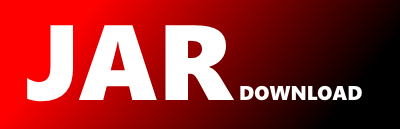
artoria.generator.JavaCodeGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package artoria.generator;
import artoria.data.bean.BeanUtils;
import artoria.exception.ExceptionUtils;
import artoria.generator.code.DatabaseTypeMapper;
import artoria.jdbc.ColumnMeta;
import artoria.jdbc.DatabaseClient;
import artoria.jdbc.TableMeta;
import artoria.renderer.TextRenderer;
import artoria.time.DateUtils;
import artoria.util.Assert;
import artoria.util.CollectionUtils;
import artoria.util.MapUtils;
import artoria.util.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.Serializable;
import java.sql.SQLException;
import java.util.*;
import static artoria.common.constant.Charsets.STR_UTF_8;
import static artoria.common.constant.Numbers.*;
import static artoria.common.constant.Symbols.*;
import static artoria.common.constant.TimePatterns.Y4MD2MI_HMS2CO_S3;
import static artoria.common.constant.Words.GET;
import static artoria.common.constant.Words.SET;
import static artoria.io.util.IOUtils.EOF;
/**
* Java code generator.
* @author Kahle
*/
@Deprecated
public class JavaCodeGenerator implements Serializable {
private static final String DEFAULT_XML_BEGIN_COVER_MARK
= "";
private static final String DEFAULT_XML_END_COVER_MARK
= "";
private static final String DEFAULT_JAVA_BEGIN_COVER_MARK
= "/* (Start) This will be covered, please do not modify. */";
private static final String DEFAULT_JAVA_END_COVER_MARK
= "/* (End) This will be covered, please do not modify. */";
private static final String DEFAULT_TEMPLATE_NAME_ARRAY
= "mapper_xml,mapper_java,entity_java,serviceImpl_java,service_java,controller_java,dto_java,vo_java";
private static final String JAVA_TYPE = "javaType";
private static final String SERVICEIMPL = "serviceimpl";
private static final String CONTROLLER = "controller";
private static final String SERVICE = "service";
private static final String MAPPER = "mapper";
private static final String ENTITY = "entity";
private static final String DTO = "dto";
private static final String VO = "vo";
private static final String JAVA = "java";
private static final String XML = "xml";
private static Logger log = LoggerFactory.getLogger(JavaCodeGenerator.class);
private Set removedTableNamePrefixes = new HashSet();
private Set reservedTables = new HashSet();
private Set excludedTables = new HashSet();
private DatabaseClient databaseClient;
private String templateCharset = STR_UTF_8;
private String outputCharset = STR_UTF_8;
private String baseTemplatePath = "classpath:templates/generator/java/default";
private String templateExtensionName = ".txt";
private String baseOutputPath;
private String basePackageName;
private TextRenderer textRenderer;
private Map attributes = new HashMap();
private Map creatorMap = new HashMap();
public JavaCodeGenerator() {
this.addAttribute("author", "artoria-extend");
this.addAttribute("date", DateUtils.format(Y4MD2MI_HMS2CO_S3));
}
public Set getRemovedTableNamePrefixes() {
return Collections.unmodifiableSet(removedTableNamePrefixes);
}
public JavaCodeGenerator addRemovedTableNamePrefixes(String... removedTableNamePrefixes) {
Assert.notEmpty(removedTableNamePrefixes
, "Parameter \"removedTableNamePrefixes\" must not empty. ");
Collections.addAll(this.removedTableNamePrefixes, removedTableNamePrefixes);
return this;
}
public Set getReservedTables() {
return Collections.unmodifiableSet(reservedTables);
}
public JavaCodeGenerator addReservedTables(String... reservedTables) {
Assert.notEmpty(reservedTables
, "Parameter \"reservedTables\" must not empty. ");
Collections.addAll(this.reservedTables, reservedTables);
return this;
}
public Set getExcludedTables() {
return Collections.unmodifiableSet(excludedTables);
}
public JavaCodeGenerator addExcludedTables(String... excludedTables) {
Assert.notEmpty(excludedTables
, "Parameter \"excludedTables\" must not empty. ");
Collections.addAll(this.excludedTables, excludedTables);
return this;
}
public DatabaseClient getDatabaseClient() {
return databaseClient;
}
public JavaCodeGenerator setDatabaseClient(DatabaseClient databaseClient) {
Assert.notNull(databaseClient
, "Parameter \"databaseClient\" must not null. ");
this.databaseClient = databaseClient;
return this;
}
public String getTemplateCharset() {
return templateCharset;
}
public JavaCodeGenerator setTemplateCharset(String templateCharset) {
Assert.notBlank(templateCharset
, "Parameter \"templateCharset\" must not blank. ");
this.templateCharset = templateCharset;
return this;
}
public String getOutputCharset() {
return outputCharset;
}
public JavaCodeGenerator setOutputCharset(String outputCharset) {
Assert.notBlank(outputCharset
, "Parameter \"outputCharset\" must not blank. ");
this.outputCharset = outputCharset;
return this;
}
public String getBaseTemplatePath() {
return baseTemplatePath;
}
public JavaCodeGenerator setBaseTemplatePath(String baseTemplatePath) {
Assert.notBlank(baseTemplatePath
, "Parameter \"baseTemplatePath\" must not blank. ");
this.baseTemplatePath = baseTemplatePath;
return this;
}
public String getTemplateExtensionName() {
return templateExtensionName;
}
public JavaCodeGenerator setTemplateExtensionName(String templateExtensionName) {
Assert.notBlank(templateExtensionName
, "Parameter \"templateExtensionName\" must not blank. ");
this.templateExtensionName = templateExtensionName;
return this;
}
public String getBaseOutputPath() {
return baseOutputPath;
}
public JavaCodeGenerator setBaseOutputPath(String baseOutputPath) {
Assert.notBlank(baseOutputPath
, "Parameter \"baseOutputPath\" must not blank. ");
this.baseOutputPath = baseOutputPath;
return this;
}
public String getBasePackageName() {
return basePackageName;
}
public JavaCodeGenerator setBasePackageName(String basePackageName) {
Assert.notBlank(basePackageName
, "Parameter \"basePackageName\" must not blank. ");
this.basePackageName = basePackageName;
return this;
}
public TextRenderer getTextRenderer() {
return textRenderer;
}
public JavaCodeGenerator setTextRenderer(TextRenderer textRenderer) {
Assert.notNull(textRenderer, "Parameter \"textRenderer\" must not null. ");
this.textRenderer = textRenderer;
return this;
}
public JavaCodeGenerator addAttribute(String name, Object value) {
attributes.put(name, value);
return this;
}
public JavaCodeGenerator addAttributes(Map data) {
attributes.putAll(data);
return this;
}
public JavaCodeGenerator removeAttribute(String name) {
attributes.remove(name);
return this;
}
public JavaCodeGenerator clearAttribute() {
attributes.clear();
return this;
}
public Map getAttributes() {
return Collections.unmodifiableMap(attributes);
}
public JavaCodeGenerator newCreator() {
this.newCreator(DEFAULT_TEMPLATE_NAME_ARRAY);
return this;
}
public JavaCodeGenerator newCreator(String templateNameArray) {
Assert.notBlank(templateNameArray
, "Parameter \"templateNameArray\" must not blank. ");
String[] split = templateNameArray.split(COMMA);
for (String templateName : split) {
if (StringUtils.isBlank(templateName)) { continue; }
this.newCreator(templateName, null);
}
return this;
}
public JavaCodeGenerator newCreator(String templateName, String businessPackageName) {
Assert.notBlank(templateName, "Parameter \"templateName\" must not blank. ");
JavaCodeCreator creator = new JavaCodeCreator();
boolean havePackageName = StringUtils.isNotBlank(businessPackageName);
if (templateName.toLowerCase().contains(MAPPER)) {
businessPackageName = havePackageName ? businessPackageName : ".persistence.mapper";
creator.setSkipExisted(false);
}
else if (templateName.toLowerCase().contains(SERVICEIMPL)) {
businessPackageName = havePackageName ? businessPackageName : ".service.impl";
creator.setSkipExisted(true);
}
else if (templateName.toLowerCase().contains(SERVICE)) {
businessPackageName = havePackageName ? businessPackageName : ".service";
creator.setSkipExisted(false);
}
else if (templateName.toLowerCase().contains(CONTROLLER)) {
businessPackageName = havePackageName ? businessPackageName : ".controller";
creator.setSkipExisted(true);
}
else if (templateName.toLowerCase().contains(ENTITY)) {
businessPackageName = havePackageName ? businessPackageName : ".persistence.entity";
creator.setSkipExisted(false);
}
else if (templateName.toLowerCase().contains(VO)) {
businessPackageName = havePackageName ? businessPackageName : ".pojo.vo";
creator.setSkipExisted(false);
}
else if (templateName.toLowerCase().contains(DTO)) {
businessPackageName = havePackageName ? businessPackageName : ".pojo.dto";
creator.setSkipExisted(false);
}
else {
businessPackageName = EMPTY_STRING;
creator.setSkipExisted(true);
}
if (templateName.toLowerCase().contains(XML)) {
creator.setBeginCoverMark(DEFAULT_XML_BEGIN_COVER_MARK);
creator.setEndCoverMark(DEFAULT_XML_END_COVER_MARK);
}
else if (templateName.toLowerCase().contains(JAVA)) {
creator.setBeginCoverMark(DEFAULT_JAVA_BEGIN_COVER_MARK);
creator.setEndCoverMark(DEFAULT_JAVA_END_COVER_MARK);
}
creator.setBusinessPackageName(businessPackageName);
creator.setTemplateName(templateName);
creatorMap.put(templateName, creator);
return this;
}
public JavaCodeGenerator removeCreator(String templateName) {
creatorMap.remove(templateName);
return this;
}
public JavaCodeGenerator clearCreator() {
creatorMap.clear();
return this;
}
public JavaCodeCreator getCreator(String templateName) {
return creatorMap.get(templateName);
}
public Map getCreatorMap() {
return Collections.unmodifiableMap(creatorMap);
}
protected Map tableMap(TableMeta tableMeta) {
if (tableMeta == null) { return null; }
Map result = BeanUtils.beanToMap(tableMeta);
// Get the column list and create a column map list.
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy