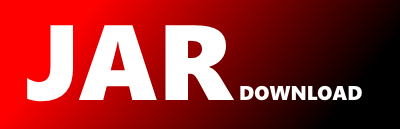
com.github.kongchen.swagger.docgen.mustache.MustacheDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swagger-maven-plugin Show documentation
Show all versions of swagger-maven-plugin Show documentation
A maven build plugin which helps you generate API document during build phase
package com.github.kongchen.swagger.docgen.mustache;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.github.kongchen.swagger.docgen.TypeUtils;
import com.wordnik.swagger.core.ApiValues;
import com.wordnik.swagger.core.Documentation;
import com.wordnik.swagger.core.DocumentationParameter;
import com.wordnik.swagger.core.DocumentationSchema;
/**
* Created with IntelliJ IDEA.
*
* @author: chekong
*/
public class MustacheDocument implements Comparable{
protected static final String VOID = "void";
protected static final String ARRAY = "Array";
protected static final String ANY = "any";
@JsonIgnore
private final HashMap models;
private int index;
String resourcePath;
String description;
List apis = new LinkedList();
@JsonIgnore
private Set requestTypes = new LinkedHashSet();
@JsonIgnore
private Set responseTypes = new LinkedHashSet();
@JsonIgnore
private int apiIndex = 1;
public MustacheDocument(Documentation swaggerDoc) {
this.models = swaggerDoc.getModels();
this.resourcePath = swaggerDoc.getResourcePath();
}
public void setResourcePath(String resourcePath) {
this.resourcePath = resourcePath;
}
public void setDescription(String description) {
this.description = description;
}
public String getResourcePath() {
return resourcePath;
}
public String getDescription() {
return description;
}
public List getApis() {
return apis;
}
public Set getRequestTypes() {
return requestTypes;
}
public Set getResponseTypes() {
return responseTypes;
}
public int getIndex() {
return index;
}
public void setIndex(int index) {
this.index = index;
}
public void addApi(MustacheApi wapi) {
wapi.apiIndex = apiIndex++;
apis.add(wapi);
}
public void addResponseType(String trueType) {
if (trueType != null) {
responseTypes.add(trueType);
}
}
public List analyzeParameters(List parameters) {
if (parameters == null) return null;
List list = new LinkedList();
Map> paraMap = toParameterTypeMap(parameters);
for (Map.Entry> entry : paraMap.entrySet()) {
list.add(new MustacheParameterSet(entry));
}
return list;
}
private Map> toParameterTypeMap(List parameters) {
Map> paraMap = new HashMap>();
for (DocumentationParameter para : parameters) {
MustacheParameter mustacheParameter = analyzeParameter(para);
List paraList = paraMap.get(para.getParamType());
if (paraList == null) {
paraList = new LinkedList();
paraMap.put(para.getParamType(), paraList);
}
paraList.add(mustacheParameter);
}
return paraMap;
}
private MustacheParameter analyzeParameter(DocumentationParameter para) {
MustacheParameter mustacheParameter = new MustacheParameter(para);
if (models != null && models.get(mustacheParameter.linkType) == null) {
mustacheParameter.setName(para.getName());
} else {
if (mustacheParameter.getLinkType() != null
&& !para.getParamType().equals(ApiValues.TYPE_HEADER)){
requestTypes.add(mustacheParameter.getLinkType());
}
if (para.getName() != null) {
mustacheParameter.setName(para.getName());
} else {
mustacheParameter.setName(para.getDataType());
}
}
return mustacheParameter;
}
public List analyzeDataTypes(String responseClass) {
if (responseClass == null || responseClass.equals(VOID) || models == null) {
return null;
}
List mustacheItemList = new LinkedList();
DocumentationSchema field = models.get(TypeUtils.upperCaseFirstCharacter(responseClass));
if (field != null && field.getProperties() != null) {
for (Map.Entry entry : field.getProperties().entrySet()) {
MustacheItem mustacheItem = new MustacheItem(entry.getKey(), entry.getValue());
DocumentationSchema item = entry.getValue().getItems();
if (models.get(mustacheItem.getType()) != null) {
responseTypes.add(mustacheItem.getType());
} else if (mustacheItem.getType().equalsIgnoreCase(ARRAY)) {
handleArrayType(mustacheItem, item);
}
mustacheItemList.add(mustacheItem);
}
}
return mustacheItemList;
}
private void handleArrayType(MustacheItem mustacheItem, DocumentationSchema item) {
if (item != null) {
if (item.getType().equals(ANY) && item.ref() != null) {
mustacheItem.setTypeAsArray(item.ref());
responseTypes.add(item.ref());
} else {
mustacheItem.setTypeAsArray(item.getType());
}
}
}
@Override
public int compareTo(MustacheDocument o) {
if (o == null) {
return 1;
}
return this.getResourcePath().compareTo(o.getResourcePath());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy