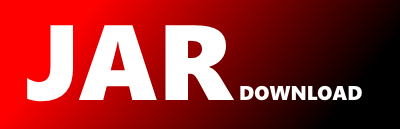
com.github.keub.maven.plugin.utils.FileUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of remote-resources-maven-plugin Show documentation
Show all versions of remote-resources-maven-plugin Show documentation
Copy files from a remote location to a path relative to the project in which the plugin is configured.
package com.github.keub.maven.plugin.utils;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
public class FileUtils {
/**
*
* copy reader into writer
*
*
* @param reader
* @param writer
* @throws IOException
*/
public static void writeFile(BufferedInputStream reader,
BufferedOutputStream writer) throws IOException {
byte[] buff = new byte[8192];
int numChars;
while ((numChars = reader.read(buff, 0, buff.length)) != -1) {
writer.write(buff, 0, numChars);
}
}
/**
*
* create target folders file if doesnt exist
*
*
* @param absoluteFile
* @throws FileNotFoundException
*/
public static void createIntermediateFolders(String absoluteFile)
throws FileNotFoundException {
if (absoluteFile == null) {
throw new FileNotFoundException("'" + absoluteFile
+ "' is not valid");
}
// normalize the path
String normalizedAbsoluteFile = normalizePath(absoluteFile);
int endIndex = normalizedAbsoluteFile.endsWith(PathUtils.SLASH) ? normalizedAbsoluteFile
.length() : normalizedAbsoluteFile.lastIndexOf(PathUtils.SLASH);
String filePath = normalizedAbsoluteFile.substring(0, endIndex);
new File(filePath).mkdirs();
}
/**
*
* standardization of a path by replacing the slash and backslash by a file
* separator
*
*
* @param path
* the path to normalize
* @return normalized string
*/
public static String normalizePath(String path) {
String retval = path;
retval = retval.replace(PathUtils.MULTIPLE_SLASH, PathUtils.SLASH);
retval = retval.replace(PathUtils.BACKSLASH, PathUtils.SLASH);
return retval;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy