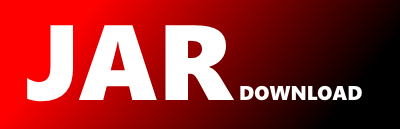
artoria.polyglot.GraalPolyglotProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package artoria.polyglot;
import artoria.data.Dict;
import artoria.data.bean.BeanUtils;
import artoria.util.Assert;
import artoria.util.MapUtils;
import org.graalvm.polyglot.Context;
import org.graalvm.polyglot.HostAccess;
import org.graalvm.polyglot.Source;
import org.graalvm.polyglot.Value;
import java.util.Map;
import java.util.function.Predicate;
import static artoria.util.ObjectUtils.cast;
/**
* The polyglot execution provider base on graalvm-js.
* @see ScriptEngine Implementation
* @see Migration Guide from Nashorn to GraalVM JavaScript
* @author Kahle
*/
public class GraalPolyglotProvider implements PolyglotProvider {
/**
* Build graalvm context.
* @param name The language name
* @param config The config
* @return The graalvm context
*/
protected Context buildContext(String name, Object config) {
Assert.notBlank(name, "Parameter \"name\" must not blank. ");
Dict configDict = Dict.of(BeanUtils.beanToMap(config));
// Context builder.
Context.Builder builder = Context.newBuilder();
// Allow host access.
builder.allowHostAccess(configDict.get("allowHostAccess", HostAccess.class, HostAccess.ALL));
// Allows access to all Java classes.
//builder.allowHostClassLookup(className -> true)
Predicate predicate = cast(configDict.get("allowHostClassLookup", Predicate.class));
builder.allowHostClassLookup(predicate != null ? predicate : new Predicate() {
@Override
public boolean test(String s) {
return true;
}
});
// Options.
Map options = cast(configDict.get("options", Map.class));
if (MapUtils.isNotEmpty(options)) {
builder.options(options);
}
// Build.
return builder.build();
}
@Override
public Object eval(String name, Object source, Object config, Object data) {
Assert.notNull(source, "Parameter \"source\" must not null. ");
Assert.notBlank(name, "Parameter \"name\" must not blank. ");
Context context = null;
try {
// Build context.
if (data instanceof Context) { context = (Context) data; }
else {
context = buildContext(name, config);
Value bindings = context.getBindings(name);
Dict dict = Dict.of(BeanUtils.beanToMap(data));
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy