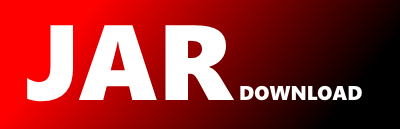
com.github.kaiwinter.androidremotenotifications.model.impl.VersionCodePolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of android-remote-notifications Show documentation
Show all versions of android-remote-notifications Show documentation
Pulls notifications from a remote JSON file and shows them in your app.
package com.github.kaiwinter.androidremotenotifications.model.impl;
import java.util.Collection;
/**
* Defines the app version codes on which a notification should be shown. If the current app install doesn't match this
* {@link VersionCodePolicy} the notification will not be shown.
* There are three ways to define on which versions of your app the notification should be shown: onAllBefore,
* onAllAfter and onSpecific. You can also mix them but have to keep them sane. Only one of the previous named
* parameters have to match to let the notification show. They are evaluated in the previous named order.
*/
public final class VersionCodePolicy {
/**
* The notification will be shown on all versions < onAllBefore
.
*/
private Integer onAllBefore;
/**
* The notification will be shown on all versions > onAllAfter
.
*/
private Integer onAllAfter;
/**
* The notification will be shown on all versions which are in onSpecific
.
*/
private Collection onSpecific;
/**
* Checks if one of onAllAfter
, onAllBefore
or onSpecific
matches the given
* versionCode
. If one matches true
is returned, else false
.
*
* @param appVersionCode The version code to match against this {@link VersionCodePolicy}.
* @return true
if the notification should be shown, else false
.
*/
public boolean hasBeShownForThisVersion(int appVersionCode) {
if (onAllAfter != null) {
if (appVersionCode > onAllAfter) {
return true;
}
}
if (onAllBefore != null) {
if (appVersionCode < onAllBefore) {
return true;
}
}
if (onSpecific != null) {
if (onSpecific.contains(appVersionCode)) {
return true;
}
}
return false;
}
/**
* @return the versionCode before which the notification should be shown
*/
public Integer getOnAllBefore() {
return onAllBefore;
}
/**
* Sets the versionCode before which the notification should be shown.
*
* @param onAllBefore the versionCode before which the notification should be shown
*/
public void setOnAllBefore(Integer onAllBefore) {
this.onAllBefore = onAllBefore;
}
/**
* @return the versionCode after which the notification should be shown
*/
public Integer getOnAllAfter() {
return onAllAfter;
}
/**
* Set the versionCode after which the notification should be shown.
*
* @param onAllAfter the versionCode after which the notification should be shown
*/
public void setOnAllAfter(Integer onAllAfter) {
this.onAllAfter = onAllAfter;
}
/**
* @return the specific app versionCodes on which the notification should be shown
*/
public Collection getOnSpecific() {
return onSpecific;
}
/**
* Sets specific app versionCodes on which the notification should be shown.
*
* @param onSpecific the app versionCodes on which the notification should be shown
*/
public void setOnSpecific(Collection onSpecific) {
this.onSpecific = onSpecific;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy