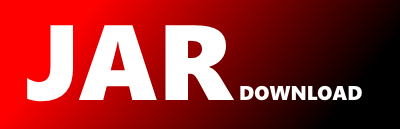
com.github.kmizu.kollection.KOption.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kollection Show documentation
Show all versions of kollection Show documentation
A parser combinator library written in Kotlin
package com.github.kmizu.kollection
sealed class KOption(): Iterable, KFoldable, KLinearSequence {
class Some(val value: T) : KOption() {
override fun equals(other: Any?): Boolean = when(other){
is Some<*> -> value == other.value
else -> false
}
override fun hashCode(): Int = value?.hashCode() ?: 0
}
object None : KOption() {
override fun equals(other: Any?): Boolean = super.equals(other)
}
/**
* Extract the value from this Option.
* Note that this method should not be used as soon as possible since it is dangerous.
*/
fun get(): T = when(this) {
is Some -> this.value
is None -> throw IllegalArgumentException("None")
}
override fun foldLeft(z: U, function: (U, T) -> U): U = when(this) {
is Some -> function(z, this.value)
is None -> z
}
override fun foldRight(z: U, function: (T, U) -> U): U = when(this) {
is Some -> function(this.value, z)
is None -> z
}
override val hd: T
get() = when(this) {
is Some -> this.value
is None -> throw IllegalArgumentException("None")
}
override val tl: KOption
get() = when(this) {
is Some -> None
is None -> throw IllegalArgumentException("None")
}
override val isEmpty: Boolean
get() = when(this) {
is Some -> false
is None -> true
}
fun map(function: (T) -> U): KOption = when(this) {
is Some -> Some(function(this.value))
is None -> None
}
fun flatMap(function: (T) -> KOption): KOption = when(this) {
is Some -> function(this.value)
is None -> None
}
fun filter(function: (T) -> Boolean): KOption = when(this) {
is Some -> if(function(this.value)) this else None
is None -> None
}
override fun toString(): String = when(this){
is Some<*> -> "Some(${value})"
is None -> "None"
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy