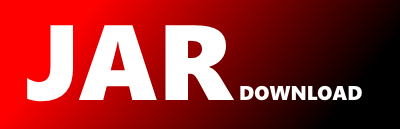
com.github.kondaurovdev.xml.XmlNode.scala Maven / Gradle / Ivy
package com.github.kondaurovdev.xml
import com.github.kondaurovdev.snippets.helper.TextHelper
import com.github.kondaurovdev.xml.XmlParser.Param
import scala.collection.mutable
import scala.xml.{Atom, Node}
object XmlNode {
val CONTENT = "content"
def apply(node: Node): XmlNode = new XmlNode(node)
}
class XmlNode(node: Node) {
def getAttrs(names: Iterable[String] = List()): Map[String, String] = {
if (names.isEmpty) {
node.attributes.flatMap(a => a.value.headOption.map(v => a.key -> v.text)).toMap
} else {
names.flatMap(n => {
getAttr(n).map(v => {
n -> v
})
}).toMap
}
}
def getPropNames: List[String] = {
node.child.flatMap { p =>
if (p.isAtom) None else Some(p.label)
}.toList
}
def getProps(names: Iterable[String] = List(), includeText: Boolean = false): Map[String, String] = {
val result = mutable.LinkedHashMap.empty[String, String]
names.foreach(name => {
getProp(name).foreach(v => result += name -> v)
})
node.child.foreach {
case a: Atom[_] =>
val text = TextHelper.trimBad(a.text)
if (text.nonEmpty) result += XmlNode.CONTENT -> text
case _ =>
}
result.toMap
}
def getAttr(attrName: String): Option[String] = {
node.attribute(attrName).flatMap(_.headOption.map(_.text))
}
def getProp(attrName: String): Option[String] = {
(node \ attrName).headOption.map(prop => {
prop.text
})
}
def getParams(param: Param): Map[String, String] = {
val res = mutable.LinkedHashMap.empty[String, String]
node.child.foreach {
case n if n.label.equalsIgnoreCase(param.tagName) =>
for (
name <- n.attribute(param.attrName);
nameFirst <- name.headOption.map(_.text)
) {
res += s"${param.prefix}.$nameFirst" -> n.text
}
case _ =>
}
res.toMap
}
def getFlatObj(fields: Iterable[String] = List(), params: Iterable[Param] = List(), includeText: Boolean = false): Map[String, String] = {
val result = mutable.LinkedHashMap.empty[String, String]
result ++= getAttrs(fields)
result ++= getProps(if (fields.isEmpty) getPropNames else fields, includeText)
params.foreach(p => {
result ++= getParams(p)
})
result.toMap
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy