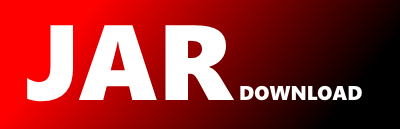
com.github.kristofa.test.http.UnsatisfiedExpectationException Maven / Gradle / Ivy
Show all versions of mock-http-server Show documentation
package com.github.kristofa.test.http;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import org.apache.commons.lang3.Validate;
/**
* Indicates there are {@link HttpRequest http requests} that we expected but did not get and/or that we got unexpected
* {@link HttpRequest http requests}.
*
* @see HttpResponseProvider
* @author kristof
*/
public class UnsatisfiedExpectationException extends Exception {
private static final long serialVersionUID = -6003072239642243697L;
private final List missingHttpRequests = new ArrayList();
private final List unexpectedHttpRequests = new ArrayList();
/**
* Creates a new instance.
*
* Both collections should not be null
. One of both collections can be empty.
*
* @param missingRequests Requests that we expected but did not get.
* @param unexpectedRequests Requests that we got but did not expect.
*/
public UnsatisfiedExpectationException(final Collection missingRequests,
final Collection unexpectedRequests) {
super();
Validate.notNull(missingRequests);
Validate.notNull(unexpectedRequests);
Validate.isTrue(!missingRequests.isEmpty() || !unexpectedRequests.isEmpty());
missingHttpRequests.addAll(missingRequests);
unexpectedHttpRequests.addAll(unexpectedRequests);
}
/**
* Gets the http requests that we expected but did not get.
*
* @return Collection of http requests that we expected but did not get.
*/
public Collection getMissingHttpRequests() {
return Collections.unmodifiableCollection(missingHttpRequests);
}
/**
* Gets the http requests that we got but did not expect.
*
* @return Collection of http requests that we got but did not expect.
*/
public Collection getUnexpectedHttpRequests() {
return Collections.unmodifiableCollection(unexpectedHttpRequests);
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
final String missingExpectedRequestsString = "Missing expected requests: " + getMissingHttpRequests();
final String unexpectedReceivedRequestsString = "Unexpected received requests: " + getUnexpectedHttpRequests();
return missingExpectedRequestsString + "\n" + unexpectedReceivedRequestsString;
}
}