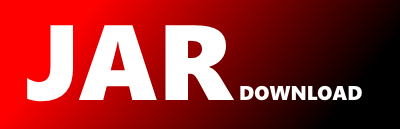
artoria.util.PagingUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package artoria.util;
import artoria.common.PageResult;
import artoria.common.Paging;
import artoria.data.bean.BeanUtils;
import com.github.pagehelper.Page;
import com.github.pagehelper.PageHelper;
import java.util.List;
/**
* Paging tools.
* @author Kahle
*/
public class PagingUtils {
public static void startPage(int pageNum, int pageSize) {
PagingUtils.startPage(new Paging(pageNum, pageSize));
}
public static void startPage(Paging paging) {
PagingUtils.startPage(paging, true, null);
}
public static void startPage(Paging paging, boolean doCount) {
PagingUtils.startPage(paging, doCount, null);
}
public static void startPage(Paging paging, String orderBy) {
PagingUtils.startPage(paging, true, orderBy);
}
public static void startPage(Paging paging, boolean doCount, String orderBy) {
if (paging == null) { return; }
Integer pageSize = paging.getPageSize();
Integer pageNum = paging.getPageNum();
Assert.notNull(pageSize, "Parameter \"pageSize\" must not null. ");
Assert.notNull(pageNum, "Parameter \"pageNum\" must not null. ");
PageHelper.startPage(pageNum, pageSize, doCount);
if (StringUtils.isNotBlank(orderBy)) {
PageHelper.orderBy(orderBy);
}
}
public static PageResult> handleResult(List data) {
if (data == null) {
return new PageResult>();
}
if (!(data instanceof Page)) {
return new PageResult>(data);
}
Page page = (Page) data;
PageResult> result = new PageResult>();
result.setPageNum(page.getPageNum());
result.setPageSize(page.getPageSize());
result.setPageCount(page.getPages());
result.setTotal(page.getTotal());
result.setData(data);
return result;
}
public static PageResult> handleResult(List data, Class clazz) {
if (data == null) {
return new PageResult>();
}
List list = BeanUtils.beanToBeanInList(data, clazz);
if (!(data instanceof Page)) {
return new PageResult>(list);
}
Page page = (Page) data;
PageResult> result = new PageResult>();
result.setPageNum(page.getPageNum());
result.setPageSize(page.getPageSize());
result.setPageCount(page.getPages());
result.setTotal(page.getTotal());
result.setData(list);
return result;
}
public static PageResult> handleResult(PageResult> data, Class clazz) {
if (data == null) {
return new PageResult>();
}
PageResult> result = new PageResult>();
result.setPageNum(data.getPageNum());
result.setPageSize(data.getPageSize());
result.setPageCount(data.getPageCount());
result.setTotal(data.getTotal());
List fList = data.getData();
List tList = BeanUtils.beanToBeanInList(fList, clazz);
result.setData(tList);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy