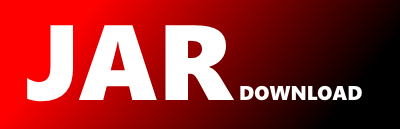
pro.jk.ejoker.common.system.enhance.EachUtilx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ejoker-common Show documentation
Show all versions of ejoker-common Show documentation
EJoker is a CQRS + EventSourcing framwork
package pro.jk.ejoker.common.system.enhance;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import pro.jk.ejoker.common.system.functional.IFunction1;
import pro.jk.ejoker.common.system.functional.IFunction2;
import pro.jk.ejoker.common.system.functional.IVoidFunction1;
import pro.jk.ejoker.common.system.functional.IVoidFunction2;
/**
* 在jdk1.8之后集合接口上都已经准备了 forEach方法了,大可用集合接口上的forEach方法
* 而且java的stream/parallelStream越来越成熟和好用了。
* 如果使用quasar协程,那么这个工具可以提供更友好的quasar织入能力
* @author kimffy
*
*/
public class EachUtilx {
/**
* 对每一个项进行vf处理
* @param
* @param
* @param targetMap
* @param vf
*/
public static void loop(Map targetMap, IVoidFunction2 vf) {
if(null == targetMap || targetMap.isEmpty())
return;
Set> entrySet = targetMap.entrySet();
for(Entry entry : entrySet)
vf.trigger(entry.getKey(), entry.getValue());
}
/**
* @see #loop(Map, IVoidFunction2)
* @param
* @param
* @param targetMap
* @param vf
* @deprecated 请改用loop系列方法
*/
public static void forEach(Map targetMap, IVoidFunction2 vf) {
loop(targetMap, vf);
}
/**
* 对每一个项进行vf处理
* @param
* @param target
* @param vf
*/
public static void loop(Collection target, IVoidFunction1 vf) {
if(null == target || target.isEmpty())
return;
for(V item : target)
vf.trigger(item);
}
/**
* @see #loop(Collection, IVoidFunction1)
* @param
* @param target
* @param vf
* @deprecated 请改用loop系列方法
*/
public static void forEach(Collection target, IVoidFunction1 vf) {
loop(target, vf);
}
/**
* 对每一个项进行vf处理
* @param
* @param target
* @param vf
*/
public static void loop(V[] target, IVoidFunction1 vf) {
if(null == target || 0 == target.length)
return;
for(int i = 0; i< target.length; i++)
vf.trigger(target[i]);
}
/**
* @see #loop(V[], IVoidFunction1)
* @param
* @param target
* @param vf
* @deprecated 请改用loop系列方法
*/
public static void forEach(V[] target, IVoidFunction1 vf) {
loop(target, vf);
}
/**
* 对每一个项进行vf处理
* @param
* @param target
* @param vf 第一个参当前迭代到的元素,第二个参数是元素的序号
*/
public static void loop(V[] target, IVoidFunction2 vf) {
if(null == target || 0 == target.length)
return;
for(int i = 0; i< target.length; i++)
vf.trigger(target[i], i);
}
/**
* @see #loop(V[], IVoidFunction2)
* @param
* @param target
* @param vf
* @deprecated 请改用loop系列方法
*/
public static void forEach(V[] target, IVoidFunction2 vf) {
loop(target, vf);
}
/**
* 1. 对每一个项进行vf处理
* 2. 剔除符合条件的目标项
* @param
* @param
* @param targetMap
* @param vf
* @param prediction
*/
public static void loopAndEliminate(Map targetMap, IVoidFunction2 vf, IFunction2 prediction) {
if(null == targetMap || targetMap.isEmpty())
return;
Iterator> iterator = targetMap.entrySet().iterator();
if(null != vf)
while(iterator.hasNext()) {
Entry current = iterator.next();
K key = current.getKey();
V value = current.getValue();
vf.trigger(key, value);
if(prediction.trigger(key, value))
iterator.remove();
}
else
while(iterator.hasNext()) {
Entry current = iterator.next();
if(prediction.trigger(current.getKey(), current.getValue()))
iterator.remove();
}
}
/**
* @see #loopAndEliminate(Map, IVoidFunction2, IFunction2)
* @param
* @param
* @param targetMap
* @param vf
* @param prediction
* @deprecated 请改用loop系列方法
*/
public static void forEachAndEliminate(Map targetMap, IVoidFunction2 vf, IFunction2 prediction) {
loopAndEliminate(targetMap, vf, prediction);
}
/**
* 1. 对每一个项进行vf处理
* 2. 剔除符合条件的目标项
* @param
* @param targetList
* @param vf
* @param prediction
*/
public static void loopAndEliminate(Collection targetList, IVoidFunction1 vf, IFunction1 prediction) {
if(null == targetList || targetList.isEmpty())
return;
Iterator iterator = targetList.iterator();
if(null != vf)
while(iterator.hasNext()) {
V current = iterator.next();
vf.trigger(current);
if(prediction.trigger(current))
iterator.remove();
}
else
while(iterator.hasNext()) {
if(prediction.trigger(iterator.next()))
iterator.remove();
}
}
/**
* @see #loopAndEliminate(Collection, IVoidFunction1, IFunction1)
* @param
* @param targetList
* @param vf
* @param prediction
* @deprecated 请改用loop系列方法
*/
public static void forEachAndEliminate(Collection targetList, IVoidFunction1 vf, IFunction1 prediction) {
loopAndEliminate(targetList, vf, prediction);
}
/**
* 剔除符合条件的目标项
* @param
* @param
* @param targetMap
* @param prediction
*/
public static void eliminate(Map targetMap, IFunction2 prediction) {
if(null == targetMap || targetMap.isEmpty())
return;
Iterator> iterator = targetMap.entrySet().iterator();
while(iterator.hasNext()) {
Entry current = iterator.next();
if(prediction.trigger(current.getKey(), current.getValue()))
iterator.remove();
}
}
/**
* @see #eliminate(Map, IFunction2)
* @param
* @param
* @param targetMap
* @param prediction
* @deprecated
*/
public static void forEachAndEliminate(Map targetMap, IFunction2 prediction) {
eliminate(targetMap, prediction);
}
/**
* 剔除符合条件的目标项
* @param
* @param targetList
* @param prediction
*/
public static void eliminate(Collection targetList, IFunction1 prediction) {
if(null == targetList || targetList.isEmpty())
return;
Iterator iterator = targetList.iterator();
while(iterator.hasNext()) {
if(prediction.trigger(iterator.next()))
iterator.remove();
}
}
/**
* @see #eliminate(Collection, IFunction1)
* @param
* @param targetList
* @param prediction
* @deprecated
*/
public static void forEachAndEliminate(Collection targetList, IFunction1 prediction) {
eliminate(targetList, prediction);
}
/**
* 在数组中选出符合条件的元素,并把结果组装成list
* @param
* @param target
* @param prediction
* @return 返回是一个LinkedList实例
*/
public static List select(V[] target, IFunction1 prediction) {
if(null == target || 0 == target.length)
return new ArrayList<>();
List resList = new LinkedList<>(); // Why use LinkedList? Cause we just consider the write speed.
for(int i = 0; i< target.length; i++)
if(prediction.trigger(target[i]))
resList.add(target[i]);
return resList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy