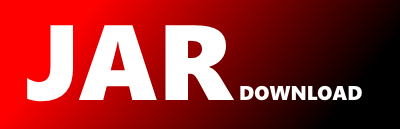
artoria.jdbc.mybatisplus.handler.AbstractMetaObjectHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package artoria.jdbc.mybatisplus.handler;
import artoria.exception.ExceptionUtils;
import artoria.util.Assert;
import artoria.util.ObjectUtils;
import com.baomidou.mybatisplus.core.handlers.MetaObjectHandler;
import org.apache.ibatis.reflection.MetaObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Date;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentHashMap;
import static artoria.convert.ConversionUtils.convert;
/**
* The abstract meta object field fill handler.
* @author Kahle
*/
public abstract class AbstractMetaObjectHandler implements MetaObjectHandler {
private static final Logger log = LoggerFactory.getLogger(AbstractMetaObjectHandler.class);
private final Map> commands = new ConcurrentHashMap>();
private final Map configs = new ConcurrentHashMap();
protected Map> getCommands() { return commands; }
protected Map getConfigs() { return configs; }
/**
* Register a command.
* @param command The name of the command that is registered
* @param callable The command logic that is registered
*/
protected void registerCommand(String command, Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy