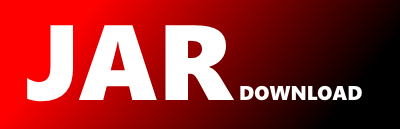
com.github.kittinunf.fuel.reactor.Reactor.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fuel-reactor Show documentation
Show all versions of fuel-reactor Show documentation
The easiest HTTP networking library in Kotlin/Android
package com.github.kittinunf.fuel.reactor
import com.github.kittinunf.fuel.core.Deserializable
import com.github.kittinunf.fuel.core.FuelError
import com.github.kittinunf.fuel.core.Request
import com.github.kittinunf.fuel.core.Response
import com.github.kittinunf.fuel.core.deserializers.ByteArrayDeserializer
import com.github.kittinunf.fuel.core.deserializers.EmptyDeserializer
import com.github.kittinunf.fuel.core.deserializers.StringDeserializer
import com.github.kittinunf.fuel.core.requests.CancellableRequest
import com.github.kittinunf.fuel.core.response
import com.github.kittinunf.result.Result
import reactor.core.publisher.Mono
import reactor.core.publisher.MonoSink
import java.nio.charset.Charset
private fun Request.monoResult(async: Request.(MonoSink) -> CancellableRequest): Mono =
Mono.create { sink ->
val cancellableRequest = async(sink)
sink.onCancel { cancellableRequest.cancel() }
}
private fun Request.monoResultFold(mapper: Deserializable): Mono =
monoResult { sink ->
response(mapper) { _, _, result ->
result.fold(sink::success, sink::error)
}
}
private fun Request.monoResultUnFolded(mapper: Deserializable): Mono> =
monoResult { sink ->
response(mapper) { _, _, result ->
sink.success(result)
}
}
/**
* Get a single [Response]
* @return [Mono] the [Mono]
*/
fun Request.monoResponse(): Mono =
monoResult { sink ->
response { _, res, _ -> sink.success(res) }
}
/**
* Get a single [ByteArray] via a [MonoSink.success], or any [FuelError] via [MonoSink.error]
*
* @see monoResultBytes
* @return [Mono] the [Mono]
*/
fun Request.monoBytes(): Mono = monoResultFold(ByteArrayDeserializer())
/**
* Get a single [String] via a [MonoSink.success], or any [FuelError] via [MonoSink.error]
*
* @see monoResultString
*
* @param charset [Charset] the charset to use for the string, defaults to [Charsets.UTF_8]
* @return [Mono] the [Mono]
*/
fun Request.monoString(charset: Charset = Charsets.UTF_8): Mono = monoResultFold(StringDeserializer(charset))
/**
* Get a single [T] via a [MonoSink.success], or any [FuelError] via [MonoSink.error]
*
* @see monoResultObject
*
* @param mapper [Deserializable] the deserializable that can turn the response int a [T]
* @return [Mono] the [Mono]
*/
fun Request.monoObject(mapper: Deserializable): Mono = monoResultFold(mapper)
/**
* Get a single [ByteArray] or [FuelError] via [Result]
*
* @see monoBytes
* @return [Mono>] the [Mono]
*/
fun Request.monoResultBytes(): Mono> =
monoResultUnFolded(ByteArrayDeserializer())
/**
* Get a single [String] or [FuelError] via [Result]
*
* @see monoString
*
* @param charset [Charset] the charset to use for the string, defaults to [Charsets.UTF_8]
* @return [Mono>] the [Mono]
*/
fun Request.monoResultString(charset: Charset = Charsets.UTF_8): Mono> =
monoResultUnFolded(StringDeserializer(charset))
/**
* Get a single [T] or [FuelError] via [Result]
*
* @see monoObject
* @return [Mono>] the [Mono]
*/
fun Request.monoResultObject(mapper: Deserializable): Mono> =
monoResultUnFolded(mapper)
/**
* Get a complete signal(success with [Unit]) via a [MonoSink.success], or any [FuelError] via [MonoSink.error]
*/
fun Request.monoUnit(): Mono = monoResultFold(EmptyDeserializer)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy