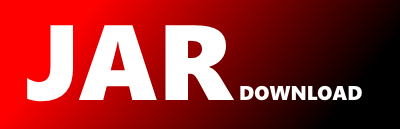
com.kidsoncoffee.cheesecakes.runner.ScenarioRunnerCreator Maven / Gradle / Ivy
package com.kidsoncoffee.cheesecakes.runner;
import com.google.inject.Inject;
import com.kidsoncoffee.cheesecakes.Example;
import com.kidsoncoffee.cheesecakes.runner.domain.ExampleRunner;
import com.kidsoncoffee.cheesecakes.runner.domain.ScenarioRunner;
import com.kidsoncoffee.cheesecakes.runner.example.ExamplesLoader;
import org.junit.runners.model.InitializationError;
import org.junit.runners.model.TestClass;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Creates all {@link ScenarioRunner}s from a test class.
*
* @author fernando.chovich
* @since 1.0
*/
public class ScenarioRunnerCreator {
/** The example loaders. */
private final Set exampleLoaders;
/**
* Constructs a {@link ScenarioRunnerCreator} with the parameters.
*
* @param exampleLoaders The example loaders.
*/
@Inject
public ScenarioRunnerCreator(final Set exampleLoaders) {
this.exampleLoaders = exampleLoaders;
}
/**
* Returns all {@link ScenarioRunner}s for the {@link TestClass}. Loads examples from all
* registered {@link ExamplesLoader} and then group by the scenario method name and create {@link
* org.junit.runner.Runner}s following the hierarchy, in which a scenario may have one or more
* examples.
*
* @param testClass The test class.
* @return The {@link ScenarioRunner}s for the {@link TestClass} parameter.
* @throws InitializationError If an error occurs while initializing the {@link ScenarioRunner} or
* {@link ExampleRunner}.
*/
public List create(final TestClass testClass) throws InitializationError {
final Map> examplesByScenario =
this.exampleLoaders.stream()
.map(examplesLoader -> examplesLoader.load(testClass.getJavaClass()))
.flatMap(Collection::stream)
.collect(Collectors.groupingBy(Example.Builder::getScenarioMethodName));
final List scenarioRunners = new ArrayList<>();
for (final String scenario : examplesByScenario.keySet()) {
final List examples = examplesByScenario.get(scenario);
final List exampleRunners = new ArrayList<>();
for (Example.Builder example : examples) {
exampleRunners.add(new ExampleRunner(testClass.getJavaClass(), example));
}
scenarioRunners.add(new ScenarioRunner(testClass.getJavaClass(), scenario, exampleRunners));
}
return Collections.unmodifiableList(scenarioRunners);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy