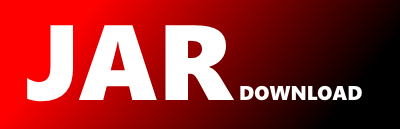
com.github.kongchen.swagger.docgen.reader.JaxrsReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swagger-maven-plugin
Show all versions of swagger-maven-plugin
A maven build plugin which helps you generate API document during build phase
package com.github.kongchen.swagger.docgen.reader;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.github.kongchen.swagger.docgen.LogAdapter;
import io.swagger.annotations.*;
import io.swagger.converter.ModelConverters;
import io.swagger.jaxrs.ext.SwaggerExtension;
import io.swagger.jaxrs.ext.SwaggerExtensions;
import io.swagger.models.*;
import io.swagger.models.Tag;
import io.swagger.models.parameters.Parameter;
import io.swagger.models.properties.ArrayProperty;
import io.swagger.models.properties.MapProperty;
import io.swagger.models.properties.Property;
import io.swagger.models.properties.RefProperty;
import io.swagger.util.Json;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.annotation.AnnotationUtils;
import javax.ws.rs.Consumes;
import javax.ws.rs.HttpMethod;
import javax.ws.rs.Produces;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.util.*;
public class JaxrsReader extends AbstractReader implements ClassSwaggerReader {
Logger LOGGER = LoggerFactory.getLogger(JaxrsReader.class);
static ObjectMapper m = Json.mapper();
public JaxrsReader(Swagger swagger, LogAdapter LOG) {
super(swagger, LOG);
}
@Override
public Swagger read(Set> classes) {
for (Class cls : classes)
read(cls);
return swagger;
}
public Swagger getSwagger() {
return this.swagger;
}
public Swagger read(Class cls) {
return read(cls, "", null, false, new String[0], new String[0], new HashMap(), new ArrayList());
}
protected Swagger read(Class> cls, String parentPath, String parentMethod, boolean readHidden, String[] parentConsumes, String[] parentProduces, Map parentTags, List parentParameters) {
if (swagger == null)
swagger = new Swagger();
Api api = AnnotationUtils.findAnnotation(cls, Api.class);
Map globalScopes = new HashMap();
javax.ws.rs.Path apiPath = AnnotationUtils.findAnnotation(cls, javax.ws.rs.Path.class);
// only read if allowing hidden apis OR api is not marked as hidden
if (!canReadApi(readHidden, api)) {
return swagger;
}
Map tags = updateTagsForApi(parentTags, api);
List securities = getSecurityRequirements(api);
// merge consumes, produces
// look for method-level annotated properties
// handle subresources by looking at return type
// parse the method
Method methods[] = cls.getMethods();
for (Method method : methods) {
ApiOperation apiOperation = AnnotationUtils.findAnnotation(method, ApiOperation.class);
if (apiOperation == null || apiOperation.hidden()) {
continue;
}
javax.ws.rs.Path methodPath = AnnotationUtils.findAnnotation(method, javax.ws.rs.Path.class);
String operationPath = getPath(apiPath, methodPath, parentPath);
if (operationPath != null && apiOperation != null) {
Map regexMap = new HashMap();
operationPath = parseOperationPath(operationPath, regexMap);
String httpMethod = extractOperationMethod(apiOperation, method, SwaggerExtensions.chain());
Operation operation = parseMethod(method);
updateOperationParameters(parentParameters, regexMap, operation);
updateOperationProtocols(apiOperation, operation);
String[] apiConsumes = new String[0];
String[] apiProduces = new String[0];
Annotation annotation = AnnotationUtils.getAnnotation(cls, Consumes.class);
if (annotation != null)
apiConsumes = ((Consumes) annotation).value();
annotation = AnnotationUtils.getAnnotation(cls, Produces.class);
if (annotation != null)
apiProduces = ((Produces) annotation).value();
apiConsumes = updateOperationConsumes(parentConsumes, apiConsumes, operation);
apiProduces = updateOperationProduces(parentProduces, apiProduces, operation);
handleSubResource(apiConsumes, httpMethod, apiProduces, tags, method, operationPath, operation);
// can't continue without a valid http method
httpMethod = httpMethod == null ? parentMethod : httpMethod;
updateTagsForOperation(operation, apiOperation);
updateOperation(apiConsumes, apiProduces, tags, securities, operation);
updatePath(operationPath, httpMethod, operation);
}
}
return swagger;
}
private void handleSubResource(String[] apiConsumes, String httpMethod, String[] apiProduces, Map tags, Method method, String operationPath, Operation operation) {
if (isSubResource(method)) {
Type t = method.getGenericReturnType();
Class> responseClass = method.getReturnType();
Swagger subSwagger = read(responseClass, operationPath, httpMethod, true, apiConsumes, apiProduces, tags, operation.getParameters());
}
}
protected boolean isSubResource(Method method) {
Type t = method.getGenericReturnType();
Class> responseClass = method.getReturnType();
if (responseClass != null && AnnotationUtils.findAnnotation(responseClass, Api.class) != null) {
return true;
}
return false;
}
String getPath(javax.ws.rs.Path classLevelPath, javax.ws.rs.Path methodLevelPath, String parentPath) {
if (classLevelPath == null && methodLevelPath == null)
return null;
StringBuilder b = new StringBuilder();
if (parentPath != null && !"".equals(parentPath) && !"/".equals(parentPath)) {
if (!parentPath.startsWith("/"))
parentPath = "/" + parentPath;
if (parentPath.endsWith("/"))
parentPath = parentPath.substring(0, parentPath.length() - 1);
b.append(parentPath);
}
if (classLevelPath != null) {
b.append(classLevelPath.value());
}
if (methodLevelPath != null && !"/".equals(methodLevelPath.value())) {
String methodPath = methodLevelPath.value();
if (!methodPath.startsWith("/") && !b.toString().endsWith("/")) {
b.append("/");
}
if (methodPath.endsWith("/")) {
methodPath = methodPath.substring(0, methodPath.length() - 1);
}
b.append(methodPath);
}
String output = b.toString();
if (!output.startsWith("/"))
output = "/" + output;
if (output.endsWith("/") && output.length() > 1)
return output.substring(0, output.length() - 1);
else
return output;
}
public Operation parseMethod(Method method) {
Operation operation = new Operation();
ApiOperation apiOperation = (ApiOperation) AnnotationUtils.findAnnotation(method, ApiOperation.class);
String operationId = method.getName();
String responseContainer = null;
Class> responseClass = null;
Map defaultResponseHeaders = null;
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy