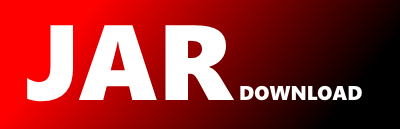
artoria.jdbc.DatabaseClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package artoria.jdbc;
import artoria.data.bean.BeanUtils;
import artoria.exception.ExceptionUtils;
import artoria.logging.Logger;
import artoria.logging.LoggerFactory;
import artoria.util.Assert;
import artoria.util.CloseUtils;
import artoria.util.StringUtils;
import javax.sql.DataSource;
import java.sql.*;
import java.util.*;
import static artoria.common.constant.Numbers.*;
import static artoria.common.constant.Symbols.COMMA;
/**
* Database client.
* @author Kahle
*/
@Deprecated
public class DatabaseClient {
private static final int DEFAULT_TRANSACTION_LEVEL = Connection.TRANSACTION_REPEATABLE_READ;
private static final Logger log = LoggerFactory.getLogger(DatabaseClient.class);
private final ThreadLocal threadConnection = new ThreadLocal();
private DataSource dataSource;
public DatabaseClient(DataSource dataSource) {
setDataSource(dataSource);
}
public DataSource getDataSource() {
return dataSource;
}
public void setDataSource(DataSource dataSource) {
Assert.notNull(dataSource, "Parameter \"dataSource\" must not null. ");
this.dataSource = dataSource;
}
public List getTableMetaList() throws SQLException {
List tableMetaList = new ArrayList();
String[] types = new String[]{"TABLE"};
ResultSet tableResultSet = null;
Connection connection = null;
try {
connection = getConnection();
DatabaseMetaData databaseMetaData = connection.getMetaData();
tableResultSet = databaseMetaData.getTables((String) null, (String) null, (String) null, types);
while (tableResultSet.next()) {
Map columnMap = new HashMap(TWENTY);
String tableName = tableResultSet.getString((String) "TABLE_NAME");
String remarks = tableResultSet.getString((String) "REMARKS");
TableMeta tableMeta = new TableMeta();
tableMetaList.add(tableMeta);
tableMeta.setName(tableName);
tableMeta.setRemarks(remarks);
fillColumnMeta(databaseMetaData, tableMeta, columnMap);
fillClassName(connection, tableName, columnMap);
}
return tableMetaList;
}
finally {
CloseUtils.closeQuietly(tableResultSet);
closeConnection(connection);
}
}
public boolean transaction(JdbcAtom atom) throws SQLException {
return transaction(atom, DEFAULT_TRANSACTION_LEVEL);
}
public boolean transaction(JdbcAtom atom, int transactionLevel) throws SQLException {
Connection connection = threadConnection.get();
// Nested transaction support.
if (connection != null) {
if (connection.getTransactionIsolation() < transactionLevel) {
connection.setTransactionIsolation(transactionLevel);
}
boolean result = atom.run();
if (result) {
return true;
}
// Important: can not return false
throw new JdbcException("Notice the outer transaction that the nested transaction return false");
}
// Normal transaction support.
Boolean autoCommit = null;
try {
connection = getConnection();
autoCommit = connection.getAutoCommit();
threadConnection.set(connection);
connection.setTransactionIsolation(transactionLevel);
connection.setAutoCommit(false);
boolean result = atom.run();
if (result) {
connection.commit();
}
else {
connection.rollback();
}
return result;
}
catch (Exception e) {
rollbackTransaction(connection);
throw ExceptionUtils.wrap(e);
}
finally {
closeTransaction(connection, autoCommit);
}
}
public List executeQuery(Class clazz, String sql, Object... params) throws SQLException {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy