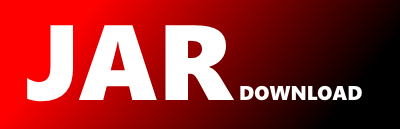
com.github.kristofa.test.http.client.ApacheHttpClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mock-http-server Show documentation
Show all versions of mock-http-server Show documentation
Mock and Proxy HTTP Server for testing purposes. Forked from https://github.com/jharlap/mock-http-server
package com.github.kristofa.test.http.client;
import java.io.IOException;
import java.io.InputStream;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpDelete;
import org.apache.http.client.methods.HttpEntityEnclosingRequestBase;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.client.methods.HttpRequestBase;
import org.apache.http.entity.ByteArrayEntity;
import org.apache.http.impl.client.DefaultHttpClient;
import com.github.kristofa.test.http.FullHttpRequest;
import com.github.kristofa.test.http.HttpMessageHeader;
import com.github.kristofa.test.http.Method;
/**
* {@link HttpClient} implementation that uses Apache HTTP
* client as 'lower level' library.
*
* @author kristof
*/
public class ApacheHttpClientImpl implements HttpClient {
public ApacheHttpClientImpl() {
}
/**
* {@inheritDoc}
*/
@Override
public HttpClientResponse execute(final FullHttpRequest request) throws HttpRequestException {
if (request.getMethod().equals(Method.GET)) {
final HttpGet httpGet = new HttpGet(request.getUrl());
populateHeader(request, httpGet);
try {
return execute(httpGet);
} catch (final IOException e1) {
throw new GetException(e1);
}
} else if (request.getMethod().equals(Method.PUT)) {
final HttpPut httpPut = new HttpPut(request.getUrl());
populateHeader(request, httpPut);
try {
return execute(httpPut, request.getContent());
} catch (final IOException e1) {
throw new PutException(e1);
}
} else if (request.getMethod().equals(Method.POST)) {
final HttpPost httpPost = new HttpPost(request.getUrl());
populateHeader(request, httpPost);
try {
return execute(httpPost, request.getContent());
} catch (final IOException e1) {
throw new PostException(e1);
}
} else if (request.getMethod().equals(Method.DELETE)) {
final HttpDelete httpDelete = new HttpDelete(request.getUrl());
populateHeader(request, httpDelete);
try {
return execute(httpDelete);
} catch (final IOException e1) {
throw new GetException(e1);
}
}
throw new HttpRequestException("Unsupported operation: " + request.getMethod());
}
/**
* Gets a new HTTPClient instance. Introduced to facilitate testing.
*
* @return A new HTTPClient instance.
*/
/* package */org.apache.http.client.HttpClient getClient() {
return new DefaultHttpClient();
}
private void populateHeader(final FullHttpRequest request, final HttpRequestBase apacheRequest) {
for (final HttpMessageHeader header : request.getHttpMessageHeaders()) {
apacheRequest.addHeader(header.getName(), header.getValue());
}
}
private HttpClientResponse execute(final HttpEntityEnclosingRequestBase request, final byte[] content)
throws IOException {
if (content != null) {
request.setEntity(new ByteArrayEntity(content));
}
return execute(request);
}
private HttpClientResponse execute(final HttpRequestBase request) throws IOException {
final org.apache.http.client.HttpClient client = getClient();
try {
final HttpResponse httpResponse = client.execute(request);
return buildResponse(client, httpResponse);
} catch (final IOException e) {
client.getConnectionManager().shutdown(); // In case of exception we should close connection manager here.
throw e;
}
}
private HttpClientResponse buildResponse(final org.apache.http.client.HttpClient client,
final HttpResponse response) throws IOException {
final int status = response.getStatusLine().getStatusCode();
final ApacheHttpClientResponseImpl httpResponse =
new ApacheHttpClientResponseImpl(status, client);
httpResponse.setResponseEntity(response.getEntity().getContent());
if (response.getEntity().getContentType() != null) {
httpResponse.setContentType(response.getEntity().getContentType().getValue());
}
if (status < 200 || status > 299) {
// In this case response will be the error message.
httpResponse.setErrorMessage("Got HTTP return code " + status);
}
return httpResponse;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy