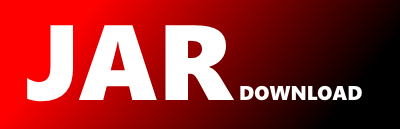
com.github.kmizu.kollection.KLazy.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kollection Show documentation
Show all versions of kollection Show documentation
A parser combinator library written in Kotlin
package com.github.kmizu.kollection
import com.github.kmizu.kollection.KOption.*
class KLazy(private val thunk: () -> T) {
private var value: KOption = None
fun force(): T = synchronized(this){
when(value) {
is Some -> value.get()
is None -> {
value = Some(thunk())
value.get()
}
}
}
val isForced: Boolean
get() = value != None
override fun equals(other: Any?): Boolean = when(other){
is KLazy<*> -> value == other.value
else -> false
}
fun map(function: (T) -> U): KLazy = KLazy{ function(force()) }
fun flatMap(function: (T) -> KLazy): KLazy = KLazy{ function(force()).force() }
override fun hashCode(): Int = value.hashCode()
override fun toString(): String = when(value){
is Some -> "KLazy(${value.get()})"
is None -> "KLazy(?)"
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy