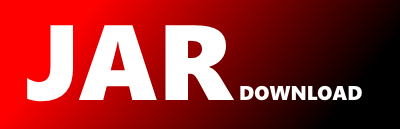
artoria.serialize.support.KryoSerializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package artoria.serialize.support;
import artoria.core.Serializer;
import artoria.util.Assert;
import com.esotericsoftware.kryo.Kryo;
import com.esotericsoftware.kryo.io.Input;
import com.esotericsoftware.kryo.io.Output;
import com.esotericsoftware.kryo.pool.KryoCallback;
import com.esotericsoftware.kryo.pool.KryoPool;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
/**
* Kryo serializer.
* @author Kahle
*/
@Deprecated
public class KryoSerializer implements Serializer {
private final KryoPool kryoPool;
public KryoSerializer(KryoPool kryoPool) {
Assert.notNull(kryoPool, "Parameter \"kryoPool\" must not null. ");
this.kryoPool = kryoPool;
}
@Override
public Object serialize(final Object object) {
Assert.notNull(object, "Parameter \"object\" must not null. ");
return kryoPool.run(new KryoCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy