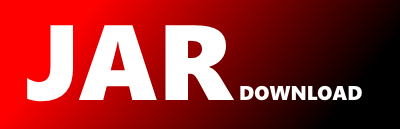
templates.generator.java.custom2.mapper_java.vm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artoria-extend Show documentation
Show all versions of artoria-extend Show documentation
Artoria is a java technology framework based on the facade pattern.
The newest version!
package ${table.mapperPackageName};
import ${table.entityPackageName}.${table.entityClassName};
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import java.util.List;
/**
* ${table.mapperClassName}.
* @author $!{author}
* @date $!{date}
*/
@Mapper
public interface ${table.mapperClassName} {
/* (Start) This will be covered, please do not modify. */
/**
* Insert.
* @param record The object to insert
* @return Number of rows effected
*/
int insert(${table.entityClassName} record);
/**
* Insert batch.
* @param recordList A list of records to insert
* @return Number of rows effected
*/
int insertBatch(@Param("recordList") List<${table.entityClassName}> recordList);
/**
* Insert selective.
* @param record The object to insert
* @return Number of rows effected
*/
int insertSelective(${table.entityClassName} record);
/**
* Delete by primary key.
#foreach(${column} in ${table.columnList})
#if($column.primaryKey)
* @param ${column.fieldName} Primary key
#end#end
* @param updaterId Current operator
* @return Number of rows effected
*/
int deleteByPrimaryKey(#set($temp = 0)
#foreach(${column} in ${table.columnList})
#if($column.primaryKey)
#if($temp > 0), #end#set($temp = $temp + 1)#*
*#@Param("${column.fieldName}") ${column.javaType} ${column.fieldName}#end
#end, @Param("updaterId") String updaterId);
/**
* Delete by primary key list.
#foreach(${column} in ${table.columnList})
#if($column.primaryKey)
* @param ${column.fieldName}List Primary key list
#end#end
* @param updaterId Current operator
* @return Number of rows effected
*/
int deleteByPrimaryKeyList(#set($temp = 0)
#foreach(${column} in ${table.columnList})
#if($column.primaryKey)
#if($temp > 0), #end#set($temp = $temp + 1)#*
*#@Param("${column.fieldName}List") List<${column.javaType}> ${column.fieldName}List#end
#end, @Param("updaterId") String updaterId);
/**
* Delete selective.
* @param record Delete conditions
* @return Number of rows effected
*/
int deleteSelective(${table.entityClassName} record);
/**
* Update by primary key.
* @param record Content to be updated
* @return Number of rows effected
*/
int updateByPrimaryKey(${table.entityClassName} record);
/**
* Update by primary key selective.
* @param record Content to be updated
* @return Number of rows effected
*/
int updateByPrimaryKeySelective(${table.entityClassName} record);
/**
* Conditional counting.
* @param record Query condition
* @return Count result
*/
int countSelective(${table.entityClassName} record);
/**
* Query by primary key.
#foreach(${column} in ${table.columnList})
#if($column.primaryKey)
* @param ${column.fieldName} Primary key
#end#end
* @return Query result
*/
${table.entityClassName} queryByPrimaryKey(#set($temp = 0)
#foreach(${column} in ${table.columnList})
#if($column.primaryKey)
#if($temp > 0), #end#set($temp = $temp + 1)#*
*#@Param("${column.fieldName}") ${column.javaType} ${column.fieldName}#end
#end);
/**
* Query by primary key list.
#foreach(${column} in ${table.columnList})
#if($column.primaryKey)
* @param ${column.fieldName}List Primary key list
#end#end
* @return Query result list
*/
List<${table.entityClassName}> queryByPrimaryKeyList(#set($temp = 0)
#foreach(${column} in ${table.columnList})
#if($column.primaryKey)
#if($temp > 0), #end#set($temp = $temp + 1)#*
*#@Param("${column.fieldName}List") List<${column.javaType}> ${column.fieldName}List#end
#end);
/**
* Find one.
* @param record Query condition
* @return Query result
*/
${table.entityClassName} findOne(${table.entityClassName} record);
/**
* Query selective.
* @param record Query condition
* @return Query result list
*/
List<${table.entityClassName}> querySelective(${table.entityClassName} record);
/* (End) This will be covered, please do not modify. */
/* Generated by artoria-extend in ${buildTime}. */
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy