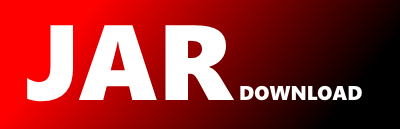
com.pega.uiframework.utils.WaitForDocStateReady Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pega-ui-testframework Show documentation
Show all versions of pega-ui-testframework Show documentation
Selenium/WebDriver Automation Framework
The newest version!
package com.pega.uiframework.utils;
import com.google.common.base.Function;
import org.apache.commons.lang3.time.StopWatch;
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.Wait;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.testng.Reporter;
import java.util.List;
public class WaitForDocStateReady {
private WebDriver driver = null;
private int QUIET_START = 1000;
private StopWatch gStopWatch = new StopWatch();
int TIMEOUT_WCOMM = 1000; // Wait for 1 second for communication to start...
// save a copy of the current document state counter.
private static int currentDocCounter = -1;
private static int currentAjaxCounter = -1;
// save a copy of the current ajax and document states.
private String currentAjaxStatus = null;
private String currentDocStatus = null;
public static int GLOBAL_TIMEOUT = 400;
public static final String DOC_STATE_XPATH= "//div[@class='document-statetracker']";
public WaitForDocStateReady(WebDriver driver) {
this.driver = driver;
}
/**
* this method is to wait till doc state is ready
* @return
*/
public boolean waitForDocStateReady() {
try {
return waitForDocStateReady(false);
} finally {
if(gStopWatch.isStarted())
gStopWatch.stop();
}
}
/**
* this method is to wait till the doc state is ready
* @param commStartTimeInMillis is maximum time to be waited for doc state is ready
* @return
*/
public boolean waitForDocStateReady(int commStartTimeInMillis) {
try {
TIMEOUT_WCOMM = commStartTimeInMillis * 1000;
return waitForDocStateReady(false);
} finally {
if(gStopWatch.isStarted())
gStopWatch.stop();
}
}
/**
* @deprecated use waitForDocStateReady(int) or waitForDocStateReady() instead.
* @param debug
* @return
*/
private boolean waitForDocStateReady(boolean debug) {
boolean everActive = false;
//long QUIET_BUMP = 500;
//int QUIET_MAX = 5000;
//long QUIET_INTERVAL = 5000;
int TIMEOUT_START = 30000;
//int TIMEOUT_WCOMM = 1000; // Wait for 1 second for communication to start...
int TIMEOUT_MAX = GLOBAL_TIMEOUT*1000;
long waitTimeout = TIMEOUT_START;
//long quietPeriod = QUIET_START;
StopWatch stopWatch = new StopWatch();
stopWatch.start();
Reporter.log ("Entering DocStateReady...",true);
if (!gStopWatch.isStarted())
gStopWatch.reset();
boolean everExisted = false;
List elements;
while (stopWatch.getTime() < waitTimeout) {
//Reporter.log(stopWatch.getTime()+":"+waitTimeout,true);
try {
waitForPageLoaded();
elements = driver.findElements(By.xpath(DOC_STATE_XPATH));
if (elements.size() > 0) {
everExisted = true;
String busyStatus = elements.get(0).getAttribute(
"data-state-busy-status");
//Reporter.log("Doc Status:"+docStatus+"\nBusy Status:"+busyStatus,true);
if (busyStatus.equals("none")) {
if(everActive)
{
System.out.println("");
}
break;
} else {
if(everActive == false)
{
everActive = true;
Reporter.log ("Document currently active, waiting entire document to load",true);
}
else
{
System.out.print(".");
}
}
if (everActive)
waitTimeout = TIMEOUT_MAX;
else
waitTimeout = TIMEOUT_WCOMM;
} else {
if (debug)
Reporter.log ("DEBUG: Doc State element not found. (Don't panic!)",true);
sleep();
}
} catch (Exception e) {
Reporter.log("Unexpected exception, will keep trying..." + e.toString(),true);
sleep();
}
}
if (!everExisted)
{
Reporter.log("!!! Document state div was never identified...are you sure your system is doc state aware?",true);
}
/* else if (!everActive)
{
Reporter.log("!!! Never detected communication - you *might* consider removing this call to 'waitForDocStateReady'.",true);
}*/
else if (stopWatch.getTime() >= waitTimeout)
{
Reporter.log("!!! Still looks like there was active calls, EVEN AFTER THE TIMEOUT (" + waitTimeout +")!",true);
}
// Final wait until ready for good luck!
//Common.safeToContinue();
waitForPageLoaded();
//Reporter.log("End of Doc state ready", true);
return everActive;
}
/**
* this provides the values of data-state-doc-counter & data-state-ajax-counter at current instance of time
* @param element
*/
private void syncDocStateCounters(WebElement element) {
currentDocCounter = Integer.parseInt(element
.getAttribute("data-state-doc-counter"));
currentAjaxCounter = Integer.parseInt(element
.getAttribute("data-state-ajax-counter"));
currentDocStatus = element.getAttribute("data-state-doc-status");
currentAjaxStatus = element.getAttribute("data-state-ajax-status");
}
/**
* this method sleeps fof 100 ms
*/
private void sleep(){
try{
Thread.sleep(100);
}catch(Exception e){}
}
public void waitForPageLoaded() {
Wait wait = new WebDriverWait(driver, GLOBAL_TIMEOUT);
try {
wait.until(checkPageLoadStatus());
} catch (Throwable error) {
}
}
private static Function checkPageLoadStatus() {
return new Function() {
public Boolean apply(WebDriver driver) {
return ((JavascriptExecutor) driver).executeScript("return document.readyState").equals("complete");
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy