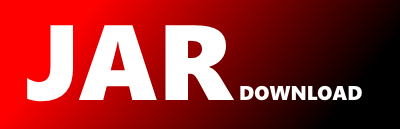
com.github.jsonldjava.core.UniqueNamer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsonld-java Show documentation
Show all versions of jsonld-java Show documentation
Json-LD core implementation
package com.github.jsonldjava.core;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
public class UniqueNamer {
private String prefix;
private int counter;
private Map existing;
/**
* Creates a new UniqueNamer. A UniqueNamer issues unique names, keeping
* track of any previously issued names.
*
* @param prefix the prefix to use ('').
*/
public UniqueNamer(String prefix) {
this.prefix = prefix;
this.counter = 0;
this.existing = new LinkedHashMap();
}
/**
* Copies this UniqueNamer.
*
* @return a copy of this UniqueNamer.
*/
public UniqueNamer clone() {
UniqueNamer copy = new UniqueNamer(this.prefix);
copy.counter = this.counter;
copy.existing = (Map) JSONLDUtils.clone(this.existing);
return copy;
}
/**
* Gets the new name for the given old name, where if no old name is given
* a new name will be generated.
*
* @param [oldName] the old name to get the new name for.
*
* @return the new name.
*/
public String getName(String oldName) {
if (oldName != null && this.existing.containsKey(oldName)) {
return this.existing.get(oldName);
}
String name = this.prefix + this.counter;
this.counter++;
if (oldName != null) {
this.existing.put(oldName, name);
}
return name;
}
public String getName() {
return getName(null);
}
public Boolean isNamed(String oldName) {
return this.existing.containsKey(oldName);
}
public Map existing() {
return existing;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy