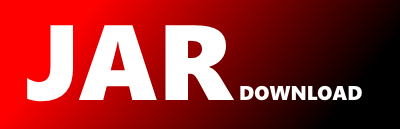
org.jsfr.json.JsonPosition Maven / Gradle / Ivy
The newest version!
/*
* The MIT License
*
* Copyright (c) 2015 WANG Lingsong
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.jsfr.json;
import org.jsfr.json.path.ArrayIndex;
import org.jsfr.json.path.ChildNode;
import org.jsfr.json.path.JsonPath;
import org.jsfr.json.path.PathOperator;
import org.jsfr.json.path.Root;
import java.lang.ref.SoftReference;
import java.util.Stack;
/**
* Created by Leo on 2015/3/27.
*/
class JsonPosition extends JsonPath {
//TODO recycling node for saving gc
private SoftReference> childNodeCache = new SoftReference>(new Stack());
private SoftReference> arrayNodeCache = new SoftReference>(new Stack());
static JsonPosition start() {
JsonPosition newPath = new JsonPosition();
newPath.operators.push(Root.instance());
return newPath;
}
void stepIntoObject() {
Stack stack = childNodeCache.get();
ChildNode node = null;
if (stack != null && !stack.isEmpty()) {
node = stack.pop();
node.setKey(null);
}
if (node == null) {
node = new ChildNode(null);
}
operators.push(node);
}
void updateObjectEntry(String key) {
((ChildNode)operators.peek()).setKey(key);
}
void stepOutObject() {
PathOperator node = operators.pop();
Stack stack = childNodeCache.get();
if (stack != null) {
stack.push((ChildNode) node);
} else {
createChildNodeCache();
}
}
void stepIntoArray() {
Stack stack = arrayNodeCache.get();
ArrayIndex node = null;
if (stack != null && !stack.isEmpty()) {
node = stack.pop();
node.reset();
}
if (node == null) {
node = new ArrayIndex();
}
operators.push(node);
}
boolean accumulateArrayIndex() {
PathOperator top = this.peek();
if (top.getType() == PathOperator.Type.ARRAY) {
((ArrayIndex) top).increaseArrayIndex();
return true;
}
return false;
}
void stepOutArray() {
PathOperator node = operators.pop();
Stack stack = arrayNodeCache.get();
if (stack != null) {
stack.push((ArrayIndex) node);
} else {
createArrayNodeCache();
}
}
void clear() {
operators.clear();
operators = null;
childNodeCache = null;
arrayNodeCache = null;
}
private void createChildNodeCache() {
childNodeCache = new SoftReference>(new Stack());
}
private void createArrayNodeCache() {
arrayNodeCache = new SoftReference>(new Stack());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy