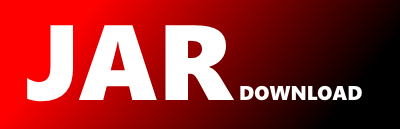
artoria.action.hutool.AbstractHutoolSmallExcelImportHandler Maven / Gradle / Ivy
Show all versions of artoria-extend Show documentation
package artoria.action.hutool;
import artoria.action.handler.AbstractImportExportActionHandler;
import artoria.action.handler.ImportHandler;
import artoria.data.bean.BeanUtils;
import artoria.exception.ExceptionUtils;
import artoria.util.Assert;
import artoria.util.CollectionUtils;
import artoria.util.MapUtils;
import artoria.util.StringUtils;
import cn.hutool.poi.excel.ExcelReader;
import cn.hutool.poi.excel.ExcelUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStream;
import java.util.Date;
import java.util.List;
import java.util.Map;
import static artoria.common.constant.Numbers.*;
import static artoria.util.ObjectUtils.cast;
/**
* The abstract hutool small excel import action handler.
* This class only supports small amount of data in excel files.
* @param The import parameter type
* @param The type of data parsed
* @author Kahle
*/
public abstract class AbstractHutoolSmallExcelImportHandler
extends AbstractImportExportActionHandler
implements ImportHandler
{
private static Logger log = LoggerFactory.getLogger(AbstractHutoolSmallExcelImportHandler.class);
public AbstractHutoolSmallExcelImportHandler(String actionName) {
super(actionName);
}
protected InputStream parseFile(ExcelImportContext
context) {
Object fileData = context.getFileData();
Assert.notNull(fileData, "Parameter \"fileData\" must not null. ");
try {
if (fileData instanceof MultipartFile) {
MultipartFile file = (MultipartFile) fileData;
context.setFilename(file.getOriginalFilename());
context.setContentType(file.getContentType());
context.setFileSize(file.getSize());
return file.getInputStream();
}
else if (fileData instanceof File) {
File file = (File) fileData;
context.setFilename(file.getName());
return new FileInputStream(file);
}
else {
throw new IllegalArgumentException(
"The type of parameter \"fileData\" is not supported. "
);
}
}
catch (Exception e) {
throw ExceptionUtils.wrap(e);
}
}
/**
* Configuring the import context object.
* @param context The import context
*/
protected abstract void configContext(ExcelImportContext
context);
@Override
public List parseData(ImportContext context, Object cursor, Object rawData) {
// Transform context object.
ExcelImportContext
nowContext = cast(context);
// The data conversion.
Class exchangeClass = nowContext.getExchangeClass();
return BeanUtils.beanToBeanInList((List>) rawData, exchangeClass);
}
@Override
public ExcelImportContext buildContext(Object... arguments) {
// Create the context.
ExcelImportContext
context = createContext(ExcelImportContext.class, arguments);
context.setBeginTime(new Date());
context.setStatus(ONE);
// Parse file data.
Object fileData = context.getFileData();
Assert.notNull(fileData, "Parameter \"fileData\" must not null. ");
InputStream inputStream = parseFile(context);
// Configuring context object.
configContext(context);
// Check of context.
Assert.notBlank(context.getModule(), "Parameter \"module\" must not blank. ");
Class exchangeClass = context.getExchangeClass();
Assert.notNull(exchangeClass, "Parameter \"exchangeClass\" must not null. ");
// Default value processing.
if (context.getAsync() == null) { context.setAsync(false); }
if (StringUtils.isBlank(context.getResultMessage()) && context.getAsync()) {
context.setResultMessage("Please wait for a moment while the data is being imported! ");
}
// Read the data.
ExcelReader excelReader = ExcelUtil.getReader(inputStream);
if (MapUtils.isNotEmpty(context.getHeaderAliases())) {
excelReader.setHeaderAlias(context.getHeaderAliases());
}
List