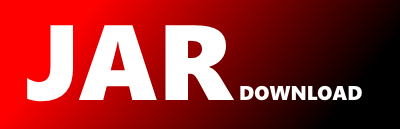
com.github.kaizen4j.algorithm.encrypt.RsaSignature Maven / Gradle / Ivy
package com.github.kaizen4j.algorithm.encrypt;
import static java.nio.charset.StandardCharsets.UTF_8;
import java.security.InvalidKeyException;
import java.security.KeyFactory;
import java.security.NoSuchAlgorithmException;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.Signature;
import java.security.SignatureException;
import java.security.spec.InvalidKeySpecException;
import java.security.spec.MGF1ParameterSpec;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import javax.crypto.Cipher;
import javax.crypto.spec.OAEPParameterSpec;
import javax.crypto.spec.PSource.PSpecified;
import org.apache.commons.codec.binary.Base64;
/**
* 基于 RSA 加密算法的数字签名类
*
* @author liuguowen
*/
public final class RsaSignature {
private static final String RSA_DECRYPTION = "RSA";
private static final String SHA256_WITH_RSA = "SHA256withRSA";
private static final String RSA_ECB_OAEP_PADDING = "RSA/ECB/OAEPWithSHA-256AndMGF1Padding";
private static final MGF1ParameterSpec SHA256 = MGF1ParameterSpec.SHA256;
private static final String MGF1 = "MGF1";
private RsaSignature() {
}
/**
* 签名方法
*
* @param data 待签名字符串
* @param privateKeyStr 私钥字符串
* @return 签名后的字节数组
*/
public static byte[] sign(String data, String privateKeyStr) {
try {
PrivateKey privatekey = toPrivateKey(privateKeyStr);
Signature signature = Signature.getInstance(SHA256_WITH_RSA);
signature.initSign(privatekey);
signature.update(data.getBytes(UTF_8));
return signature.sign();
} catch (NoSuchAlgorithmException | InvalidKeyException | SignatureException e) {
throw new RuntimeException(e);
}
}
/**
* 校验方法
*
* @param data 待校验字符串
* @param signData 已签名的字节数组
* @param publicKeyStr 公钥
* @return 校验通过返回 true,失败返回 false
*/
public static boolean verify(String data, byte[] signData, String publicKeyStr) {
try {
PublicKey publicKey = toPublicKey(publicKeyStr);
Signature signature = Signature.getInstance(SHA256_WITH_RSA);
signature.initVerify(publicKey);
signature.update(data.getBytes(UTF_8));
return signature.verify(signData);
} catch (NoSuchAlgorithmException | InvalidKeyException | SignatureException e) {
throw new RuntimeException(e);
}
}
/**
* 加密数据
*
* @return 加密后的字节数组
*/
@SuppressWarnings("all")
public static byte[] encrypt(byte[] data, String publicKey) {
try {
Cipher cipher = Cipher.getInstance(RSA_ECB_OAEP_PADDING);
OAEPParameterSpec oaepParameterSpec = new OAEPParameterSpec(SHA256.getDigestAlgorithm(), MGF1, SHA256,
PSpecified.DEFAULT);
cipher.init(Cipher.ENCRYPT_MODE, RsaSignature.toPublicKey(publicKey), oaepParameterSpec);
return cipher.doFinal(data);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* 解密数据
*
* @return 解密后的原始字节数组
*/
@SuppressWarnings("all")
public static byte[] decrypt(byte[] data, String privateKey) {
try {
Cipher cipher = Cipher.getInstance(RSA_ECB_OAEP_PADDING);
OAEPParameterSpec oaepParameterSpec = new OAEPParameterSpec(SHA256.getDigestAlgorithm(), MGF1, SHA256,
PSpecified.DEFAULT);
cipher.init(Cipher.DECRYPT_MODE, RsaSignature.toPrivateKey(privateKey), oaepParameterSpec);
return cipher.doFinal(data);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* 转换字符串到 PublicKey 对象
*
* @return PublicKey 对象
*/
public static PublicKey toPublicKey(String key) {
try {
byte[] keyBytes = Base64.decodeBase64(key);
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(keyBytes);
KeyFactory keyFactory = KeyFactory.getInstance(RSA_DECRYPTION);
return keyFactory.generatePublic(keySpec);
} catch (InvalidKeySpecException | NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
}
/**
* 转换字符串到 PrivateKey 对象
*
* @return PrivateKey 对象
*/
public static PrivateKey toPrivateKey(String key) {
try {
byte[] keyBytes = Base64.decodeBase64(key);
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(keyBytes);
KeyFactory keyFactory = KeyFactory.getInstance(RSA_DECRYPTION);
return keyFactory.generatePrivate(keySpec);
} catch (InvalidKeySpecException | NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy