.crypto_aead_chacha20poly1305_nsecbytes();
}
public static int crypto_aead_chacha20poly1305_npubbytes() {
return SodiumJNI.crypto_aead_chacha20poly1305_npubbytes();
}
public static int crypto_aead_chacha20poly1305_abytes() {
return SodiumJNI.crypto_aead_chacha20poly1305_abytes();
}
public static int crypto_aead_chacha20poly1305_messagebytes_max() {
return SodiumJNI.crypto_aead_chacha20poly1305_messagebytes_max();
}
public static void crypto_aead_chacha20poly1305_keygen(byte[] k) {
SodiumJNI.crypto_aead_chacha20poly1305_keygen(k);
}
public static int crypto_aead_chacha20poly1305_encrypt(byte[] c, int[] clen_p, byte[] m, int mlen, byte[] ad, int adlen, byte[] nsec, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_chacha20poly1305_encrypt(c, clen_p, m, mlen, ad, adlen, nsec, npub, k);
}
public static int crypto_aead_chacha20poly1305_encrypt_detached(byte[] c, byte[] mac, int[] maclen_p, byte[] m, int mlen, byte[] ad, int adlen, byte[] nsec, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_chacha20poly1305_encrypt_detached(c, mac, maclen_p, m, mlen, ad, adlen, nsec, npub, k);
}
public static int crypto_aead_chacha20poly1305_decrypt(byte[] m, int[] mlen_p, byte[] nsec, byte[] c, int clen, byte[] ad, int adlen, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_chacha20poly1305_decrypt(m, mlen_p, nsec, c, clen, ad, adlen, npub, k);
}
public static int crypto_aead_chacha20poly1305_decrypt_detached(byte[] m, byte[] nsec, byte[] c, int clen, byte[] mac, byte[] ad, int adlen, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_chacha20poly1305_decrypt_detached(m, nsec, c, clen, mac, ad, adlen, npub, k);
}
public static int crypto_aead_chacha20poly1305_ietf_keybytes() {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_keybytes();
}
public static int crypto_aead_chacha20poly1305_ietf_nsecbytes() {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_nsecbytes();
}
public static int crypto_aead_chacha20poly1305_ietf_abytes() {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_abytes();
}
public static int crypto_aead_chacha20poly1305_ietf_messagebytes_max() {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_messagebytes_max();
}
public static int crypto_aead_chacha20poly1305_ietf_npubbytes() {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_npubbytes();
}
public static void crypto_aead_chacha20poly1305_ietf_keygen(byte[] k) {
SodiumJNI.crypto_aead_chacha20poly1305_ietf_keygen(k);
}
public static int crypto_aead_chacha20poly1305_ietf_encrypt(byte[] c, int[] clen_p, byte[] m, int mlen, byte[] ad, int adlen, byte[] nsec, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_encrypt(c, clen_p, m, mlen, ad, adlen, nsec, npub, k);
}
public static int crypto_aead_chacha20poly1305_ietf_encrypt_detached(byte[] c, byte[] mac, int[] maclen_p, byte[] m, int mlen, byte[] ad, int adlen, byte[] nsec, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_encrypt_detached(c, mac, maclen_p, m, mlen, ad, adlen, nsec, npub, k);
}
public static int crypto_aead_chacha20poly1305_ietf_decrypt(byte[] m, int[] mlen_p, byte[] nsec, byte[] c, int clen, byte[] ad, int adlen, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_decrypt(m, mlen_p, nsec, c, clen, ad, adlen, npub, k);
}
public static int crypto_aead_chacha20poly1305_ietf_decrypt_detached(byte[] m, byte[] nsec, byte[] c, int clen, byte[] mac, byte[] ad, int adlen, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_chacha20poly1305_ietf_decrypt_detached(m, nsec, c, clen, mac, ad, adlen, npub, k);
}
public static int crypto_aead_xchacha20poly1305_ietf_keybytes() {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_keybytes();
}
public static int crypto_aead_xchacha20poly1305_ietf_npubbytes() {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_npubbytes();
}
public static int crypto_aead_xchacha20poly1305_ietf_nsecbytes() {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_nsecbytes();
}
public static int crypto_aead_xchacha20poly1305_ietf_abytes() {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_abytes();
}
public static int crypto_aead_xchacha20poly1305_ietf_messagebytes_max() {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_messagebytes_max();
}
public static void crypto_aead_xchacha20poly1305_ietf_keygen(byte[] k) {
SodiumJNI.crypto_aead_xchacha20poly1305_ietf_keygen(k);
}
public static int crypto_aead_xchacha20poly1305_ietf_encrypt_detached(byte[] c, byte[] mac, int[] maclen_p, byte[] m, int mlen, byte[] ad, int adlen, byte[] nsec, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_encrypt_detached(c, mac, maclen_p, m, mlen, ad, adlen, nsec, npub, k);
}
public static int crypto_aead_xchacha20poly1305_ietf_encrypt(byte[] c, int[] clen_p, byte[] m, int mlen, byte[] ad, int adlen, byte[] nsec, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_encrypt(c, clen_p, m, mlen, ad, adlen, nsec, npub, k);
}
public static int crypto_aead_xchacha20poly1305_ietf_decrypt_detached(byte[] m, byte[] nsec, byte[] c, int clen, byte[] mac, byte[] ad, int adlen, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_decrypt_detached(m, nsec, c, clen, mac, ad, adlen, npub, k);
}
public static int crypto_aead_xchacha20poly1305_ietf_decrypt(byte[] m, int[] mlen_p, byte[] nsec, byte[] c, int clen, byte[] ad, int adlen, byte[] npub, byte[] k) {
return SodiumJNI.crypto_aead_xchacha20poly1305_ietf_decrypt(m, mlen_p, nsec, c, clen, ad, adlen, npub, k);
}
public static int crypto_auth_hmacsha256_bytes() {
return SodiumJNI.crypto_auth_hmacsha256_bytes();
}
public static int crypto_auth_hmacsha256_keybytes() {
return SodiumJNI.crypto_auth_hmacsha256_keybytes();
}
public static int crypto_auth_hmacsha256(byte[] out, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_auth_hmacsha256(out, in, inlen, k);
}
public static int crypto_auth_hmacsha256_verify(byte[] h, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_auth_hmacsha256_verify(h, in, inlen, k);
}
public static int crypto_auth_hmacsha256_statebytes() {
return SodiumJNI.crypto_auth_hmacsha256_statebytes();
}
public static int crypto_auth_hmacsha256_init(byte[] state, byte[] key, int keylen) {
return SodiumJNI.crypto_auth_hmacsha256_init(state, key, keylen);
}
public static int crypto_auth_hmacsha256_update(byte[] state, byte[] in, int inlen) {
return SodiumJNI.crypto_auth_hmacsha256_update(state, in, inlen);
}
public static int crypto_auth_hmacsha256_final(byte[] state, byte[] out) {
return SodiumJNI.crypto_auth_hmacsha256_final(state, out);
}
public static int crypto_auth_hmacsha512_bytes() {
return SodiumJNI.crypto_auth_hmacsha512_bytes();
}
public static int crypto_auth_hmacsha512_keybytes() {
return SodiumJNI.crypto_auth_hmacsha512_keybytes();
}
public static int crypto_auth_hmacsha512(byte[] out, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_auth_hmacsha512(out, in, inlen, k);
}
public static int crypto_auth_hmacsha512_verify(byte[] h, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_auth_hmacsha512_verify(h, in, inlen, k);
}
public static int crypto_auth_hmacsha512_statebytes() {
return SodiumJNI.crypto_auth_hmacsha512_statebytes();
}
public static int crypto_auth_hmacsha512_init(byte[] state, byte[] key, int keylen) {
return SodiumJNI.crypto_auth_hmacsha512_init(state, key, keylen);
}
public static int crypto_auth_hmacsha512_update(byte[] state, byte[] in, int inlen) {
return SodiumJNI.crypto_auth_hmacsha512_update(state, in, inlen);
}
public static int crypto_auth_hmacsha512_final(byte[] state, byte[] out) {
return SodiumJNI.crypto_auth_hmacsha512_final(state, out);
}
public static int crypto_auth_hmacsha512256_bytes() {
return SodiumJNI.crypto_auth_hmacsha512256_bytes();
}
public static int crypto_auth_hmacsha512256_keybytes() {
return SodiumJNI.crypto_auth_hmacsha512256_keybytes();
}
public static int crypto_auth_hmacsha512256(byte[] out, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_auth_hmacsha512256(out, in, inlen, k);
}
public static int crypto_auth_hmacsha512256_verify(byte[] h, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_auth_hmacsha512256_verify(h, in, inlen, k);
}
public static int crypto_auth_hmacsha512256_statebytes() {
return SodiumJNI.crypto_auth_hmacsha512256_statebytes();
}
public static int crypto_auth_hmacsha512256_init(byte[] state, byte[] key, int keylen) {
return SodiumJNI.crypto_auth_hmacsha512256_init(state, key, keylen);
}
public static int crypto_auth_hmacsha512256_update(byte[] state, byte[] in, int inlen) {
return SodiumJNI.crypto_auth_hmacsha512256_update(state, in, inlen);
}
public static int crypto_auth_hmacsha512256_final(byte[] state, byte[] out) {
return SodiumJNI.crypto_auth_hmacsha512256_final(state, out);
}
public static int crypto_box_curve25519xsalsa20poly1305_seedbytes() {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_seedbytes();
}
public static int crypto_box_curve25519xsalsa20poly1305_publickeybytes() {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_publickeybytes();
}
public static int crypto_box_curve25519xsalsa20poly1305_secretkeybytes() {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_secretkeybytes();
}
public static int crypto_box_curve25519xsalsa20poly1305_beforenmbytes() {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_beforenmbytes();
}
public static int crypto_box_curve25519xsalsa20poly1305_noncebytes() {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_noncebytes();
}
public static int crypto_box_curve25519xsalsa20poly1305_zerobytes() {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_zerobytes();
}
public static int crypto_box_curve25519xsalsa20poly1305_boxzerobytes() {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_boxzerobytes();
}
public static int crypto_box_curve25519xsalsa20poly1305_macbytes() {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_macbytes();
}
public static int crypto_box_curve25519xsalsa20poly1305(byte[] c, byte[] m, int mlen, byte[] n, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305(c, m, mlen, n, pk, sk);
}
public static int crypto_box_curve25519xsalsa20poly1305_open(byte[] m, byte[] c, int clen, byte[] n, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_open(m, c, clen, n, pk, sk);
}
public static int crypto_box_curve25519xsalsa20poly1305_seed_keypair(byte[] pk, byte[] sk, byte[] seed) {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_seed_keypair(pk, sk, seed);
}
public static int crypto_box_curve25519xsalsa20poly1305_keypair(byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_keypair(pk, sk);
}
public static int crypto_box_curve25519xsalsa20poly1305_beforenm(byte[] k, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_beforenm(k, pk, sk);
}
public static int crypto_box_curve25519xsalsa20poly1305_afternm(byte[] c, byte[] m, int mlen, byte[] n, byte[] k) {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_afternm(c, m, mlen, n, k);
}
public static int crypto_box_curve25519xsalsa20poly1305_open_afternm(byte[] m, byte[] c, int clen, byte[] n, byte[] k) {
return SodiumJNI.crypto_box_curve25519xsalsa20poly1305_open_afternm(m, c, clen, n, k);
}
public static int crypto_core_hsalsa20_outputbytes() {
return SodiumJNI.crypto_core_hsalsa20_outputbytes();
}
public static int crypto_core_hsalsa20_inputbytes() {
return SodiumJNI.crypto_core_hsalsa20_inputbytes();
}
public static int crypto_core_hsalsa20_keybytes() {
return SodiumJNI.crypto_core_hsalsa20_keybytes();
}
public static int crypto_core_hsalsa20_constbytes() {
return SodiumJNI.crypto_core_hsalsa20_constbytes();
}
public static int crypto_core_hsalsa20(byte[] out, byte[] in, byte[] k, byte[] c) {
return SodiumJNI.crypto_core_hsalsa20(out, in, k, c);
}
public static int crypto_core_salsa20_outputbytes() {
return SodiumJNI.crypto_core_salsa20_outputbytes();
}
public static int crypto_core_salsa20_inputbytes() {
return SodiumJNI.crypto_core_salsa20_inputbytes();
}
public static int crypto_core_salsa20_keybytes() {
return SodiumJNI.crypto_core_salsa20_keybytes();
}
public static int crypto_core_salsa20_constbytes() {
return SodiumJNI.crypto_core_salsa20_constbytes();
}
public static int crypto_core_salsa20(byte[] out, byte[] in, byte[] k, byte[] c) {
return SodiumJNI.crypto_core_salsa20(out, in, k, c);
}
public static int crypto_generichash_blake2b_bytes_min() {
return SodiumJNI.crypto_generichash_blake2b_bytes_min();
}
public static int crypto_generichash_blake2b_bytes_max() {
return SodiumJNI.crypto_generichash_blake2b_bytes_max();
}
public static int crypto_generichash_blake2b_bytes() {
return SodiumJNI.crypto_generichash_blake2b_bytes();
}
public static int crypto_generichash_blake2b_keybytes_min() {
return SodiumJNI.crypto_generichash_blake2b_keybytes_min();
}
public static int crypto_generichash_blake2b_keybytes_max() {
return SodiumJNI.crypto_generichash_blake2b_keybytes_max();
}
public static int crypto_generichash_blake2b_keybytes() {
return SodiumJNI.crypto_generichash_blake2b_keybytes();
}
public static int crypto_generichash_blake2b_saltbytes() {
return SodiumJNI.crypto_generichash_blake2b_saltbytes();
}
public static int crypto_generichash_blake2b_personalbytes() {
return SodiumJNI.crypto_generichash_blake2b_personalbytes();
}
public static int crypto_generichash_blake2b(byte[] out, int outlen, byte[] in, int inlen, byte[] key, int keylen) {
return SodiumJNI.crypto_generichash_blake2b(out, outlen, in, inlen, key, keylen);
}
public static int crypto_generichash_blake2b_salt_personal(byte[] out, int outlen, byte[] in, int inlen, byte[] key, int keylen, byte[] salt, byte[] personal) {
return SodiumJNI.crypto_generichash_blake2b_salt_personal(out, outlen, in, inlen, key, keylen, salt, personal);
}
public static int crypto_generichash_blake2b_init(byte[] state, byte[] key, int keylen, int outlen) {
return SodiumJNI.crypto_generichash_blake2b_init(state, key, keylen, outlen);
}
public static int crypto_generichash_blake2b_init_salt_personal(byte[] state, byte[] key, int keylen, int outlen, byte[] salt, byte[] personal) {
return SodiumJNI.crypto_generichash_blake2b_init_salt_personal(state, key, keylen, outlen, salt, personal);
}
public static int crypto_generichash_blake2b_update(byte[] state, byte[] in, int inlen) {
return SodiumJNI.crypto_generichash_blake2b_update(state, in, inlen);
}
public static int crypto_generichash_blake2b_final(byte[] state, byte[] out, int outlen) {
return SodiumJNI.crypto_generichash_blake2b_final(state, out, outlen);
}
public static int crypto_hash_sha256_bytes() {
return SodiumJNI.crypto_hash_sha256_bytes();
}
public static int crypto_hash_sha256(byte[] out, byte[] in, int inlen) {
return SodiumJNI.crypto_hash_sha256(out, in, inlen);
}
public static int crypto_hash_sha256_statebytes() {
return SodiumJNI.crypto_hash_sha256_statebytes();
}
public static int crypto_hash_sha256_init(byte[] state) {
return SodiumJNI.crypto_hash_sha256_init(state);
}
public static int crypto_hash_sha256_update(byte[] state, byte[] in, int inlen) {
return SodiumJNI.crypto_hash_sha256_update(state, in, inlen);
}
public static int crypto_hash_sha256_final(byte[] state, byte[] out) {
return SodiumJNI.crypto_hash_sha256_final(state, out);
}
public static int crypto_hash_sha512_bytes() {
return SodiumJNI.crypto_hash_sha512_bytes();
}
public static int crypto_hash_sha512(byte[] out, byte[] in, int inlen) {
return SodiumJNI.crypto_hash_sha512(out, in, inlen);
}
public static int crypto_hash_sha512_statebytes() {
return SodiumJNI.crypto_hash_sha512_statebytes();
}
public static int crypto_hash_sha512_init(byte[] state) {
return SodiumJNI.crypto_hash_sha512_init(state);
}
public static int crypto_hash_sha512_update(byte[] state, byte[] in, int inlen) {
return SodiumJNI.crypto_hash_sha512_update(state, in, inlen);
}
public static int crypto_hash_sha512_final(byte[] state, byte[] out) {
return SodiumJNI.crypto_hash_sha512_final(state, out);
}
public static int crypto_onetimeauth_poly1305_bytes() {
return SodiumJNI.crypto_onetimeauth_poly1305_bytes();
}
public static int crypto_onetimeauth_poly1305_keybytes() {
return SodiumJNI.crypto_onetimeauth_poly1305_keybytes();
}
public static int crypto_onetimeauth_poly1305(byte[] out, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_onetimeauth_poly1305(out, in, inlen, k);
}
public static int crypto_onetimeauth_poly1305_verify(byte[] h, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_onetimeauth_poly1305_verify(h, in, inlen, k);
}
public static int crypto_onetimeauth_poly1305_init(byte[] state, byte[] key) {
return SodiumJNI.crypto_onetimeauth_poly1305_init(state, key);
}
public static int crypto_onetimeauth_poly1305_update(byte[] state, byte[] in, int inlen) {
return SodiumJNI.crypto_onetimeauth_poly1305_update(state, in, inlen);
}
public static int crypto_onetimeauth_poly1305_final(byte[] state, byte[] out) {
return SodiumJNI.crypto_onetimeauth_poly1305_final(state, out);
}
public static int crypto_pwhash_alg_argon2i13() {
return SodiumJNI.crypto_pwhash_alg_argon2i13();
}
public static int crypto_pwhash_alg_default() {
return SodiumJNI.crypto_pwhash_alg_default();
}
public static int crypto_pwhash_bytes_min() {
return SodiumJNI.crypto_pwhash_bytes_min();
}
public static int crypto_pwhash_bytes_max() {
return SodiumJNI.crypto_pwhash_bytes_max();
}
public static int crypto_pwhash_passwd_min() {
return SodiumJNI.crypto_pwhash_passwd_min();
}
public static int crypto_pwhash_passwd_max() {
return SodiumJNI.crypto_pwhash_passwd_max();
}
public static int crypto_pwhash_saltbytes() {
return SodiumJNI.crypto_pwhash_saltbytes();
}
public static int crypto_pwhash_strbytes() {
return SodiumJNI.crypto_pwhash_strbytes();
}
public static byte[] crypto_pwhash_strprefix() {
return SodiumJNI.crypto_pwhash_strprefix();
}
public static int crypto_pwhash_opslimit_min() {
return SodiumJNI.crypto_pwhash_opslimit_min();
}
public static int crypto_pwhash_opslimit_max() {
return SodiumJNI.crypto_pwhash_opslimit_max();
}
public static int crypto_pwhash_memlimit_min() {
return SodiumJNI.crypto_pwhash_memlimit_min();
}
public static int crypto_pwhash_memlimit_max() {
return SodiumJNI.crypto_pwhash_memlimit_max();
}
public static int crypto_pwhash_opslimit_interactive() {
return SodiumJNI.crypto_pwhash_opslimit_interactive();
}
public static int crypto_pwhash_memlimit_interactive() {
return SodiumJNI.crypto_pwhash_memlimit_interactive();
}
public static int crypto_pwhash_opslimit_moderate() {
return SodiumJNI.crypto_pwhash_opslimit_moderate();
}
public static int crypto_pwhash_memlimit_moderate() {
return SodiumJNI.crypto_pwhash_memlimit_moderate();
}
public static int crypto_pwhash_opslimit_sensitive() {
return SodiumJNI.crypto_pwhash_opslimit_sensitive();
}
public static int crypto_pwhash_memlimit_sensitive() {
return SodiumJNI.crypto_pwhash_memlimit_sensitive();
}
public static int crypto_pwhash(byte[] out, int outlen, byte[] passwd, int passwdlen, byte[] salt, int opslimit, int memlimit, int alg) {
return SodiumJNI.crypto_pwhash(out, outlen, passwd, passwdlen, salt, opslimit, memlimit, alg);
}
public static int crypto_pwhash_str(byte[] out, byte[] passwd, int passwdlen, int opslimit, int memlimit) {
return SodiumJNI.crypto_pwhash_str(out, passwd, passwdlen, opslimit, memlimit);
}
public static int crypto_pwhash_str_verify(byte[] str, byte[] passwd, int passwdlen) {
return SodiumJNI.crypto_pwhash_str_verify(str, passwd, passwdlen);
}
public static byte[] crypto_pwhash_primitive() {
return SodiumJNI.crypto_pwhash_primitive();
}
public static int crypto_pwhash_scryptsalsa208sha256_saltbytes() {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_saltbytes();
}
public static int crypto_pwhash_scryptsalsa208sha256_strbytes() {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_strbytes();
}
public static byte[] crypto_pwhash_scryptsalsa208sha256_strprefix() {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_strprefix();
}
public static int crypto_pwhash_scryptsalsa208sha256_opslimit_interactive() {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_opslimit_interactive();
}
public static int crypto_pwhash_scryptsalsa208sha256_memlimit_interactive() {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_memlimit_interactive();
}
public static int crypto_pwhash_scryptsalsa208sha256_opslimit_sensitive() {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_opslimit_sensitive();
}
public static int crypto_pwhash_scryptsalsa208sha256_memlimit_sensitive() {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_memlimit_sensitive();
}
public static int crypto_pwhash_scryptsalsa208sha256(byte[] out, int outlen, byte[] passwd, int passwdlen, byte[] salt, int opslimit, int memlimit) {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256(out, outlen, passwd, passwdlen, salt, opslimit, memlimit);
}
public static int crypto_pwhash_scryptsalsa208sha256_str(byte[] out, byte[] passwd, int passwdlen, int opslimit, int memlimit) {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_str(out, passwd, passwdlen, opslimit, memlimit);
}
public static int crypto_pwhash_scryptsalsa208sha256_str_verify(byte[] str, byte[] passwd, int passwdlen) {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_str_verify(str, passwd, passwdlen);
}
public static int crypto_pwhash_scryptsalsa208sha256_ll(byte[] passwd, int passwdlen, byte[] salt, int saltlen, int N, int r, int p, byte[] buf, int buflen) {
return SodiumJNI.crypto_pwhash_scryptsalsa208sha256_ll(passwd, passwdlen, salt, saltlen, N, r, p, buf, buflen);
}
public static int crypto_scalarmult_curve25519_bytes() {
return SodiumJNI.crypto_scalarmult_curve25519_bytes();
}
public static int crypto_scalarmult_curve25519_scalarbytes() {
return SodiumJNI.crypto_scalarmult_curve25519_scalarbytes();
}
public static int crypto_scalarmult_curve25519(byte[] q, byte[] n, byte[] p) {
return SodiumJNI.crypto_scalarmult_curve25519(q, n, p);
}
public static int crypto_scalarmult_curve25519_base(byte[] q, byte[] n) {
return SodiumJNI.crypto_scalarmult_curve25519_base(q, n);
}
public static int crypto_secretbox_xsalsa20poly1305_keybytes() {
return SodiumJNI.crypto_secretbox_xsalsa20poly1305_keybytes();
}
public static int crypto_secretbox_xsalsa20poly1305_noncebytes() {
return SodiumJNI.crypto_secretbox_xsalsa20poly1305_noncebytes();
}
public static int crypto_secretbox_xsalsa20poly1305_zerobytes() {
return SodiumJNI.crypto_secretbox_xsalsa20poly1305_zerobytes();
}
public static int crypto_secretbox_xsalsa20poly1305_boxzerobytes() {
return SodiumJNI.crypto_secretbox_xsalsa20poly1305_boxzerobytes();
}
public static int crypto_secretbox_xsalsa20poly1305_macbytes() {
return SodiumJNI.crypto_secretbox_xsalsa20poly1305_macbytes();
}
public static int crypto_secretbox_xsalsa20poly1305(byte[] c, byte[] m, int mlen, byte[] n, byte[] k) {
return SodiumJNI.crypto_secretbox_xsalsa20poly1305(c, m, mlen, n, k);
}
public static int crypto_secretbox_xsalsa20poly1305_open(byte[] m, byte[] c, int clen, byte[] n, byte[] k) {
return SodiumJNI.crypto_secretbox_xsalsa20poly1305_open(m, c, clen, n, k);
}
public static int crypto_shorthash_siphash24_bytes() {
return SodiumJNI.crypto_shorthash_siphash24_bytes();
}
public static int crypto_shorthash_siphash24_keybytes() {
return SodiumJNI.crypto_shorthash_siphash24_keybytes();
}
public static int crypto_shorthash_siphash24(byte[] out, byte[] in, int inlen, byte[] k) {
return SodiumJNI.crypto_shorthash_siphash24(out, in, inlen, k);
}
public static int crypto_sign_ed25519_bytes() {
return SodiumJNI.crypto_sign_ed25519_bytes();
}
public static int crypto_sign_ed25519_seedbytes() {
return SodiumJNI.crypto_sign_ed25519_seedbytes();
}
public static int crypto_sign_ed25519_publickeybytes() {
return SodiumJNI.crypto_sign_ed25519_publickeybytes();
}
public static int crypto_sign_ed25519_secretkeybytes() {
return SodiumJNI.crypto_sign_ed25519_secretkeybytes();
}
public static int crypto_sign_ed25519(byte[] sm, int[] smlen_p, byte[] m, int mlen, byte[] sk) {
return SodiumJNI.crypto_sign_ed25519(sm, smlen_p, m, mlen, sk);
}
public static int crypto_sign_ed25519_open(byte[] m, int[] mlen_p, byte[] sm, int smlen, byte[] pk) {
return SodiumJNI.crypto_sign_ed25519_open(m, mlen_p, sm, smlen, pk);
}
public static int crypto_stream_xsalsa20(byte[] c, int clen, byte[] n, byte[] k) {
return SodiumJNI.crypto_stream_xsalsa20(c, clen, n, k);
}
public static int crypto_sign_ed25519_detached(byte[] sig, int[] siglen_p, byte[] m, int mlen, byte[] sk) {
return SodiumJNI.crypto_sign_ed25519_detached(sig, siglen_p, m, mlen, sk);
}
public static int crypto_sign_ed25519_verify_detached(byte[] sig, byte[] m, int mlen, byte[] pk) {
return SodiumJNI.crypto_sign_ed25519_verify_detached(sig, m, mlen, pk);
}
public static int crypto_sign_ed25519_keypair(byte[] pk, byte[] sk) {
return SodiumJNI.crypto_sign_ed25519_keypair(pk, sk);
}
public static int crypto_sign_ed25519_seed_keypair(byte[] pk, byte[] sk, byte[] seed) {
return SodiumJNI.crypto_sign_ed25519_seed_keypair(pk, sk, seed);
}
public static int crypto_sign_ed25519_pk_to_curve25519(byte[] curve25519_pk, byte[] ed25519_pk) {
return SodiumJNI.crypto_sign_ed25519_pk_to_curve25519(curve25519_pk, ed25519_pk);
}
public static int crypto_sign_ed25519_sk_to_curve25519(byte[] curve25519_sk, byte[] ed25519_sk) {
return SodiumJNI.crypto_sign_ed25519_sk_to_curve25519(curve25519_sk, ed25519_sk);
}
public static int crypto_stream_chacha20_keybytes() {
return SodiumJNI.crypto_stream_chacha20_keybytes();
}
public static int crypto_stream_chacha20_noncebytes() {
return SodiumJNI.crypto_stream_chacha20_noncebytes();
}
public static int crypto_stream_chacha20(byte[] c, int clen, byte[] n, byte[] k) {
return SodiumJNI.crypto_stream_chacha20(c, clen, n, k);
}
public static int crypto_stream_chacha20_xor(byte[] c, byte[] m, int mlen, byte[] n, byte[] k) {
return SodiumJNI.crypto_stream_chacha20_xor(c, m, mlen, n, k);
}
public static int crypto_stream_chacha20_xor_ic(byte[] c, byte[] m, int mlen, byte[] n, int ic, byte[] k) {
return SodiumJNI.crypto_stream_chacha20_xor_ic(c, m, mlen, n, ic, k);
}
public static int crypto_stream_chacha20_ietf_noncebytes() {
return SodiumJNI.crypto_stream_chacha20_ietf_noncebytes();
}
public static int crypto_stream_chacha20_ietf(byte[] c, int clen, byte[] n, byte[] k) {
return SodiumJNI.crypto_stream_chacha20_ietf(c, clen, n, k);
}
public static int crypto_stream_chacha20_ietf_xor(byte[] c, byte[] m, int mlen, byte[] n, byte[] k) {
return SodiumJNI.crypto_stream_chacha20_ietf_xor(c, m, mlen, n, k);
}
public static int crypto_stream_chacha20_ietf_xor_ic(byte[] c, byte[] m, int mlen, byte[] n, int ic, byte[] k) {
return SodiumJNI.crypto_stream_chacha20_ietf_xor_ic(c, m, mlen, n, ic, k);
}
public static int crypto_stream_salsa20_keybytes() {
return SodiumJNI.crypto_stream_salsa20_keybytes();
}
public static int crypto_stream_salsa20_noncebytes() {
return SodiumJNI.crypto_stream_salsa20_noncebytes();
}
public static int crypto_stream_salsa20(byte[] c, int clen, byte[] n, byte[] k) {
return SodiumJNI.crypto_stream_salsa20(c, clen, n, k);
}
public static int crypto_stream_salsa20_xor(byte[] c, byte[] m, int mlen, byte[] n, byte[] k) {
return SodiumJNI.crypto_stream_salsa20_xor(c, m, mlen, n, k);
}
public static int crypto_stream_salsa20_xor_ic(byte[] c, byte[] m, int mlen, byte[] n, int ic, byte[] k) {
return SodiumJNI.crypto_stream_salsa20_xor_ic(c, m, mlen, n, ic, k);
}
public static int crypto_stream_xsalsa20_keybytes() {
return SodiumJNI.crypto_stream_xsalsa20_keybytes();
}
public static int crypto_stream_xsalsa20_noncebytes() {
return SodiumJNI.crypto_stream_xsalsa20_noncebytes();
}
public static int crypto_stream_xsalsa20_xor(byte[] c, byte[] m, int mlen, byte[] n, byte[] k) {
return SodiumJNI.crypto_stream_xsalsa20_xor(c, m, mlen, n, k);
}
public static int crypto_stream_xsalsa20_xor_ic(byte[] c, byte[] m, int mlen, byte[] n, int ic, byte[] k) {
return SodiumJNI.crypto_stream_xsalsa20_xor_ic(c, m, mlen, n, ic, k);
}
public static int crypto_secretstream_xchacha20poly1305_keybytes() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_keybytes();
}
public static int crypto_secretstream_xchacha20poly1305_headerbytes() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_headerbytes();
}
public static int crypto_secretstream_xchacha20poly1305_abytes() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_abytes();
}
public static int crypto_secretstream_xchacha20poly1305_statebytes() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_statebytes();
}
public static int crypto_secretstream_xchacha20poly1305_tag_message() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_tag_message();
}
public static int crypto_secretstream_xchacha20poly1305_tag_push() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_tag_push();
}
public static int crypto_secretstream_xchacha20poly1305_tag_rekey() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_tag_rekey();
}
public static int crypto_secretstream_xchacha20poly1305_tag_final() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_tag_final();
}
public static int crypto_secretstream_xchacha20poly1305_messagebytes_max() {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_messagebytes_max();
}
public static void crypto_secretstream_xchacha20poly1305_keygen(byte[] k) {
SodiumJNI.crypto_secretstream_xchacha20poly1305_keygen(k);
}
public static int crypto_secretstream_xchacha20poly1305_init_push(byte[] state, byte[] header, byte[] k) {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_init_push(state, header, k);
}
public static int crypto_secretstream_xchacha20poly1305_push(byte[] state, byte[] c, int[] clen_p, byte[] m, int mlen, byte[] ad, int adlen, short tag) {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_push(state, c, clen_p, m, mlen, ad, adlen, tag);
}
public static int crypto_secretstream_xchacha20poly1305_init_pull(byte[] state, byte[] header, byte[] k) {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_init_pull(state, header, k);
}
public static int crypto_secretstream_xchacha20poly1305_pull(byte[] state, byte[] m, int[] mlen_p, byte[] tag_p, byte[] c, int clen, byte[] ad, int adlen) {
return SodiumJNI.crypto_secretstream_xchacha20poly1305_pull(state, m, mlen_p, tag_p, c, clen, ad, adlen);
}
public static void crypto_secretstream_xchacha20poly1305_rekey(byte[] state) {
SodiumJNI.crypto_secretstream_xchacha20poly1305_rekey(state);
}
public static int crypto_box_curve25519xchacha20poly1305_seedbytes() {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_seedbytes();
}
public static int crypto_box_curve25519xchacha20poly1305_publickeybytes() {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_publickeybytes();
}
public static int crypto_box_curve25519xchacha20poly1305_secretkeybytes() {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_secretkeybytes();
}
public static int crypto_box_curve25519xchacha20poly1305_beforenmbytes() {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_beforenmbytes();
}
public static int crypto_box_curve25519xchacha20poly1305_noncebytes() {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_noncebytes();
}
public static int crypto_box_curve25519xchacha20poly1305_macbytes() {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_macbytes();
}
public static int crypto_box_curve25519xchacha20poly1305_messagebytes_max() {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_messagebytes_max();
}
public static int crypto_box_curve25519xchacha20poly1305_sealbytes() {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_sealbytes();
}
public static int crypto_box_curve25519xchacha20poly1305_seed_keypair(byte[] pk, byte[] sk, byte[] seed) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_seed_keypair(pk, sk, seed);
}
public static int crypto_box_curve25519xchacha20poly1305_keypair(byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_keypair(pk, sk);
}
public static int crypto_box_curve25519xchacha20poly1305_beforenm(byte[] k, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_beforenm(k, pk, sk);
}
public static int crypto_box_curve25519xchacha20poly1305_detached_afternm(byte[] c, byte[] mac, byte[] m, int mlen, byte[] n, byte[] k) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_detached_afternm(c, mac, m, mlen, n, k);
}
public static int crypto_box_curve25519xchacha20poly1305_detached(byte[] c, byte[] mac, byte[] m, int mlen, byte[] n, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_detached(c, mac, m, mlen, n, pk, sk);
}
public static int crypto_box_curve25519xchacha20poly1305_easy_afternm(byte[] c, byte[] m, int mlen, byte[] n, byte[] k) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_easy_afternm(c, m, mlen, n, k);
}
public static int crypto_box_curve25519xchacha20poly1305_easy(byte[] c, byte[] m, int mlen, byte[] n, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_easy(c, m, mlen, n, pk, sk);
}
public static int crypto_box_curve25519xchacha20poly1305_open_detached_afternm(byte[] m, byte[] c, byte[] mac, int clen, byte[] n, byte[] k) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_open_detached_afternm(m, c, mac, clen, n, k);
}
public static int crypto_box_curve25519xchacha20poly1305_open_detached(byte[] m, byte[] c, byte[] mac, int clen, byte[] n, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_open_detached(m, c, mac, clen, n, pk, sk);
}
public static int crypto_box_curve25519xchacha20poly1305_open_easy_afternm(byte[] m, byte[] c, int clen, byte[] n, byte[] k) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_open_easy_afternm(m, c, clen, n, k);
}
public static int crypto_box_curve25519xchacha20poly1305_open_easy(byte[] m, byte[] c, int clen, byte[] n, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_open_easy(m, c, clen, n, pk, sk);
}
public static int crypto_box_curve25519xchacha20poly1305_seal(byte[] c, byte[] m, int mlen, byte[] pk) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_seal(c, m, mlen, pk);
}
public static int crypto_box_curve25519xchacha20poly1305_seal_open(byte[] m, byte[] c, int clen, byte[] pk, byte[] sk) {
return SodiumJNI.crypto_box_curve25519xchacha20poly1305_seal_open(m, c, clen, pk, sk);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy