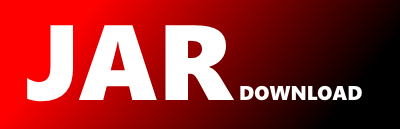
artoria.event.EventHolder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package artoria.event;
import artoria.convert.ConversionUtils;
import artoria.util.ArrayUtils;
import artoria.util.Assert;
import artoria.util.Current;
import artoria.util.ObjectUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import static artoria.common.Constants.THIRTY;
/**
* The event temporary management related tools.
* @deprecated The event tools based on ThreadLocal should not be provided,
* Because exception handling of ThreadLocal is a hassle (20220504).
* @author Kahle
*/
@Deprecated // TODO: Deletable
public class EventHolder {
private static final Logger log = LoggerFactory.getLogger(EventHolder.class);
private static final String EVENT_MAP_NAME = "event_map";
protected static Map getEventMap() {
Map eventMap = ObjectUtils.cast(Current.get(EVENT_MAP_NAME));
//Assert.notNull(eventMap, "The thread local container is not ready. ")
if (eventMap == null) {
eventMap = new HashMap(THIRTY);
Current.put(EVENT_MAP_NAME, eventMap);
}
return eventMap;
}
protected static void submit(Event event) {
Assert.notNull(event, "Parameter \"event\" must not null. ");
EventUtils.track(event.getCode(),
event.getTime(),
event.getPrincipalId(),
event.getProperties());
boolean managed = event.getManaged();
String alias = event.getAlias();
if (managed) { getEventMap().remove(alias); }
}
public static Event alias(String alias) {
return alias(alias, true);
}
public static Event alias(String alias, boolean managed) {
// In some cases, the same event can occur more than once,
// So aliases are more appropriate for event code.
// For example, the integral obtain event, it's possible to have more than one at a time.
alias = String.valueOf(alias);
Map eventMap = getEventMap();
Event event = eventMap.get(alias);
if (event == null) {
event = new Event(alias, managed);
if (managed) { eventMap.put(alias, event); }
}
return event;
}
public static Event aliasAndCode(String aliasAndCode) {
return alias(aliasAndCode).setCode(aliasAndCode);
}
public static Event aliasAndCode(String aliasAndCode, boolean managed) {
return alias(aliasAndCode, managed).setCode(aliasAndCode);
}
public static void submit(String alias) {
alias = String.valueOf(alias);
Event event = getEventMap().get(alias);
Assert.state(event != null,
"The event corresponding to the alias does not exist. ");
submit(event);
}
public static void cancel(String alias) {
alias = String.valueOf(alias);
getEventMap().remove(alias);
}
public static void clear() {
getEventMap().clear();
Current.remove(EVENT_MAP_NAME);
}
/**
* The convenient event object.
*/
public static class Event {
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy