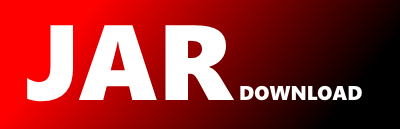
artoria.spring.config.hystrix.RequestContextHystrixConcurrencyStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package artoria.spring.config.hystrix;
import com.netflix.hystrix.HystrixThreadPoolKey;
import com.netflix.hystrix.strategy.concurrency.HystrixConcurrencyStrategy;
import com.netflix.hystrix.strategy.concurrency.HystrixRequestVariable;
import com.netflix.hystrix.strategy.concurrency.HystrixRequestVariableLifecycle;
import com.netflix.hystrix.strategy.properties.HystrixProperty;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.context.request.RequestAttributes;
import org.springframework.web.context.request.RequestContextHolder;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.Callable;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
/**
* Spring request context holder hystrix concurrency strategy.
* @author Kahle
*/
public class RequestContextHystrixConcurrencyStrategy extends HystrixConcurrencyStrategy {
private static final Logger log = LoggerFactory.getLogger(RequestContextHystrixConcurrencyStrategy.class);
private final HystrixConcurrencyStrategy existingConcurrencyStrategy;
public RequestContextHystrixConcurrencyStrategy(HystrixConcurrencyStrategy existingConcurrencyStrategy) {
this.existingConcurrencyStrategy = existingConcurrencyStrategy;
}
@Override
public ThreadPoolExecutor getThreadPool(HystrixThreadPoolKey threadPoolKey,
HystrixProperty corePoolSize,
HystrixProperty maximumPoolSize,
HystrixProperty keepAliveTime,
TimeUnit unit,
BlockingQueue workQueue) {
return existingConcurrencyStrategy == null
? super.getThreadPool(threadPoolKey, corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue)
: existingConcurrencyStrategy.getThreadPool(threadPoolKey, corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue);
}
@Override
public BlockingQueue getBlockingQueue(int maxQueueSize) {
return existingConcurrencyStrategy == null
? super.getBlockingQueue(maxQueueSize)
: existingConcurrencyStrategy.getBlockingQueue(maxQueueSize);
}
@Override
public Callable wrapCallable(Callable callable) {
RequestAttributes requestAttributes = RequestContextHolder.getRequestAttributes();
Callable wrappedCallable = new WrappedCallable(callable, requestAttributes);
return existingConcurrencyStrategy == null
? super.wrapCallable(wrappedCallable)
: existingConcurrencyStrategy.wrapCallable(wrappedCallable);
}
@Override
public HystrixRequestVariable getRequestVariable(HystrixRequestVariableLifecycle rv) {
return existingConcurrencyStrategy == null
? super.getRequestVariable(rv)
: existingConcurrencyStrategy.getRequestVariable(rv);
}
private static class WrappedCallable implements Callable {
private final RequestAttributes requestAttributes;
private final Callable delegate;
WrappedCallable(Callable delegate, RequestAttributes requestAttributes) {
this.requestAttributes = requestAttributes;
this.delegate = delegate;
}
@Override
public T call() throws Exception {
try {
RequestContextHolder.setRequestAttributes(requestAttributes);
return delegate.call();
}
finally {
RequestContextHolder.resetRequestAttributes();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy